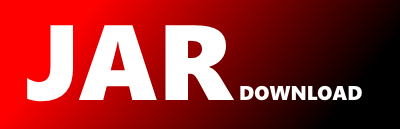
cdc.perfs.core.Measure Maven / Gradle / Ivy
package cdc.perfs.core;
import java.util.Comparator;
import cdc.perfs.api.MeasureLevel;
import cdc.perfs.api.Source;
/**
* Description of a measure.
*
* @author Damine Carbonne
*
*/
public interface Measure {
/**
* A comparator of measures bases on begin time.
*/
public static final Comparator BEGIN_COMPARATOR = (o1,
o2) -> Long.compare(o1.getAbsoluteBeginNanos(), o2.getAbsoluteBeginNanos());
/**
* @return The environment to which this measure belongs.
*/
public Environment getEnvironment();
/**
* @return The associated source.
*/
public Source getSource();
/**
* @return The details associated to this measure.
*/
public String getDetails();
/**
* @return The current status of this measure.
*/
public MeasureStatus getStatus();
/**
* @return The level of this measure.
*/
public MeasureLevel getLevel();
/**
* @return A String built from source name and details (if any).
*/
public String getLabel();
/**
* Returns the depth of this measure.
*
* The height of a root measure is 0.
*
* @return The depth of this measure.
*/
public int getDepth();
/**
* Returns the height of the tree under this measure.
*
* The height of a measure that has no children is 1.
*
* @return The height of the tree under this measure.
*/
public int getHeight();
/**
* @return The absolute beginning nano time of this measure.
*/
public long getAbsoluteBeginNanos();
/**
* @return The relative beginning nano time of this measure.
*/
public long getRelativeBeginNanos();
/**
* Returns the absolute end nano time of this measure.
*
* When status is RUNNING, this is meaningless.
* It is only meaningful when status is frozen.
*
* @return The absolute end nano time of this measure.
*/
public long getAbsoluteEndNanos();
/**
* Returns the absolute end or current nano time of this measure.
*
* When status is RUNNING, returns absolute current nano time.
* Otherwise, returns absolute current nano time.
*
* @return The absolute end or current nano time of this measure.
*/
public long getAbsoluteEndOrCurrentNanos();
/**
* Returns the relative end nano time of this measure.
*
* When status is RUNNING, this is meaningless.
* It is only meaningful when status is frozen.
*
* @return The relative end nano time of this measure.
*/
public long getRelativeEndNanos();
/**
* Returns the relative end or current nano time of this measure.
*
* When status is RUNNING, returns relative current nano time.
* Otherwise, returns relative end nano time.
*
* @return The relative end or current nano time of this measure.
*/
public long getRelativeEndOrCurrentNanos();
/**
* Returns the duration of this measurement.
*
* If the measure is frozen, then return the frozen value, that is
* the difference between the end and the begin value.
* If the measure is still running, compute the difference between
* current System nanoTime and the begin value.
*
* @return the duration of this measurement.
*/
public long getElapsedNanos();
/**
* @return The first child measure or null.
*/
public Measure getFirstChild();
/**
* @return The last child, if any, or null.
*/
public Measure getLastChild();
/**
* @return The number of children measures.
*/
public int getChildrenCount();
/**
* Returns the index-th (0-based) child measure.
*
* If index is negative or too large, return null.
*
* @param index Index of the searched child.
* @return The corresponding child or null.
*/
public Measure getChild(int index);
/**
* @return The next sibling measure or null.
*/
public Measure getNextSibling();
/**
* Returns the first child, if any, whose time extent is totally included in
* a given time interval.
*
* @param inf Lower bound (inclusive) of the time interval.
* @param sup Higher bound (inclusive) of the time interval.
* @return The corresponding child or null.
*/
public Measure getFirstChild(long inf,
long sup);
public Measure getFirstSubChild(int level,
long inf,
long sup);
/**
* Returns the span position of the measure.
*
* @param inf Absolute lower bound of the time interval.
* @param sup Absolute upper bound of the time interval.
* @return The span position of this measure relatively to {@code [low, high]}.
*/
public SpanPosition getPosition(long inf,
long sup);
}