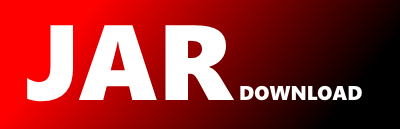
cdc.issues.NameValueMap Maven / Gradle / Ivy
package cdc.issues;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
public interface NameValueMap {
/**
* @return {@code true} if this map is empty.
*/
public boolean isEmpty();
/**
* @return The set of names.
*/
public Set getNames();
/**
* @return The sorted names.
*/
public List getSortedNames();
/**
* @param name The name.
* @return The value associated to {@code name} or {@code null}.
*/
public String getValue(String name);
/**
* @param name The name.
* @param def The default value to return.
* @return The value associated to {@code name} or {@code def}.
*/
public String getValue(String name,
String def);
/**
* @return A collection of {@link NameValue}.
*/
public Collection extends NameValue> getNameValues();
/**
* @return A list of {@link NameValue} sorted using their name.
*/
public List extends NameValue> getSortedNameValues();
public abstract static class Builder> {
protected Map map = new HashMap<>();
protected Builder() {
}
@SuppressWarnings("unchecked")
protected B self() {
return (B) this;
}
/**
* Adds a new (name, value) pair.
*
* @param name The meta name.
* @param value The meta value.
* @return This builder.
* @throws IllegalArgumentException When {@code name} is not valid.
*/
public B entry(String name,
String value) {
map.put(name, value);
return self();
}
public B entries(Collection extends NameValue> entries) {
for (final NameValue entry : entries) {
map.put(entry.getName(), entry.getValue());
}
return self();
}
public B entries(NameValueMap other) {
for (final String name : other.getNames()) {
map.put(name, other.getValue(name));
}
return self();
}
public abstract M build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy