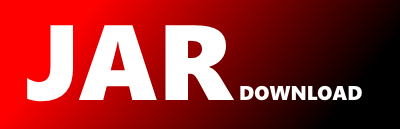
cdc.mf.html.adaptors.IssueAdaptor Maven / Gradle / Ivy
The newest version!
package cdc.mf.html.adaptors;
import java.io.File;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.stringtemplate.v4.Interpreter;
import org.stringtemplate.v4.ST;
import org.stringtemplate.v4.misc.ObjectModelAdaptor;
import org.stringtemplate.v4.misc.STNoSuchPropertyException;
import cdc.issues.Issue;
import cdc.mf.html.MfHtmlGenerationArgs;
import cdc.mf.html.MfHtmlGenerationHint;
import cdc.mf.html.MfParams;
public class IssueAdaptor extends ObjectModelAdaptor {
private final MfHtmlGenerationArgs args;
private static final Pattern DESCRIPTION = Pattern.compile("^description\\((.*)\\)$");
public IssueAdaptor(MfHtmlGenerationArgs args) {
this.args = args;
}
@Override
public synchronized Object getProperty(Interpreter interp,
ST self,
Issue issue,
Object property,
String propertyName) throws STNoSuchPropertyException {
switch (propertyName) {
case "number":
return args.getNumber(issue);
case "htmlId":
return MfParams.getHtmlId(issue, args);
case "id":
return MfParams.getId(issue);
case "issueRef":
// The down path
if (args.getHints().contains(MfHtmlGenerationHint.SINGLE_PAGE)) {
return "#" + MfParams.getHtmlId(issue, args);
} else {
return toString(MfParams.Files.getHtmlIssuesFile()) + "#" + MfParams.getHtmlId(issue, args);
}
case "ruleRef":
// The down path
if (args.getHints().contains(MfHtmlGenerationHint.SINGLE_PAGE)) {
return "#" + MfParams.getHtmlId(issue.getRuleId());
} else {
return toString(MfParams.Files.getHtmlProfileFile()) + "#" + MfParams.getHtmlId(issue.getRuleId());
}
default:
break;
}
final Matcher m = DESCRIPTION.matcher(propertyName);
if (m.matches()) {
final String upPath = m.group(1);
return MfParams.toHtml(issue.getDescription(), upPath, args);
}
return super.getProperty(interp, self, issue, property, propertyName);
}
private static String toString(File file) {
return file == null ? "???" : file.getPath().replace('\\', '/');
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy