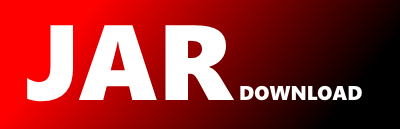
cdc.perfs.io.PerfsIo Maven / Gradle / Ivy
package cdc.perfs.io;
import java.io.File;
import java.io.IOException;
import java.net.URL;
import cdc.perfs.Environment;
import cdc.perfs.api.MeasureLevel;
import cdc.perfs.api.RuntimeProbe;
import cdc.perfs.api.Source;
import cdc.perfs.runtime.RuntimeEnvironment;
import cdc.perfs.snapshot.SnapshotEnvironment;
import cdc.util.compress.Compressor;
import cdc.util.data.xml.XmlDataReader;
import cdc.util.lang.Checks;
import cdc.util.lang.FailureReaction;
public final class PerfsIo {
private static final Source SOURCE = RuntimeEnvironment.getInstance().getSource(PerfsIo.class);
public static final String STAR = "*";
public static final String DAT = ".dat";
public static final String DAT_GZ = ".dat.gz";
public static final String XML = ".xml";
public static final String XML_GZ = ".xml.gz";
public static final String COMPACT_XML = ".cp.xml";
public static final String COMPACT_XML_GZ = ".cp.xml.gz";
private static final String[] EXTENSIONS = {
DAT,
DAT_GZ,
XML,
XML_GZ,
COMPACT_XML,
COMPACT_XML_GZ
};
private static final String[] STAR_EXTENSIONS = {
STAR + DAT,
STAR + DAT_GZ,
STAR + XML,
STAR + XML_GZ,
STAR + COMPACT_XML,
STAR + COMPACT_XML_GZ,
};
private PerfsIo() {
}
public static String[] getExtensions() {
return EXTENSIONS.clone();
}
public static String[] getStarExtensions() {
return STAR_EXTENSIONS.clone();
}
public static SnapshotEnvironment load(File file) throws IOException {
final RuntimeProbe probe = RuntimeEnvironment.getInstance().createProbe(SOURCE, MeasureLevel.INFO);
probe.start("load(" + file + ")");
try {
Checks.isNotNull(file, "file");
if (file.getPath().endsWith(DAT)) {
final PerfsBin.Reader reader = new PerfsBin.Reader();
return reader.load(file.getPath(), Compressor.NONE);
} else if (file.getPath().endsWith(DAT_GZ)) {
final PerfsBin.Reader reader = new PerfsBin.Reader();
return reader.load(file.getPath(), Compressor.GZIP);
} else if (file.getPath().endsWith(XML) || file.getPath().endsWith(COMPACT_XML)) {
final PerfsXml.Loader loader = new PerfsXml.Loader(FailureReaction.WARN);
return loader.loadXml(file.getPath(),
XmlDataReader.Feature.SHARE_ATTRIBUTE_NAMES,
XmlDataReader.Feature.SHARE_ELEMENT_NAMES);
} else if (file.getPath().endsWith(XML_GZ) || file.getPath().endsWith(COMPACT_XML_GZ)) {
final PerfsXml.Loader loader = new PerfsXml.Loader(FailureReaction.WARN);
return loader.loadXml(file.getPath(),
Compressor.GZIP,
XmlDataReader.Feature.SHARE_ATTRIBUTE_NAMES,
XmlDataReader.Feature.SHARE_ELEMENT_NAMES);
} else {
throw new IOException("Unexpected extension '" + file + "'");
}
} finally {
probe.stop();
}
}
public static SnapshotEnvironment load(URL url) throws IOException {
final RuntimeProbe probe = RuntimeEnvironment.getInstance().createProbe(SOURCE, MeasureLevel.INFO);
probe.start("load(" + url + ")");
try {
Checks.isNotNull(url, "url");
if (url.getPath().endsWith(DAT)) {
final PerfsBin.Reader reader = new PerfsBin.Reader();
return reader.load(url, Compressor.NONE);
} else if (url.getPath().endsWith(DAT_GZ)) {
final PerfsBin.Reader reader = new PerfsBin.Reader();
return reader.load(url, Compressor.GZIP);
} else if (url.getPath().endsWith(XML) || url.getPath().endsWith(COMPACT_XML)) {
final PerfsXml.Loader loader = new PerfsXml.Loader(FailureReaction.WARN);
return loader.loadXml(url);
} else if (url.getPath().endsWith(XML_GZ) || url.getPath().endsWith(COMPACT_XML_GZ)) {
final PerfsXml.Loader loader = new PerfsXml.Loader(FailureReaction.WARN);
return loader.loadXml(url, Compressor.GZIP);
} else {
throw new IOException("Unexpected extension + '" + url + "'");
}
} finally {
probe.stop();
}
}
public static String fixFilename(String filename) {
if (filename.endsWith(DAT)
|| filename.endsWith(DAT_GZ)
|| filename.endsWith(XML)
|| filename.endsWith(XML_GZ)
|| filename.endsWith(COMPACT_XML)
|| filename.endsWith(COMPACT_XML_GZ)) {
return filename;
} else {
return filename + XML;
}
}
/**
* Saves an environment to a file, auto-detecting format from extension.
*
* @param environment The environment.
* @param filename The file name.
* @throws IOException When an IO error occurs.
*/
public static void save(Environment environment,
String filename) throws IOException {
final RuntimeProbe probe = RuntimeEnvironment.getInstance().createProbe(SOURCE, MeasureLevel.INFO);
probe.start("save(" + filename + ")");
try {
if (filename.endsWith(DAT)) {
final PerfsBin.Writer writer = new PerfsBin.Writer();
writer.save(environment, filename, Compressor.NONE);
} else if (filename.endsWith(DAT_GZ)) {
final PerfsBin.Writer writer = new PerfsBin.Writer();
writer.save(environment, filename, Compressor.GZIP);
} else if (filename.endsWith(COMPACT_XML)) {
// Check this before XML
final PerfsXml.Printer printer = new PerfsXml.Printer(PerfsXml.Format.COMPACT);
printer.save(environment, filename, Compressor.NONE);
} else if (filename.endsWith(XML)) {
final PerfsXml.Printer printer = new PerfsXml.Printer();
printer.save(environment, filename, Compressor.NONE);
} else if (filename.endsWith(COMPACT_XML_GZ)) {
// Check this before XML_GZ
final PerfsXml.Printer printer = new PerfsXml.Printer(PerfsXml.Format.COMPACT);
printer.save(environment, filename, Compressor.GZIP);
} else if (filename.endsWith(XML_GZ)) {
final PerfsXml.Printer printer = new PerfsXml.Printer();
printer.save(environment, filename, Compressor.GZIP);
} else {
throw new IOException("Unexpected extension '" + filename + "'");
}
} finally {
probe.stop();
}
}
/**
* Saves an environment to a file, auto-detecting format from extension.
*
* @param environment The environment.
* @param file The file.
* @throws IOException When an IO error occurs.
*/
public static void save(Environment environment,
File file) throws IOException {
save(environment, file.getPath());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy