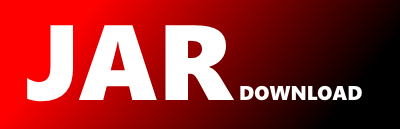
cdc.perfs.core.Environment Maven / Gradle / Ivy
package cdc.perfs.core;
import java.time.Instant;
import java.util.Collection;
import java.util.List;
import cdc.perfs.api.Source;
import cdc.util.time.TimeMeasureMode;
/**
* Base interface for Environments.
*
* An environment is an organization of Measures.
* A Runtime environment allows creation of new Measures.
* A Snapshot environment does not allow creation of measures.
* Each Measure is created in a Context, by a Source at a given Level.
* A Context corresponds to a Thread.
* A Source defines a type of measures.
* Level defines the importance of the Measure.
*
* Time can be expressed in nanoseconds relatively to the environment reference time or as an 'absolute' time.
* 'absolute' time corresponds to the value returned by {@link System#nanoTime()}.
*
* @author Damien Carbonne
*/
public interface Environment {
/**
* @return The kind of this environment.
*/
public EnvironmentKind getKind();
/**
* Adds an EnvironmentListener to this Environment.
*
* Adding several times the same listener is supported.
* In that case, it will be triggered as many times it is registered.
*
* @param listener The listener to add.
*/
public void addListener(EnvironmentListener listener);
/**
* Removes an EnvironmentListener from this Environment.
*
* If this listener was not registered, then nothing is done.
*
* @param listener The listener to remove.
*/
public void removeListener(EnvironmentListener listener);
/**
* @return The reference (absolute) nano time value.
*/
public long getReferenceNanos();
/**
* Returns the current nano time.
*
* For a Runtime environment, this is equivalent to System.nanoTime.
* For a Snapshot environment, this value is frozen.
*
* @return The current nano time.
*/
public long getCurrentNanos();
/**
* Returns elapsed time since reference time.
*
* For a Runtime environment, this is elapsed time between start time and
* current time.
*
* For a snapshot environment, this is elapsed time between start time and
* end time of the corresponding application.
*
* @return The elapsed time since reference time.
*/
public long getElapsedNanos();
/**
* Converts a relative time to absolute time.
*
* @param time The relative time. It must be relative for this Environment.
* @return The absolute time value corresponding to time.
*/
public long toAbsolute(long time);
/**
* Converts a relative or absolute time to absolute time.
*
* Note: {@code toAbsolute(v, TimeMeasureMode.ABSOLUTE) == v}
*
* @param time The absolute or relative time.
* @param mode The absoluteness / relativeness of time.
* @return The absolute time value corresponding to time.
*/
public long toAbsolute(long time,
TimeMeasureMode mode);
/**
* Converts an absolute time to a relative time.
*
* @param time The absolute time.
* @return The relative time value corresponding to time.
*/
public long toRelative(long time);
/**
* Converts a relative or absolute time to the relative time equivalence.
*
* Note: {@code toRelative(v, TimeMeasureMode.RELATIVE) == v}
*
* @param time The absolute or relative time.
* @param mode The absoluteness / relativeness of time.
* @return The relative time value corresponding to time.
*/
public long toRelative(long time,
TimeMeasureMode mode);
/**
* @return The reference date.
*/
public Instant getReferenceInstant();
/**
* @return A List of declared contexts.
*/
public List extends Context> getContexts();
/**
* Remove all measures of all contexts.
*/
public void removeAllMeasures();
/**
* Removes all contexts that are dead (not alive).
*/
public void removeDeadContexts();
/**
* Returns the shared source that has a given name.
*
* If a source with that name already exists, then it returns it.
* Otherwise, creates a new Source and returns it.
*
* @param name Name of the source.
* @return The shared source that is named name.
*/
public Source getSource(String name);
/**
* Returns the source associated to a class.
*
* Equivalent to getSource(klass.getCanonicalName())
*
* @param cls The class whose name will be associated to source.
* @return getSource(klass.getCanonicalName())
*/
public default Source getSource(Class> cls) {
return getSource(cls.getCanonicalName());
}
/**
* @return A collection of all Sources declared in this environment.
*/
public Collection extends Source> getSources();
/**
* @return The number of measures in this environment.
*/
public default int getMeasuresCount() {
int total = 0;
for (final Context context : getContexts()) {
total += context.getMeasuresCount();
}
return total;
}
}