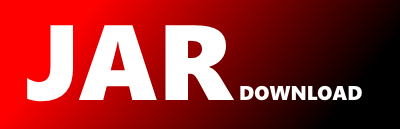
cdc.issues.ui.swing.IssuesPanel Maven / Gradle / Ivy
package cdc.issues.ui.swing;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.GridBagConstraints;
import java.awt.GridBagLayout;
import javax.swing.JButton;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.event.TableModelEvent;
import cdc.issues.IssueSeverity;
import cdc.issues.locations.Location;
import cdc.ui.swing.GridBagConstraintsBuilder;
import cdc.ui.swing.SwingUtils;
import cdc.ui.swing.cells.EnumUi;
public class IssuesPanel extends JPanel {
private static final long serialVersionUID = 1L;
private final JTable wTable;
private final IssuesTableModel model = new IssuesTableModel();
private final JButton wClear = new JButton("Clear");
private final JLabel wCount = new JLabel();
public IssuesPanel() {
setLayout(new GridBagLayout());
final JScrollPane wScrollPane = new JScrollPane();
wScrollPane.setPreferredSize(new Dimension(200, 100));
add(wScrollPane,
GridBagConstraintsBuilder.builder()
.gridx(0)
.gridy(0)
.gridwidth(2)
.weightx(1.0)
.weighty(1.0)
.fill(GridBagConstraints.BOTH)
.build());
wTable = new JTable(model);
wTable.setDefaultRenderer(Location[].class, new LocationsCellRenderer());
wScrollPane.setViewportView(wTable);
wTable.setAutoCreateRowSorter(true);
wTable.setAutoResizeMode(JTable.AUTO_RESIZE_OFF);
wTable.setDefaultRenderer(IssueSeverity.class,
new EnumUi.LabelCellRenderer<>(IssueSeverity.class, IssueSeverityUi.SETTINGS));
model.addTableModelListener(this::updateCounts);
wTable.getRowSorter().addRowSorterListener(e -> updateCounts());
SwingUtils.setColumnWidths(wTable,
200, // timestamp
100, // domain
200, // name
200, // params
75, // project
75, // snapshot
75, // severity
600, // description
600, // locations
200); // metas
add(wCount,
GridBagConstraintsBuilder.builder()
.gridx(0)
.gridy(1)
.weightx(1.0)
.anchor(GridBagConstraints.LINE_START)
.insets(5, 0, 0, 0)
.build());
add(wClear,
GridBagConstraintsBuilder.builder()
.gridx(1)
.gridy(1)
.weightx(1.0)
.anchor(GridBagConstraints.LINE_END)
.insets(5, 0, 0, 0)
.build());
wClear.addActionListener(e -> model.clear());
updateCounts();
}
public IssuesTableModel getModel() {
return model;
}
private void updateCounts(TableModelEvent event) {
if (event.getType() == TableModelEvent.DELETE
|| event.getType() == TableModelEvent.INSERT) {
updateCounts();
}
}
private void updateCounts() {
final int visible = wTable.getRowCount();
final int total = model.getRowCount();
wCount.setText(String.format("%,d issues", visible));
if (visible == total) {
wCount.setForeground(Color.BLACK);
} else {
wCount.setForeground(Color.BLUE);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy