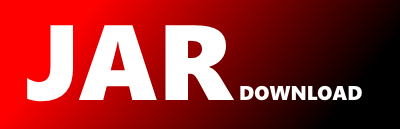
cdc.perfs.snapshot.SnapshotContext Maven / Gradle / Ivy
package cdc.perfs.snapshot;
import cdc.perfs.Context;
import cdc.perfs.Measure;
import cdc.perfs.MeasureStatus;
import cdc.perfs.api.MeasureLevel;
import cdc.perfs.api.Source;
import cdc.perfs.core.AbstractContext;
/**
* Implementation of Context for snapshots.
*
* @author Damien Carbonne
*
*/
public final class SnapshotContext extends AbstractContext {
private long absoluteEnd;
/** Height of this context. */
private int height = 0;
private final boolean alive;
SnapshotContext(SnapshotEnvironment environment,
int id,
String name,
boolean alive) {
super(environment, id, name);
this.alive = alive;
}
SnapshotContext(SnapshotEnvironment environment,
Context context) {
super(environment, context.getId(), context.getName());
this.alive = context.isAlive();
// Clone measures
SnapshotMeasure previous = null;
for (int index = 0; index < context.getRootMeasuresCount(); index++) {
previous = clone(context.getRootMeasure(index), null, 0, previous);
}
}
private SnapshotMeasure clone(Measure measure,
SnapshotMeasure parent,
int parentDepth,
SnapshotMeasure previous) {
final SnapshotMeasure clone = new SnapshotMeasure(measure, parent, previous, this);
height = Math.max(height, parentDepth + 1);
Measure child = measure.getFirstChild();
SnapshotMeasure prev = null;
while (child != null) {
prev = clone(child, clone, parentDepth + 1, prev);
child = child.getNextSibling();
}
return clone;
}
public SnapshotMeasure createMeasure(SnapshotMeasure parent,
int parentDepth,
SnapshotMeasure previous,
Source source,
String details,
long begin,
long end,
MeasureStatus status,
MeasureLevel level) {
final SnapshotMeasure measure =
new SnapshotMeasure(parent, previous, source, details, begin, end, status, level, this);
height = Math.max(height, parentDepth + 1);
return measure;
}
@Override
public SnapshotEnvironment getEnvironment() {
return (SnapshotEnvironment) super.getEnvironment();
}
@Override
public Thread getThread() {
return null;
}
@Override
public boolean isAlive() {
return alive;
}
public void setAbsoluteEndNano(long end) {
absoluteEnd = end;
}
public long getAbsoluteEndNano() {
return absoluteEnd;
}
@Override
public int getHeight() {
return height;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy