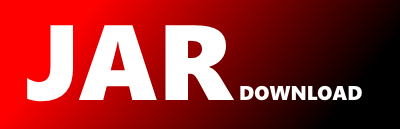
cdc.issues.io.OutSettings Maven / Gradle / Ivy
package cdc.issues.io;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Comparator;
import java.util.EnumSet;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import cdc.issues.Issue;
import cdc.issues.answers.IssueAnswer;
import cdc.issues.answers.IssuesAndAnswers;
import cdc.util.lang.Checks;
import cdc.util.lang.CollectionUtils;
import cdc.util.lang.IntHolder;
/**
* Class used to configure the writing of issues.
*/
public class OutSettings {
public static final OutSettings ALL_DATA_ANSWERS =
OutSettings.builder()
.build();
public static final OutSettings ALL_DATA_NO_ANSWERS =
OutSettings.builder()
.hint(OutSettings.Hint.NO_ANSWERS)
.build();
/**
* Enumeration of possible hints.
*/
public enum Hint {
/** If set, the Project column/field is not written. */
NO_PROJECT_COL,
/** If set, the Snapshot column/field is not written. */
NO_SNAPSHOT_COL,
/** If set, the Domain column/field is not written. */
NO_DOMAIN_COL,
/** If set, the Metas column/field is not written. */
NO_METAS_COL,
/** If set, Meta columns are automatically created. */
AUTO_METAS,
/** If set, the Labels column/field is not written. */
NO_LABELS_COL,
/** If set, the Params column/field is not written. */
NO_PARAMS_COL,
/** If set, Param columns are automatically created. */
AUTO_PARAMS,
/** If set, Answers are not written with issues. */
NO_ANSWERS,
/** If set, the Locations column/field is not written. */
NO_LOCATIONS_COL,
/** If set, Location columns are automatically created. */
AUTO_LOCATIONS,
/** If set, the Comments column/field is not written. */
NO_COMMENTS_COL,
/** If set, Comment columns are automatically created. */
AUTO_COMMENTS,
/** If set, the user (answer) metas column/field is not written. */
NO_USER_METAS_COL,
/** If set, User Meta columns are automatically created. */
AUTO_USER_METAS,
/** If set, the user (answer) labels column/field is not written. */
NO_USER_LABELS_COL;
/**
* @return {@code true} if this hint is one of the AUTO hints.
*/
public boolean isAuto() {
return this == AUTO_LOCATIONS
|| this == AUTO_METAS
|| this == AUTO_PARAMS
|| this == AUTO_COMMENTS
|| this == AUTO_USER_METAS;
}
}
private final Set hints;
private final List params;
private final String paramPrefix;
private final List metas;
private final String metaPrefix;
private final Comparator super String> metaComparator;
private final int numberOfLocations;
private final int numberOfComments;
private final List userMetas;
private final String userMetaPrefix;
private final Comparator super String> userMetaComparator;
protected OutSettings(Builder builder) {
this.hints = Checks.isNotNull(builder.hints, "hints");
this.params = Checks.isNotNull(builder.params, "params");
this.paramPrefix = builder.paramPrefix;
this.metas = Checks.isNotNull(builder.metas, "metas");
this.metaPrefix = builder.metaPrefix;
this.metaComparator = Checks.isNotNull(builder.metaComparator, "metaComparator");
this.numberOfLocations = builder.numberOfLocations;
this.numberOfComments = builder.numberOfComments;
this.userMetas = Checks.isNotNull(builder.userMetas, "userMetas");
this.userMetaPrefix = builder.userMetaPrefix;
this.userMetaComparator = Checks.isNotNull(builder.userMetaComparator, "userMetaComparator");
}
/**
* @return The set of enabled hints.
*/
public Set getHints() {
return hints;
}
/**
* @param hint The hint.
* @return {@code true} if {@code hint} is enabled.
*/
public boolean isEnabled(OutSettings.Hint hint) {
return hints.contains(hint);
}
/**
* @return The list of param data that have a special columns/fields.
*/
public List getParams() {
return params;
}
/**
* @return The prefix to use with param columns.
*/
public String getParamPrefix() {
return paramPrefix;
}
/**
* @return The list of meta data that have a special columns/fields.
*/
public List getMetas() {
return metas;
}
/**
* @return The prefix to use with meta columns.
*/
public String getMetaPrefix() {
return metaPrefix;
}
/**
* @return The Comparator that should be used to sort metas in meta column/field.
*/
public Comparator super String> getMetaComparator() {
return metaComparator;
}
/**
* @return The number of location columns to add.
*/
public int getNumberOfLocations() {
return numberOfLocations;
}
/**
* @return The number of comment columns to add.
*/
public int getNumberOfComments() {
return numberOfComments;
}
/**
* @return The list of meta data that have a special columns/fields.
*/
public List getUserMetas() {
return userMetas;
}
/**
* @return The prefix to use with meta columns.
*/
public String getUserMetaPrefix() {
return userMetaPrefix;
}
/**
* @return The Comparator that should be used to sort metas in meta column/field.
*/
public Comparator super String> getUserMetaComparator() {
return userMetaComparator;
}
public OutSettings replaceAuto(IssuesAndAnswers issuesAndAnswers) {
final OutSettings.Builder builder = builder();
boolean hasAuto = false;
for (final Hint hint : getHints()) {
if (hint.isAuto()) {
hasAuto = true;
} else {
builder.hint(hint);
}
}
if (hasAuto) {
final Set tmpParams = new HashSet<>();
final Set tmpMetas = new HashSet<>();
final IntHolder tmpNumberOfLocations = new IntHolder();
final IntHolder tmpNumberOfComments = new IntHolder();
final Set tmpUserMetas = new HashSet<>();
for (final Issue issue : issuesAndAnswers.getIssues()) {
tmpParams.addAll(issue.getParams().getNames());
tmpMetas.addAll(issue.getMetas().getNames());
tmpNumberOfLocations.value = Math.max(tmpNumberOfLocations.value, issue.getLocations().length);
final IssueAnswer answer = issuesAndAnswers.getAnswer(issue.getId()).orElse(null);
if (answer != null) {
tmpNumberOfComments.value = Math.max(tmpNumberOfComments.value, answer.getComments().size());
tmpUserMetas.addAll(answer.getMetas().getNames());
}
}
builder.metaPrefix(getMetaPrefix());
builder.paramPrefix(getParamPrefix());
builder.userMetaPrefix(getUserMetaPrefix());
if (isEnabled(Hint.AUTO_LOCATIONS)) {
builder.numberOfLocations(tmpNumberOfLocations.value);
} else {
builder.numberOfLocations(getNumberOfLocations());
}
if (isEnabled(Hint.AUTO_METAS)) {
builder.metas(CollectionUtils.toSortedList(tmpMetas));
} else {
builder.metas(getMetas());
}
if (isEnabled(Hint.AUTO_PARAMS)) {
builder.params(CollectionUtils.toSortedList(tmpParams));
} else {
builder.params(getParams());
}
if (isEnabled(Hint.AUTO_COMMENTS)) {
builder.numberOfComments(tmpNumberOfComments.value);
} else {
builder.numberOfComments(getNumberOfComments());
}
if (isEnabled(Hint.AUTO_USER_METAS)) {
builder.userMetas(CollectionUtils.toSortedList(tmpUserMetas));
} else {
builder.metas(getUserMetas());
}
return builder.build();
} else {
return this;
}
}
public static OutSettings.Builder builder() {
return new Builder();
}
/**
* Builder of {@link OutSettings}.
*
* @author Damien Carbonne
*/
public static class Builder {
private final Set hints = EnumSet.noneOf(OutSettings.Hint.class);
private final List params = new ArrayList<>();
private String paramPrefix = "Param.";
private final List metas = new ArrayList<>();
private String metaPrefix = "Meta.";
private Comparator super String> metaComparator = Comparator.naturalOrder();
private int numberOfLocations = -1;
private int numberOfComments = -1;
private final List userMetas = new ArrayList<>();
private String userMetaPrefix = "UserMeta.";
private Comparator super String> userMetaComparator = Comparator.naturalOrder();
protected Builder() {
}
public Builder hint(OutSettings.Hint hint) {
this.hints.add(hint);
return this;
}
public Builder param(String param) {
this.params.add(param);
return this;
}
public Builder params(Collection params) {
this.params.addAll(params);
return this;
}
public Builder paramPrefix(String paramPrefix) {
this.paramPrefix = paramPrefix;
return this;
}
public Builder meta(String meta) {
this.metas.add(meta);
return this;
}
public Builder metas(Collection metas) {
this.metas.addAll(metas);
return this;
}
public Builder metaPrefix(String metaPrefix) {
this.metaPrefix = metaPrefix;
return this;
}
public Builder metaComparator(Comparator super String> metaComparator) {
this.metaComparator = metaComparator;
return this;
}
public Builder numberOfLocations(int numberOfLocations) {
this.numberOfLocations = numberOfLocations;
return this;
}
public Builder numberOfComments(int numberOfComments) {
this.numberOfComments = numberOfComments;
return this;
}
public Builder userMeta(String userMeta) {
this.userMetas.add(userMeta);
return this;
}
public Builder userMetas(Collection userMetas) {
this.userMetas.addAll(userMetas);
return this;
}
public Builder userMetaPrefix(String userMetaPrefix) {
this.userMetaPrefix = userMetaPrefix;
return this;
}
public Builder userMetaComparator(Comparator super String> userMetaComparator) {
this.userMetaComparator = userMetaComparator;
return this;
}
public OutSettings build() {
return new OutSettings(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy