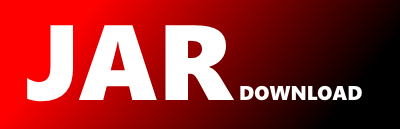
cdc.perfs.core.io.IoExtension Maven / Gradle / Ivy
package cdc.perfs.core.io;
import java.util.ArrayList;
import java.util.List;
public enum IoExtension {
DAT("dat", "Perfs binary files"),
DAT_GZ("dat.gz", "Perfs compress binary files"),
XML("xml", "Perfs XML files"),
XML_GZ("xml.gz", "Perfs compressed XML files"),
COMPACT_XML("cp.xml", "Perfs compact XML files"),
COMPACT_XML_GZ("cp.xml.gz", "Perfs compressed compact XML files"),
CSV("csv", "CSV"),
XLSX("xlsx", "XLSX"),
ODS("ods", "ODS");
private final String label;
private final String description;
private IoExtension(String label,
String description) {
this.label = label;
this.description = description;
}
public String getLabel() {
return label;
}
public String getDescription() {
return description;
}
public boolean isSupported(IoMode mode) {
if (mode == IoMode.EXPORT) {
return true;
} else {
return this != CSV && this != XLSX && this != ODS;
}
}
public static String[] getExtensions(String prefix,
IoMode mode) {
final List list = new ArrayList<>();
for (final IoExtension value : IoExtension.values()) {
if (value.isSupported(mode)) {
list.add(prefix + value.getLabel());
}
}
return list.toArray(new String[list.size()]);
}
public static String getExtensionsString(String prefix,
IoMode mode) {
final StringBuilder builder = new StringBuilder();
boolean first = true;
for (final IoExtension value : IoExtension.values()) {
if (value.isSupported(mode)) {
if (first) {
first = false;
} else {
builder.append(",");
}
builder.append(prefix);
builder.append(value.getLabel());
}
}
return builder.toString();
}
public static String fixFilename(String filename) {
for (final IoExtension value : IoExtension.values()) {
if (filename.endsWith("." + value.getLabel())) {
return filename;
}
}
return filename + "." + XML.getLabel();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy