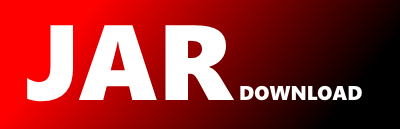
cdc.mf.ea.EaProfileExporter Maven / Gradle / Ivy
The newest version!
package cdc.mf.ea;
import java.io.File;
import java.io.IOException;
import java.sql.SQLException;
import java.util.function.UnaryOperator;
import java.util.regex.Pattern;
import org.apache.commons.cli.CommandLine;
import org.apache.commons.cli.Option;
import org.apache.commons.cli.Options;
import org.apache.commons.cli.ParseException;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import cdc.issues.core.io.MdProfileIo;
import cdc.issues.io.ProfileFormat;
import cdc.issues.io.ProfileIo;
import cdc.issues.io.ProfileIoFeatures;
import cdc.mf.Config;
import cdc.mf.ea.checks.EaProfile;
import cdc.util.cli.AbstractMainSupport;
import cdc.util.cli.FeatureMask;
import cdc.util.cli.MainResult;
import cdc.util.cli.OptionEnum;
import cdc.util.time.Chronometer;
public final class EaProfileExporter {
private static final Logger LOGGER = LogManager.getLogger(EaProfileExporter.class);
/**
* Pattern of sections that must be surrounded by ``.
*/
private static final Pattern EM_PATTERN = Pattern.compile("(<<[a-zA-Z0-9_ ]*>>|\\[[<>a-zA-Z0-9_ ]*\\])");
private final MainArgs margs;
public static class MainArgs {
/** Name of the generated file. */
public File output;
/** Set of enabled features. */
public final FeatureMask features = new FeatureMask<>();
public enum Feature implements OptionEnum {
SHOW_EMPTY_SECTIONS("show-empty-sections", "If enabled, empty sections are exported."),
SHOW_RULES_SEVERITY("show-rules-severities", "If enabled, rules severities are exported."),
SHOW_RULES_ENABLING("show-rules-enabling", "If enabled, rules enabling is exported."),
SHOW_RULES_DOMAIN("show-rules-domain", "If enabled, rules domain is exported."),
VERBOSE("verbose", "Prints messages.");
private final String name;
private final String description;
private Feature(String name,
String description) {
this.name = name;
this.description = description;
}
@Override
public final String getName() {
return name;
}
@Override
public final String getDescription() {
return description;
}
}
}
private EaProfileExporter(MainArgs margs) {
this.margs = margs;
}
private void log(String message) {
if (margs.features.isEnabled(MainArgs.Feature.VERBOSE)) {
LOGGER.info(message);
}
}
private void execute() throws IOException {
final Chronometer chrono = new Chronometer();
log("Export EA profile to " + margs.output);
chrono.start();
// Save profile
final ProfileFormat format = ProfileFormat.from(margs.output);
final UnaryOperator converter;
if (format == ProfileFormat.MD) {
converter = s -> MdProfileIo.wrap(MdProfileIo.mdReplaceNL(s), EM_PATTERN, "`", "`");
} else {
converter = UnaryOperator.identity();
}
final ProfileIoFeatures.Builder features =
ProfileIoFeatures.builder()
.hint(ProfileIoFeatures.Hint.PRETTY_PRINT)
.itemConverter(converter);
if (margs.features.contains(MainArgs.Feature.SHOW_EMPTY_SECTIONS)) {
features.hint(ProfileIoFeatures.Hint.SHOW_EMPTY_SECTIONS);
}
if (margs.features.contains(MainArgs.Feature.SHOW_RULES_DOMAIN)) {
features.hint(ProfileIoFeatures.Hint.SHOW_RULE_DOMAIN);
}
if (margs.features.contains(MainArgs.Feature.SHOW_RULES_ENABLING)) {
features.hint(ProfileIoFeatures.Hint.SHOW_RULE_ENABLING);
}
if (margs.features.contains(MainArgs.Feature.SHOW_RULES_SEVERITY)) {
features.hint(ProfileIoFeatures.Hint.SHOW_RULE_SEVERITY);
}
margs.output.getParentFile().mkdirs();
ProfileIo.save(features.build(), EaProfile.PROFILE, margs.output);
chrono.suspend();
log("Exported EA Profile(" + chrono + ")");
}
public static void execute(MainArgs margs) throws IOException {
final EaProfileExporter instance = new EaProfileExporter(margs);
instance.execute();
}
public static MainResult exec(String... args) {
final MainSupport support = new MainSupport();
support.main(args);
return support.getResult();
}
public static void main(String... args) {
final int code = exec(args).getCode();
System.exit(code);
}
private static class MainSupport extends AbstractMainSupport {
public MainSupport() {
super(EaProfileExporter.class, LOGGER);
}
@Override
protected String getVersion() {
return Config.VERSION;
}
@Override
protected boolean addArgsFileOption(Options options) {
return true;
}
@Override
protected String getHelpHeader() {
return """
Utility that can export the EA Profile.
Supported formats are: md, html, xml, json, xlsx, xls, xlsm, csv and ods.
Some of them cannot be loaded.""";
}
@Override
protected String getHelpFooter() {
return null;
}
@Override
protected void addSpecificOptions(Options options) {
options.addOption(Option.builder()
.longOpt(OUTPUT)
.desc("Name of the export file. Its extension must be recognized and supported.")
.hasArg()
.required()
.build());
addNoArgOptions(options, MainArgs.Feature.class);
}
@Override
protected MainArgs analyze(CommandLine cl) throws ParseException {
final MainArgs margs = new MainArgs();
margs.output = getValueAsFile(cl, OUTPUT);
setMask(cl, MainArgs.Feature.class, margs.features::setEnabled);
final ProfileFormat format = ProfileFormat.from(margs.output);
if (format == null || !format.isProfileExportFormat()) {
throw new ParseException("Non recognized or supported format: " + margs.output.getName());
}
return margs;
}
@Override
protected Void execute(MainArgs margs) throws IOException, SQLException {
EaProfileExporter.execute(margs);
return null;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy