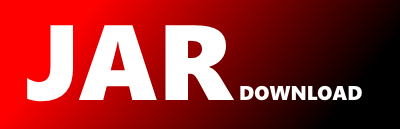
cdc.mf.model.io.MfModelToOffice Maven / Gradle / Ivy
The newest version!
package cdc.mf.model.io;
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.stream.Collectors;
import cdc.args.Strictness;
import cdc.issues.Meta;
import cdc.mf.model.MfAggregation;
import cdc.mf.model.MfAssociation;
import cdc.mf.model.MfCardinality;
import cdc.mf.model.MfClass;
import cdc.mf.model.MfComposition;
import cdc.mf.model.MfConnector;
import cdc.mf.model.MfDecoratedElement;
import cdc.mf.model.MfDocumentation;
import cdc.mf.model.MfElement;
import cdc.mf.model.MfEnumeration;
import cdc.mf.model.MfInterface;
import cdc.mf.model.MfModel;
import cdc.mf.model.MfNameItem;
import cdc.mf.model.MfPackage;
import cdc.mf.model.MfProperty;
import cdc.mf.model.MfQNameItem;
import cdc.mf.model.MfTag;
import cdc.mf.model.MfTagOwner;
import cdc.mf.model.MfType;
import cdc.mf.model.MfTypeOwner;
import cdc.mf.model.MfUtils;
import cdc.office.ss.WorkbookWriter;
import cdc.office.ss.WorkbookWriterFactory;
import cdc.office.ss.WorkbookWriterFeatures;
import cdc.office.tables.TableSection;
import cdc.util.lang.Checks;
/**
* Utility used to produce an Office (Excel, ...) view of an {@link MfModel}.
*
* @author Damien Carbonne
*/
public final class MfModelToOffice {
private static final String ABSTRACTNESS = "Abstractness";
private static final String AGGREGATIONS = "Aggregations";
private static final String ALL_EXTENDED_BY_NAME = "All Extended By (Name)";
private static final String ALL_EXTENDED_BY_QNAME = "All Extended By (QName)";
private static final String ALL_EXTENDS_NAME = "All Extends (Name)";
private static final String ALL_EXTENDS_QNAME = "All Extends (QName)";
private static final String ALL_IMPLEMENTED_BY_NAME = "All Implemented By (Name)";
private static final String ALL_IMPLEMENTED_BY_QNAME = "All Implemented By (QName)";
private static final String ALL_IMPLEMENTS_NAME = "All Implements (Name)";
private static final String ALL_IMPLEMENTS_QNAME = "All Implements (QName)";
private static final String ASSOCIATIONS = "Associations";
private static final String ATTRIBUTES = "Attributes";
private static final String CARDINALITY = "Cardinality";
private static final String CLASSES = "Classes";
private static final String COMPOSITIONS = "Compositions";
private static final String DOCUMENTATIONS = "Documentations";
private static final String EXTENDED_BY_NAME = "Extended By (Name)";
private static final String EXTENDED_BY_QNAME = "Extended By (QName)";
private static final String EXTENDS_NAME = "Extends (Name)";
private static final String EXTENDS_QNAME = "Extends (QName)";
private static final String GUID = "Guid";
private static final String ID = "Id";
private static final String IMPLEMENTED_BY_NAME = "Implemented By (Name)";
private static final String IMPLEMENTED_BY_QNAME = "Implemented By (QName)";
private static final String IMPLEMENTS_NAME = "Implements (Name)";
private static final String IMPLEMENTS_QNAME = "Implements (QName)";
private static final String INTERFACES = "Interfaces";
private static final String KIND = "Kind";
private static final String METAS = "Metas";
private static final String NAME = "Name";
private static final String ORIGIN = "Origin";
private static final String ORIGIN_ID = "Origin Id";
private static final String ORIGIN_NAME = "Origin Name";
private static final String ORIGIN_QNAME = "Origin QName";
private static final String PACKAGES = "Packages";
private static final String PARENT_ID = "Parent Id";
private static final String PARENT_NAME = "Parent Name";
private static final String PARENT_KIND = "Parent Kind";
private static final String PARENT_QNAME = "Parent QName";
private static final String PARENT_STEREOTYPES = "Parent Stereotypes";
private static final String QNAME = "QName";
private static final String SOURCE_CARDINALITY = "Source Cardinality";
private static final String SOURCE_NAVIGABILITY = "Source Navigability";
private static final String SOURCE_OBJECT_ID = "Source Object Id";
private static final String SOURCE_OBJECT_KIND = "Source Object Kind";
private static final String SOURCE_OBJECT_PATH = "Source Object Path";
private static final String SOURCE_OBJECT_NAME = "Source Object Name";
private static final String SOURCE_OBJECT_QNAME = "Source Object QName";
private static final String SOURCE_OBJECT_STEREOTYPES = "Source Object Stereotypes";
private static final String SOURCE_ORDERING = "Source Ordering";
private static final String SOURCE_ROLE = "Source Role";
private static final String STEREOTYPES = "Stereotypes";
private static final String TAGS = "Tags";
private static final String TARGET_CARDINALITY = "Target Cardinality";
private static final String TARGET_NAVIGABILITY = "Target Navigability";
private static final String TARGET_OBJECT_ID = "Target Object Id";
private static final String TARGET_OBJECT_KIND = "Target Object Kind";
private static final String TARGET_OBJECT_PATH = "Target Object Path";
private static final String TARGET_OBJECT_NAME = "Target Object Name";
private static final String TARGET_OBJECT_QNAME = "Target Object QName";
private static final String TARGET_OBJECT_STEREOTYPES = "Target Object Stereotypes";
private static final String TARGET_ORDERING = "Target Ordering";
private static final String TARGET_ROLE = "Target Role";
private static final String TYPE_ID = "Type Id";
private static final String TYPE_KIND = "Type Kind";
private static final String TYPE_NAME = "Type Name";
private static final String TYPE_QNAME = "Type QName";
private static final String TYPE_STEREOTYPES = "Type Stereotypes";
private static final String ABSTRACT = "abstract";
private static final String CONCRETE = "concrete";
private static final String LOCAL = "local";
private static final String INHERITED = "inherited";
private final MfModel model;
private final File file;
private final WorkbookWriterFeatures features;
public MfModelToOffice(MfModel model,
File file,
WorkbookWriterFeatures features) {
this.model = Checks.isNotNull(model, "model");
this.file = Checks.isNotNull(file, "file");
this.features = Checks.isNotNull(features, "features");
}
public void generate() throws IOException {
final WorkbookWriterFactory factory = new WorkbookWriterFactory();
factory.setEnabled(WorkbookWriterFactory.Hint.POI_STREAMING, true);
try (WorkbookWriter> writer = factory.create(file, features)) {
dumpPackages(writer);
dumpClasses(writer);
dumpInterfaces(writer);
dumpAttributes(writer);
dumpConnections(writer);
dumpImplementations(writer);
writer.flush();
}
}
private static void appendDocumentations(MfElement owner,
StringBuilder builder,
int level) {
boolean first = true;
for (final MfDocumentation child : owner.getDocumentations()) {
if (first) {
first = false;
} else {
builder.append("\n");
}
builder.append(child.getText());
appendTags(child, builder, level + 1);
}
}
private static void appendTags(MfTagOwner owner,
StringBuilder builder,
int level) {
boolean first = true;
for (final MfTag child : owner.getTags()) {
if (first) {
first = false;
} else {
builder.append("\n");
}
builder.append(child.getName())
.append(':')
.append(child.getValue());
appendDocumentations(child, builder, level + 1);
}
}
private static void writeDocumentations(WorkbookWriter> writer,
MfElement owner) throws IOException {
if (owner.hasDocumentations()) {
final StringBuilder builder = new StringBuilder();
appendDocumentations(owner, builder, 0);
writer.addCell(builder.toString());
} else {
writer.addEmptyCell();
}
}
private static void writeTags(WorkbookWriter> writer,
MfTagOwner owner) throws IOException {
if (owner.hasTags()) {
final StringBuilder builder = new StringBuilder();
appendTags(owner, builder, 0);
writer.addCell(builder.toString());
} else {
writer.addEmptyCell();
}
}
private static void writeMetas(WorkbookWriter> writer,
MfElement owner) throws IOException {
if (owner.getMetas().isEmpty()) {
writer.addEmptyCell();
} else {
final StringBuilder builder = new StringBuilder();
boolean first = true;
for (final Meta meta : owner.getMetas().getSortedMetas()) {
if (first) {
first = false;
} else {
builder.append("\n");
}
builder.append(meta.getName() + ":" + meta.getValue());
}
writer.addCell(builder.toString());
}
}
private static void writeNames(WorkbookWriter> writer,
Collection extends MfNameItem> objects) throws IOException {
final List list = new ArrayList<>(objects);
list.sort(MfNameItem.NAME_COMPARATOR);
boolean first = true;
final StringBuilder builder = new StringBuilder();
for (final MfNameItem object : list) {
if (first) {
first = false;
} else {
builder.append("\n");
}
builder.append(object.getName());
}
writer.addCell(builder.toString());
}
private static void writeQNames(WorkbookWriter> writer,
Collection extends MfQNameItem> objects) throws IOException {
final List list = new ArrayList<>(objects);
list.sort(MfQNameItem.QNAME_COMPARATOR);
boolean first = true;
final StringBuilder builder = new StringBuilder();
for (final MfQNameItem object : list) {
if (first) {
first = false;
} else {
builder.append("\n");
}
builder.append(object.getQName());
}
writer.addCell(builder.toString());
}
private static String format(Collection c) {
return c.stream()
.sorted()
.collect(Collectors.joining(", "));
}
private void dumpPackages(WorkbookWriter> writer) throws IOException {
writer.beginSheet(PACKAGES);
writer.addRow(TableSection.HEADER,
KIND,
ID,
GUID,
STEREOTYPES,
NAME,
METAS,
QNAME,
PARENT_KIND,
PARENT_ID,
PARENT_STEREOTYPES,
PARENT_NAME,
PARENT_QNAME,
TAGS,
DOCUMENTATIONS,
CLASSES,
INTERFACES,
ATTRIBUTES,
COMPOSITIONS,
AGGREGATIONS,
ASSOCIATIONS);
for (final MfPackage pack : model.collect(MfPackage.class).stream().sorted(MfQNameItem.QNAME_COMPARATOR)
.toList()) {
final MfPackage parent = pack.getParent() instanceof final MfPackage x ? x : null;
writer.beginRow(TableSection.DATA);
writer.addCell(pack.getKind());
writer.addCell(pack.getId());
writer.addCell(pack.getGuid());
writer.addCell(format(pack.getStereotypes()));
writer.addCell(pack.getName());
writeMetas(writer, pack);
writer.addCell(pack.getQName());
if (parent == null) {
writer.addEmptyCells(5);
} else {
writer.addCell(parent.getKind());
writer.addCell(parent.getId());
writer.addCell(format(parent.getStereotypes()));
writer.addCell(parent.getName());
writer.addCell(parent.getQName());
}
writeTags(writer, pack);
writeDocumentations(writer, pack);
writer.addCell(pack.getChildren()
.stream()
.filter(MfClass.class::isInstance)
.count());
writer.addCell(pack.getChildren()
.stream()
.filter(MfInterface.class::isInstance)
.count());
writer.addEmptyCell();
writer.addCell(model.collect(MfComposition.class)
.stream()
.filter(x -> x.getSourceTip().getType().getParent() == pack)
.count());
writer.addCell(model.collect(MfAggregation.class)
.stream()
.filter(x -> x.getSourceTip().getType().getParent() == pack)
.count());
writer.addCell(model.collect(MfAssociation.class)
.stream()
.filter(x -> x.getSourceTip().getType().getParent() == pack)
.count());
}
}
private void dumpClasses(WorkbookWriter> writer) throws IOException {
writer.beginSheet(CLASSES);
writer.addRow(TableSection.HEADER,
KIND,
ID,
GUID,
STEREOTYPES,
NAME,
METAS,
QNAME,
PARENT_KIND,
PARENT_ID,
PARENT_STEREOTYPES,
PARENT_NAME,
PARENT_QNAME,
ABSTRACTNESS,
EXTENDS_NAME,
EXTENDS_QNAME,
ALL_EXTENDS_NAME,
ALL_EXTENDS_QNAME,
IMPLEMENTS_NAME,
IMPLEMENTS_QNAME,
ALL_IMPLEMENTS_NAME,
ALL_IMPLEMENTS_QNAME,
EXTENDED_BY_NAME,
EXTENDED_BY_QNAME,
ALL_EXTENDED_BY_NAME,
ALL_EXTENDED_BY_QNAME,
TAGS,
DOCUMENTATIONS);
for (final MfClass x : model.collect(MfClass.class).stream().sorted(MfQNameItem.QNAME_COMPARATOR)
.toList()) {
final MfTypeOwner parent = x.getParent();
writer.beginRow(TableSection.DATA);
writer.addCell(x.getKind());
writer.addCell(x.getId());
writer.addCell(x.getGuid());
writer.addCell(format(x.getStereotypes()));
writer.addCell(x.getName());
writeMetas(writer, x);
writer.addCell(x.getQName());
writer.addCell(parent.getKind());
writer.addCell(parent.getId());
writer.addCell(format(parent.getStereotypes()));
writer.addCell(parent.getName());
writer.addCell(parent.getQName());
writer.addCell(x.isAbstract() ? ABSTRACT : CONCRETE);
writeNames(writer, x.getDirectAncestors(MfClass.class));
writeQNames(writer, x.getDirectAncestors(MfClass.class));
writeNames(writer, x.getAllAncestors(Strictness.STRICT, MfClass.class));
writeQNames(writer, x.getAllAncestors(Strictness.STRICT, MfClass.class));
writeNames(writer, x.getDirectProGenitors());
writeQNames(writer, x.getDirectProGenitors());
writeNames(writer, x.getAllAncestors(Strictness.STRICT, MfInterface.class));
writeQNames(writer, x.getAllAncestors(Strictness.STRICT, MfInterface.class));
writeNames(writer, x.getDirectDescendants());
writeQNames(writer, x.getDirectDescendants());
writeNames(writer, x.getAllDescendants(Strictness.STRICT));
writeQNames(writer, x.getAllDescendants(Strictness.STRICT));
writeTags(writer, x);
writeDocumentations(writer, x);
}
}
private void dumpInterfaces(WorkbookWriter> writer) throws IOException {
writer.beginSheet(INTERFACES);
writer.addRow(TableSection.HEADER,
KIND,
ID,
GUID,
STEREOTYPES,
NAME,
METAS,
QNAME,
PARENT_KIND,
PARENT_ID,
PARENT_STEREOTYPES,
PARENT_NAME,
PARENT_QNAME,
EXTENDS_NAME,
EXTENDS_QNAME,
ALL_EXTENDS_NAME,
ALL_EXTENDS_QNAME,
EXTENDED_BY_NAME,
EXTENDED_BY_QNAME,
ALL_EXTENDED_BY_NAME,
ALL_EXTENDED_BY_QNAME,
IMPLEMENTED_BY_NAME,
IMPLEMENTED_BY_QNAME,
ALL_IMPLEMENTED_BY_NAME,
ALL_IMPLEMENTED_BY_QNAME,
TAGS,
DOCUMENTATIONS);
for (final MfInterface x : model.collect(MfInterface.class).stream().sorted(MfQNameItem.QNAME_COMPARATOR)
.toList()) {
final MfTypeOwner parent = x.getParent();
writer.beginRow(TableSection.DATA);
writer.addCell(x.getKind());
writer.addCell(x.getId());
writer.addCell(x.getGuid());
writer.addCell(format(x.getStereotypes()));
writer.addCell(x.getName());
writeMetas(writer, x);
writer.addCell(x.getQName());
writer.addCell(parent.getKind());
writer.addCell(parent.getId());
writer.addCell(format(parent.getStereotypes()));
writer.addCell(parent.getName());
writer.addCell(parent.getQName());
writeNames(writer, x.getDirectAncestors());
writeQNames(writer, x.getDirectAncestors());
writeNames(writer, x.getAllAncestors(Strictness.STRICT));
writeQNames(writer, x.getAllAncestors(Strictness.STRICT));
writeNames(writer, x.getDirectDescendants(MfInterface.class));
writeQNames(writer, x.getDirectDescendants(MfInterface.class));
writeNames(writer, x.getAllDescendants(Strictness.STRICT, MfInterface.class));
writeQNames(writer, x.getAllDescendants(Strictness.STRICT, MfInterface.class));
writeNames(writer, x.getDirectImplementors());
writeQNames(writer, x.getDirectImplementors());
writeNames(writer, x.getAllImplementors());
writeQNames(writer, x.getAllImplementors());
writeTags(writer, x);
writeDocumentations(writer, x);
}
}
private static void dumpAttribute(WorkbookWriter> writer,
MfProperty att,
MfType owner,
MfType ancestor) throws IOException {
writer.beginRow(TableSection.DATA);
writer.addCell(att.getKind());
writer.addCell(att.getId());
writer.addCell(att.getGuid());
writer.addCell(format(att.getStereotypes()));
writer.addCell(att.getName());
writeMetas(writer, att);
writer.addCell(owner.getQName() + "@" + att.getName());
writer.addCell(owner.getKind());
writer.addCell(owner.getId());
writer.addCell(format(owner.getStereotypes()));
writer.addCell(owner.getName());
writer.addCell(owner.getQName());
if (ancestor == null) {
writer.addCell(LOCAL);
} else {
writer.addCell(INHERITED);
}
if (ancestor == null) {
writer.addEmptyCells(3);
} else {
writer.addCell(ancestor.getId());
writer.addCell(ancestor.getName());
writer.addCell(ancestor.getQName());
}
if (att.getCardinality() == null) {
writer.addEmptyCell();
} else {
writer.addCell(att.getCardinality().toExpandedString());
}
if (att.hasValidType()) {
writer.addCell(att.getType().getKind());
writer.addCell(att.getType().getId());
writer.addCell(format(att.getType().getStereotypes()));
writer.addCell(att.getType().getName());
writer.addCell(att.getType().getQName());
} else {
writer.addEmptyCells(5);
}
writeTags(writer, att);
writeDocumentations(writer, att);
}
private void dumpAttributes(WorkbookWriter> writer) throws IOException {
writer.beginSheet(ATTRIBUTES);
writer.addRow(TableSection.HEADER,
KIND,
ID,
GUID,
STEREOTYPES,
NAME,
METAS,
QNAME,
PARENT_KIND,
PARENT_ID,
PARENT_STEREOTYPES,
PARENT_NAME,
PARENT_QNAME,
ORIGIN,
ORIGIN_ID,
ORIGIN_NAME,
ORIGIN_QNAME,
CARDINALITY,
TYPE_KIND,
TYPE_ID,
TYPE_STEREOTYPES,
TYPE_NAME,
TYPE_QNAME,
TAGS,
DOCUMENTATIONS);
for (final MfType owner : model.collect(MfType.class).stream().sorted(MfQNameItem.QNAME_COMPARATOR)
.toList()) {
for (final MfProperty att : owner.getProperties()) {
dumpAttribute(writer, att, owner, null);
}
for (final MfType ancestor : owner.getAllAncestors(Strictness.STRICT)
.stream()
.sorted(MfQNameItem.QNAME_COMPARATOR)
.toList()) {
for (final MfProperty att : ancestor.getProperties()) {
dumpAttribute(writer, att, owner, ancestor);
}
}
}
}
private static void dumpConnector(WorkbookWriter> writer,
MfConnector connector,
MfType sourceDescendant,
MfType targetDescendant) throws IOException {
writer.beginRow(TableSection.DATA);
writer.addCell(connector.getKind());
writer.addCell(connector.getId());
writer.addCell(connector.getGuid());
writer.addCell(format(connector.getStereotypes()));
writer.addCell(connector.getName());
writeMetas(writer, connector);
writeTags(writer, connector);
writeDocumentations(writer, connector);
if (sourceDescendant == null && targetDescendant == null) {
writer.addCell(LOCAL);
} else {
writer.addCell(INHERITED);
}
writer.addCell(connector.getSourceTip().getRole());
writer.addCell(MfUtils.toNavigability(connector.getSourceTip().isNavigable()));
writer.addCell(MfCardinality.toExpandedString(connector.getSourceTip().getCardinality()));
writer.addCell(MfUtils.toOrdering(connector.getSourceTip().isOrdered()));
if (sourceDescendant == null) {
writer.addCell(connector.getSourceTip().getType().getKind());
writer.addCell(connector.getSourceTip().getType().getId());
writer.addCell(format(connector.getSourceTip().getType().getStereotypes()));
writer.addCell(connector.getSourceTip().getType().getQName().getParent().normalize());
writer.addCell(connector.getSourceTip().getType().getName());
writer.addCell(connector.getSourceTip().getType().getQName());
} else {
writer.addCell(sourceDescendant.getKind());
writer.addCell(sourceDescendant.getId());
writer.addCell(format(sourceDescendant.getStereotypes()));
writer.addCell(sourceDescendant.getQName().getParent().normalize());
writer.addCell(sourceDescendant.getName());
writer.addCell(sourceDescendant.getQName());
}
writer.addCell(connector.getTargetTip().getRole());
writer.addCell(MfUtils.toNavigability(connector.getTargetTip().isNavigable()));
writer.addCell(MfCardinality.toExpandedString(connector.getTargetTip().getCardinality()));
writer.addCell(MfUtils.toOrdering(connector.getTargetTip().isOrdered()));
if (targetDescendant == null) {
writer.addCell(connector.getTargetTip().getType().getKind());
writer.addCell(connector.getTargetTip().getType().getId());
writer.addCell(format(connector.getTargetTip().getType().getStereotypes()));
writer.addCell(connector.getTargetTip().getType().getQName().getParent().normalize());
writer.addCell(connector.getTargetTip().getType().getName());
writer.addCell(connector.getTargetTip().getType().getQName());
} else {
writer.addCell(targetDescendant.getKind());
writer.addCell(targetDescendant.getId());
writer.addCell(format(targetDescendant.getStereotypes()));
writer.addCell(targetDescendant.getQName().getParent().normalize());
writer.addCell(targetDescendant.getName());
writer.addCell(targetDescendant.getQName());
}
}
private void dumpConnections(WorkbookWriter> writer) throws IOException {
writer.beginSheet("Connections");
writer.addRow(TableSection.HEADER,
KIND,
ID,
GUID,
STEREOTYPES,
NAME,
METAS,
TAGS,
DOCUMENTATIONS,
ORIGIN,
SOURCE_ROLE,
SOURCE_NAVIGABILITY,
SOURCE_CARDINALITY,
SOURCE_ORDERING,
SOURCE_OBJECT_KIND,
SOURCE_OBJECT_ID,
SOURCE_OBJECT_STEREOTYPES,
SOURCE_OBJECT_PATH,
SOURCE_OBJECT_NAME,
SOURCE_OBJECT_QNAME,
TARGET_ROLE,
TARGET_NAVIGABILITY,
TARGET_CARDINALITY,
TARGET_ORDERING,
TARGET_OBJECT_KIND,
TARGET_OBJECT_ID,
TARGET_OBJECT_STEREOTYPES,
TARGET_OBJECT_PATH,
TARGET_OBJECT_NAME,
TARGET_OBJECT_QNAME);
final List connectors = model.collect(MfConnector.class);
connectors.sort(MfElement.ID_COMPARATOR);
for (final MfConnector connector : connectors) {
dumpConnector(writer, connector, null, null);
for (final MfType descendant : connector.getSourceTip().getType().getAllDescendants(Strictness.STRICT)) {
dumpConnector(writer, connector, descendant, null);
}
for (final MfType descendant : connector.getTargetTip().getType().getAllDescendants(Strictness.STRICT)) {
dumpConnector(writer, connector, null, descendant);
}
}
}
private void dumpImplementations(WorkbookWriter> writer) throws IOException {
writer.beginSheet("Implementations");
final List xfces = model.collect(MfInterface.class);
Collections.sort(xfces, MfQNameItem.NAME_PARENT_PATH_COMPARATOR);
final List types = model.collect(MfType.class, x -> x instanceof MfClass || x instanceof MfEnumeration);
Collections.sort(types, MfQNameItem.NAME_PARENT_PATH_COMPARATOR);
writer.beginRow(TableSection.HEADER);
writer.addCell(KIND);
writer.addCell(ID);
writer.addCell(GUID);
writer.addCell("Type");
writer.addCell("Count");
for (final MfInterface xfce : xfces) {
writer.addCell(xfce.getId() + "\n" + xfce.getName());
}
for (final MfType type : types) {
writer.beginRow(TableSection.DATA);
writer.addCell(type.getKind());
writer.addCell(type.getId());
writer.addCell(type.getGuid());
writer.addCell(type.getName());
final Set> set = type.getDecoratedAncestors(MfInterface.class);
writer.addCell(set.size());
final Map map = new HashMap<>();
for (final MfDecoratedElement d : set) {
map.put(d.getElement(), d.isDirect());
}
for (final MfInterface xfce : xfces) {
final Boolean direct = map.get(xfce);
if (direct == null) {
writer.addEmptyCell();
} else if (direct.booleanValue()) {
writer.addCell("D");
} else {
writer.addCell("I");
}
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy