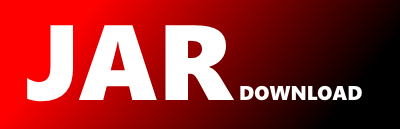
cdc.issues.Metas Maven / Gradle / Ivy
package cdc.issues;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.stream.Collectors;
import cdc.util.lang.Checks;
/**
* Set of {@link Meta}, with unique names.
*/
public interface Metas extends NameValueMap {
public static final Metas NO_METAS = new MetasImpl(Collections.emptyMap());
/**
* @return A collection of {@link Meta}.
*/
public Collection getMetas();
/**
* @return A list of {@link Meta} sorted using their name.
*/
public List getSortedMetas();
public static Builder builder() {
return new Builder();
}
@Deprecated(since = "2024-03-30", forRemoval = true)
static Metas of(Params params) {
final Builder builder = builder();
for (final String name : params.getNames()) {
builder.meta(name, params.getValue(name));
}
return builder.build();
}
public static final class Builder extends NameValueMap.Builder {
private Builder() {
}
/**
* Adds a new (name, value) pair.
*
* @param name The meta name.
* @param value The meta value.
* @return This builder.
* @throws IllegalArgumentException When {@code name} is not valid.
*/
public Builder meta(String name,
String value) {
return entry(name, value);
}
public Builder metas(Collection metas) {
return entries(metas);
}
public Builder metas(Metas metas) {
return entries(metas);
}
@Override
public Metas build() {
if (map.isEmpty()) {
return NO_METAS;
} else {
return new MetasImpl(map);
}
}
}
}
record MetasImpl(Map map) implements Metas {
MetasImpl {
for (final String name : map.keySet()) {
Checks.isTrue(Meta.isValidName(name), "name: '{}'", name);
}
}
@Override
public boolean isEmpty() {
return map.isEmpty();
}
@Override
public Set getNames() {
return map.keySet();
}
@Override
public List getSortedNames() {
return map.keySet()
.stream()
.sorted()
.toList();
}
@Override
public String getValue(String name) {
return map.get(name);
}
@Override
public String getValue(String name,
String def) {
return map.getOrDefault(name, def);
}
@Override
public Collection getMetas() {
return map.keySet()
.stream()
.map(name -> Meta.of(name, getValue(name)))
.toList();
}
@Override
public List getSortedMetas() {
return map.keySet()
.stream()
.sorted()
.map(name -> Meta.of(name, getValue(name)))
.toList();
}
@Override
public String toString() {
return getSortedMetas().stream()
.map(Meta::toString)
.collect(Collectors.joining(", ", "[", "]"));
}
@Override
public Collection extends NameValue> getNameValues() {
return getMetas();
}
@Override
public List extends NameValue> getSortedNameValues() {
return getSortedMetas();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy