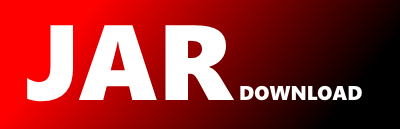
cdc.perfs.core.Context Maven / Gradle / Ivy
package cdc.perfs.core;
import cdc.util.time.TimeMeasureMode;
/**
* A Context is associated to an Environment and a Thread.
*
* A Context is dead when the associated Thread is dead.
* A snapshot context is always dead as its associated thread is null.
*
* @author Damien Carbonne
*/
public interface Context {
/**
* Returns the associated environment.
*
* @return The associated environment.
*/
public Environment getEnvironment();
/**
* Returns the name of this context.
*
* It is the name of the Thread that was associated to this context when it
* was alive.
*
* @return The name of this context.
*/
public String getName();
/**
* Returns the id of this context.
*
* This id is unique in the environment of the context.
* The same value can be reused among environments.
*
* @return The id of this context.
*/
public int getId();
/**
* Returns the thread associated to this context.
*
* Will be null for a dead context.
*
* @return The Thread associated to this context.
*/
public Thread getThread();
/**
* Returns whether this context is alive or not.
*
* An alive context can have new measures in the future.
* A dead context won't change anymore.
* For snapshots, this returns the status of the cloned context at time of
* cloning.
*
* @return True when this context is alive, false otherwise.
*/
public boolean isAlive();
/**
* @return The number of root measures in this context.
*/
public int getRootMeasuresCount();
/**
* @return The number of measures in this context.
*/
public int getMeasuresCount();
/**
* Returns the root measure at index.
*
* @param index The root measure index.
* @return The root measure at index or {@code null} if {@code index} is invalid.
*/
public Measure getRootMeasure(int index);
/**
* @return The height of measures tree of this Context.
*/
public int getHeight();
/**
* Returns the Measure, if any, that exists at a given time and depth with a
* time tolerance.
*
* @param time The absolute or relative nano time at which a measure is
* searched.
* @param mode Time measure mode.
* @param epsilon The nano time tolerance ({@code >= 0}).
* @param depth The measure depth. TODO 0 or 1-based ?
* @return The Measure the exists at given time and depth location.
*/
public Measure getMeasure(long time,
TimeMeasureMode mode,
long epsilon,
int depth);
/**
* Returns the Index of the root that has a given position relatively to a
* reference time.
*
* @param time The absolute or relative reference nano time.
* @param mode Time measure mode.
* @param position The relative position of the searched root.
* @return the index of the matching root, or -1 if none is found.
*/
public int getRootMeasureIndex(long time,
TimeMeasureMode mode,
Position position);
/**
* @return The minimum relative begin nanos of associated measures,
* or current relative time if this context is empty.
*/
public long getMinRelativeBeginNanosOrCurrent();
/**
* @return The maximum relative begin nanos of associated measures,
* or current relative time if last measure is RUNNING.
*/
public long getMaxRelativeEndNanosOrCurrent();
}