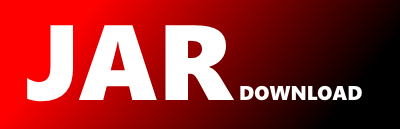
cdc.mf.html.index.Index Maven / Gradle / Ivy
The newest version!
package cdc.mf.html.index;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import cdc.mf.model.MfModel;
import cdc.mf.model.MfNameItem;
public class Index {
private final List sortedKeys = new ArrayList<>();
private final List sortedSections = new ArrayList<>();
private final Map keyToSection = new HashMap<>();
public Index(MfModel model) {
for (final MfNameItem entry : model.collect(MfNameItem.class)) {
final String name = getEffectiveName(entry);
final char key = getKey(name);
final IndexSection section = keyToSection.computeIfAbsent(key, IndexSection::new);
section.add(entry);
}
// Sort keys
sortedKeys.addAll(keyToSection.keySet());
Collections.sort(sortedKeys);
// Sort entries in sections and number sections
int number = 0;
for (final Character key : sortedKeys) {
final IndexSection section = keyToSection.get(key);
section.sort();
section.setNumber(number);
number++;
sortedSections.add(section);
}
}
static String getEffectiveName(MfNameItem item) {
final String name = item.getName();
if (name == null || name.isEmpty()) {
return "?";
} else {
return name;
}
}
static char getKey(String name) {
return Character.toUpperCase(name.charAt(0));
}
public List getKeys() {
return sortedKeys;
}
public List getSections() {
return sortedSections;
}
public IndexSection getSection(char key) {
return keyToSection.get(key);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy