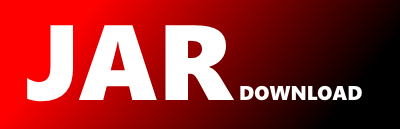
cdc.issues.checks.CheckContext Maven / Gradle / Ivy
package cdc.issues.checks;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import cdc.issues.locations.LocatedObject;
import cdc.util.lang.Checks;
/**
* Calling context passed to checkers.
*/
public final class CheckContext {
/** The empty check context. */
public static final CheckContext EMPTY = new CheckContext();
/** The stack of checked located objects. */
private final List> stack;
private CheckContext() {
this.stack = Collections.emptyList();
}
private CheckContext(List> elements) {
this.stack = elements;
}
/**
* @return The stack of checked located objects.
*/
public List> getStack() {
return stack;
}
/**
* @return The stack size.
*/
public final int size() {
return getStack().size();
}
/**
* Returns the located element at an index.
*
* @param The object type.
* @param objectClass The object class.
* @param index The index of the searched element.
* @return The element at index.
*/
public final LocatedObject extends O> get(Class objectClass,
int index) {
return getStack().get(index).cast(objectClass);
}
/**
* Creates a new context by appending an element to stack.
*
* @param The object type.
* @param element The element to push.
* @return A new CheckContext with element pushed at end.
*/
public CheckContext push(LocatedObject extends O> element) {
Checks.isNotNull(element, "element");
final List> list = new ArrayList<>(this.stack);
list.add(element);
return new CheckContext(list);
}
@Override
public String toString() {
return getStack().toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy