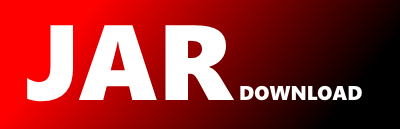
cdc.issues.io.IssuesReader Maven / Gradle / Ivy
package cdc.issues.io;
import java.io.File;
import java.io.IOException;
import java.io.OutputStream;
import java.util.EnumSet;
import java.util.Set;
import cdc.issues.answers.IssuesAndAnswers;
import cdc.util.events.ProgressController;
import cdc.util.lang.Checks;
/**
* Interface implemented by classes that can read Issues and Answers
* from a {@link File} or an {@link OutputStream}.
*
* @author Damien Carbonne
* @see IssuesFormat
*/
@FunctionalInterface
public interface IssuesReader {
/**
* Class used to configure loading of issues.
*/
public static class Settings {
/**
* Enumeration of possible hints.
*/
public enum Hint {
/** If set, answers are not loaded. */
NO_ANSWERS
}
private final Set hints;
protected Settings(Set hints) {
this.hints = Checks.isNotNull(hints, "hints");
}
/**
* @return The set of enabled hints.
*/
public Set getHints() {
return hints;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private final Set hints = EnumSet.noneOf(Hint.class);
protected Builder() {
}
public Builder hint(Hint hint) {
this.hints.add(hint);
return this;
}
public Settings build() {
return new Settings(hints);
}
}
}
/**
* Settings used to load answers with issues.
*/
public static final Settings ANSWERS =
Settings.builder()
.build();
/**
* Settings used to ignore answers.
*/
public static final Settings NO_ANSWERS =
Settings.builder()
.hint(Settings.Hint.NO_ANSWERS)
.build();
/**
* Loads issues and associated answers (if asked in settings).
*
* @param settings The settings.
* @param controller The progress controller.
* @return The loaded issues and answers.
* @throws IOException When an IO error occurs.
*/
public IssuesAndAnswers load(Settings settings,
ProgressController controller) throws IOException;
/**
* Loads issues and answers from a file.
*
* @param file The file.
* @param settings The settings.
* @param controller The progress controller.
* @param features The features used to configure the factory.
* @return The loaded issues and answers.
* @throws IOException When an IO error occurs.
*/
public static IssuesAndAnswers load(File file,
Settings settings,
ProgressController controller,
IssuesIoFactoryFeatures features) throws IOException {
final IssuesIoFactory factory = new IssuesIoFactory(features);
final IssuesReader reader = factory.createIssuesReader(file);
return reader.load(settings, controller);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy