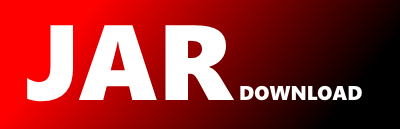
cdc.issues.rules.RuleConfig Maven / Gradle / Ivy
package cdc.issues.rules;
import java.util.Objects;
import java.util.Optional;
import cdc.issues.IssueSeverity;
import cdc.issues.Params;
import cdc.util.lang.Checks;
/**
* Configuration of a rule:
*
* - Its enabling
*
- The overridden severity.
*
- Its parameters
*
*
*/
public final class RuleConfig {
public static final RuleConfig DEFAULT = builder().build();
/** Is the rule enabled? */
private final boolean enabled;
/** The severity. May be null. */
private final Optional cvstomizedSeverity;
/** Parameters. */
private final Params params;
private RuleConfig(Builder builder) {
this.enabled = builder.enabled;
this.cvstomizedSeverity = Optional.ofNullable(builder.customizedSeverity);
this.params = Checks.isNotNull(builder.params, "params");
}
public boolean isEnabled() {
return enabled;
}
public Optional getCustomizedSeverity() {
return cvstomizedSeverity;
}
public Params getParams() {
return params;
}
@Override
public int hashCode() {
return Objects.hash(enabled,
cvstomizedSeverity,
params);
}
@Override
public boolean equals(Object object) {
if (this == object) {
return true;
}
if (!(object instanceof RuleConfig)) {
return false;
}
final RuleConfig other = (RuleConfig) object;
return enabled == other.enabled
&& Objects.equals(cvstomizedSeverity, other.cvstomizedSeverity)
&& Objects.equals(params, other.params);
}
@Override
public String toString() {
return "[" + isEnabled() + ", " + getCustomizedSeverity() + ", " + getParams() + "]";
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private boolean enabled = true;
private IssueSeverity customizedSeverity;
private Params params = Params.NO_PARAMS;
Builder() {
}
public Builder set(RuleConfig other) {
this.enabled = other.enabled;
this.customizedSeverity = other.cvstomizedSeverity.orElse(null);
this.params = other.params;
return this;
}
public Builder enabled(boolean enabled) {
this.enabled = enabled;
return this;
}
public Builder customizedSeverity(IssueSeverity customizedSeverity) {
this.customizedSeverity = customizedSeverity;
return this;
}
public Builder params(Params params) {
this.params = params;
return this;
}
public RuleConfig build() {
return new RuleConfig(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy