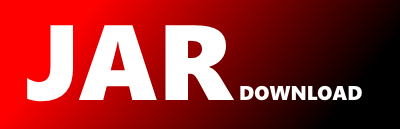
cdc.issues.IssueId Maven / Gradle / Ivy
package cdc.issues;
import java.util.Arrays;
import java.util.Comparator;
import java.util.List;
import java.util.Objects;
import cdc.issues.locations.Location;
import cdc.issues.rules.RuleId;
import cdc.util.lang.Checks;
/**
* Data issue identifier.
*
* It is composed of:
*
* - a rule identifier (domain and rule name)
*
- rule parameters
*
- a project name
*
- locations
*
* Note: several occurrences of issues may have the same identifier.
* They will differ by their snapshot.
*
* @author Damien Carbonne
*/
public final class IssueId implements Comparable {
private static final String DOMAIN = "domain";
private static final String LOCATION = "location";
private static final String LOCATIONS = "locations";
private static final String NAME = "name";
private static final String PARAMS = "params";
private static final String RULE_ID = "ruleId";
/** Identifier of the associated rule. */
private final RuleId ruleId;
/** Parameters used to configure the associated rule. */
private final Params params;
/** Name of the project. */
private final String project;
/** Target locations. */
private final Location[] locations;
private IssueId(Builder builder) {
this.ruleId = new RuleId(builder.domain, builder.name);
this.params = Checks.isNotNull(builder.params, PARAMS);
this.project = builder.project;
this.locations = Checks.isNotNull(builder.locations, LOCATIONS).clone();
}
/**
* @return The rule identifier.
*/
public RuleId getRuleId() {
return ruleId;
}
/**
* @return The rule domain name (part of its identifier).
*/
public String getDomain() {
return ruleId.getDomain();
}
/**
* @return The rule name (part of its identifier).
*/
public String getName() {
return ruleId.getName();
}
/**
* @param The enum type.
* @param cls The enum class.
* @return The rule name as an enum.
*/
public > T getName(Class cls) {
return ruleId.getName(cls);
}
/**
* @return The parameters used to configure the rule.
*/
public Params getParams() {
return params;
}
/**
* @return The project name (possibly {@code null}).
*/
public String getProject() {
return project;
}
/**
* @return The locations related to the issue.
*/
public Location[] getLocations() {
return locations.clone();
}
@Override
public int hashCode() {
return Objects.hash(ruleId,
params,
project)
+ 31 * Arrays.hashCode(locations);
}
@Override
public boolean equals(Object object) {
if (this == object) {
return true;
}
if (!(object instanceof IssueId)) {
return false;
}
final IssueId other = (IssueId) object;
return this.ruleId.equals(other.ruleId)
&& Objects.equals(params, other.params)
&& Objects.equals(project, other.project)
&& Arrays.equals(this.locations, other.locations);
}
private static final Comparator STRING_COMPARATOR =
Comparator.nullsFirst(Comparator.naturalOrder());
@Override
public int compareTo(IssueId other) {
final int ruleIdCmp = ruleId.compareTo(other.ruleId);
if (ruleIdCmp != 0) {
return ruleIdCmp;
}
final int paramsCmp = params.compareTo(other.params);
if (paramsCmp != 0) {
return paramsCmp;
}
final int projectCmp = STRING_COMPARATOR.compare(project, other.project);
if (projectCmp != 0) {
return projectCmp;
}
return Arrays.compare(locations, other.locations);
}
@Override
public String toString() {
return "[" + ruleId + " " + params + " " + project + " " + Arrays.toString(locations) + "]";
}
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private String domain;
private String name;
private Params params = Params.NO_PARAMS;
private String project;
private Location[] locations = {};
private Builder() {
}
public Builder ruleId(RuleId ruleId) {
Checks.isNotNull(ruleId, RULE_ID);
this.domain = ruleId.getDomain();
this.name = ruleId.getName();
return this;
}
public Builder domain(String domain) {
this.domain = Checks.isNotNull(domain, DOMAIN);
return this;
}
public Builder name(String name) {
this.name = Checks.isNotNull(name, NAME);
return this;
}
public Builder name(Enum> name) {
this.name = Checks.isNotNull(name, NAME).name();
return this;
}
public Builder params(Params params) {
this.params = Checks.isNotNull(params, PARAMS);
return this;
}
public Builder project(String project) {
// May be null
this.project = project;
return this;
}
public Builder location(Location location) {
this.locations = new Location[] { Checks.isNotNull(location, LOCATION) };
return this;
}
public Builder locations(Location... locations) {
this.locations = Checks.isNotNull(locations, LOCATIONS);
return this;
}
public Builder locations(List extends Location> locations) {
Checks.isNotNull(locations, LOCATIONS);
this.locations = locations.toArray(new Location[locations.size()]);
return this;
}
public IssueId build() {
return new IssueId(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy