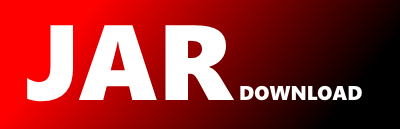
cdc.mf.html.PumlToAny Maven / Gradle / Ivy
The newest version!
package cdc.mf.html;
import java.io.File;
import java.io.IOException;
import java.util.List;
import org.apache.commons.cli.CommandLine;
import org.apache.commons.cli.Option;
import org.apache.commons.cli.Options;
import org.apache.commons.cli.ParseException;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import cdc.mf.Config;
import cdc.util.cli.AbstractMainSupport;
import cdc.util.cli.FeatureMask;
import cdc.util.cli.OptionEnum;
import cdc.util.files.Files;
import cdc.util.time.Chronometer;
import net.sourceforge.plantuml.FileFormat;
import net.sourceforge.plantuml.FileFormatOption;
import net.sourceforge.plantuml.GeneratedImage;
import net.sourceforge.plantuml.SourceFileReader;
/**
* Conversion of a PlantUML file to image.
*/
public final class PumlToAny {
private static final Logger LOGGER = LogManager.getLogger(PumlToAny.class);
private final MainArgs margs;
public static class MainArgs {
/** Input PlantUML file. */
public File input;
/** Output base directory. */
public File outputDir;
/** Set of enabled features. */
public final FeatureMask features = new FeatureMask<>();
public enum Feature implements OptionEnum {
FORCE("force",
"Force image generation when the target image exists and is newer than the PlantUML file."
+ "\nIf the target image does not exist or is older than the PlantUML file, it is always generated."),
NO_FORCE("no-force", "Do not force image generation (default)."),
PNG("png", "Generate a PNG image (default)."),
SVG("svg", "Generate an SVG image."),
VERBOSE("verbose", "Prints messages.");
private final String name;
private final String description;
private Feature(String name,
String description) {
this.name = name;
this.description = description;
}
@Override
public final String getName() {
return name;
}
@Override
public final String getDescription() {
return description;
}
}
}
private PumlToAny(MainArgs margs) {
this.margs = margs;
}
private void log(String message) {
if (margs.features.isEnabled(MainArgs.Feature.VERBOSE)) {
LOGGER.info(message);
}
}
private String getTargetExtension() {
if (margs.features.isEnabled(MainArgs.Feature.SVG)) {
return "svg";
} else {
return "png";
}
}
private void execute() throws IOException {
final Chronometer chrono = new Chronometer();
final File target = new File(margs.input.getParentFile(), Files.getNakedBasename(margs.input) + "." + getTargetExtension());
log("Convert " + margs.input);
chrono.start();
if (margs.features.contains(MainArgs.Feature.FORCE) || !target.exists() || Files.isNewerThan(margs.input, target)) {
final FileFormatOption ffo;
if (margs.features.isEnabled(MainArgs.Feature.SVG)) {
ffo = new FileFormatOption(FileFormat.SVG);
} else {
ffo = new FileFormatOption(FileFormat.PNG);
}
final SourceFileReader reader = new SourceFileReader(margs.input,
margs.input.getAbsoluteFile().getParentFile(),
ffo);
final List list = reader.getGeneratedImages();
for (final GeneratedImage img : list) {
log(" - " + img);
}
chrono.suspend();
log("Converted " + margs.input + " (" + chrono + ")");
} else {
chrono.suspend();
log("Skipped " + margs.input + " (" + chrono + ")");
}
}
public static void execute(MainArgs margs) throws IOException {
final PumlToAny instance = new PumlToAny(margs);
instance.execute();
}
public static void main(String... args) {
final MainSupport support = new MainSupport();
support.main(args);
}
private static class MainSupport extends AbstractMainSupport {
public MainSupport() {
super(PumlToAny.class, LOGGER);
}
@Override
protected String getVersion() {
return Config.VERSION;
}
@Override
protected boolean addArgsFileOption(Options options) {
return true;
}
@Override
protected String getHelpHeader() {
return "Converts a PlantUML file to an image.";
}
@Override
protected String getHelpFooter() {
return null;
}
@Override
protected void addSpecificOptions(Options options) {
options.addOption(Option.builder()
.longOpt(INPUT)
.desc("Mandatory name of the input PlantUML file.")
.hasArg()
.required()
.build());
options.addOption(Option.builder()
.longOpt(OUTPUT_DIR)
.desc("Mandatory name of the output directory.")
.hasArg()
.required()
.build());
addNoArgOptions(options, MainArgs.Feature.class);
createGroup(options,
MainArgs.Feature.FORCE,
MainArgs.Feature.NO_FORCE);
createGroup(options,
MainArgs.Feature.PNG,
MainArgs.Feature.SVG);
}
@Override
protected MainArgs analyze(CommandLine cl) throws ParseException {
final MainArgs margs = new MainArgs();
margs.input = getValueAsResolvedFile(cl, INPUT, IS_FILE);
margs.outputDir = getValueAsResolvedFile(cl, OUTPUT_DIR);
setMask(cl, MainArgs.Feature.class, margs.features::setEnabled);
return margs;
}
@Override
protected Void execute(MainArgs margs) throws IOException {
PumlToAny.execute(margs);
return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy