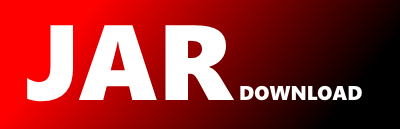
cdc.mf.ea.EaConnectionRole Maven / Gradle / Ivy
The newest version!
package cdc.mf.ea;
import cdc.mf.model.MfTipSide;
import cdc.util.lang.UnexpectedValueException;
/**
* Enumeration of roles that an object can play in a connection.
*
* @author Damien Carbonne
*/
public enum EaConnectionRole {
/** A class extends another class, or interface extends another interface. */
EXTENDS(MfTipSide.SOURCE, "specialization"),
/** A class is extended by another class, or interface is extended by another interface. */
EXTENDED_BY(MfTipSide.TARGET, "generalization"),
/** A class implements an interface. */
IMPLEMENTS(MfTipSide.SOURCE, "implementation"),
/** An interface is implemented by a class. */
IMPLEMENTED_BY(MfTipSide.TARGET, "implemented"),
/** A class or interface (whole) aggregates another class or interface (part). */
WHOLE(MfTipSide.SOURCE, "whole"),
/** A class or interface (part) is aggregated by another class or interface (whole). */
PART(MfTipSide.TARGET, "part"),
ANNOTATES(MfTipSide.SOURCE, "annotation"),
ANNOTATED_BY(MfTipSide.TARGET, "annotated"),
/** A class or interface (source) is associated to another class or interface (target). */
ASSOCIATION_SRC(MfTipSide.SOURCE, "source"),
/** A class or interface (target) is associated to another class or interface (source). */
ASSOCIATION_TGT(MfTipSide.TARGET, "target"),
/** An element that depends on another element, a dependency source. */
DEPENDER(MfTipSide.SOURCE, "depender"),
/** An element that is depended on by another element, a dependency target. */
DEPENDEE(MfTipSide.TARGET, "dependee");
private final MfTipSide side;
private final String literal;
private EaConnectionRole(MfTipSide side,
String literal) {
this.side = side;
this.literal = literal;
}
public MfTipSide getSide() {
return side;
}
public EaConnectorType getConnectorType() {
switch (this) {
case PART, WHOLE:
return EaConnectorType.AGGREGATION;
case ANNOTATED_BY, ANNOTATES:
return EaConnectorType.ANNOTATION;
case ASSOCIATION_SRC, ASSOCIATION_TGT:
return EaConnectorType.ASSOCIATION;
case EXTENDED_BY, EXTENDS:
return EaConnectorType.GENERALIZATION;
case IMPLEMENTED_BY, IMPLEMENTS:
return EaConnectorType.IMPLEMENTATION;
case DEPENDER, DEPENDEE:
return EaConnectorType.DEPENDENCY;
default:
throw new UnexpectedValueException(this);
}
}
public String getLiteral() {
return literal;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy