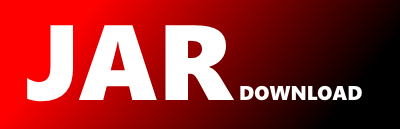
cdc.perfs.demos.PerfsSupport Maven / Gradle / Ivy
package cdc.perfs.demos;
import java.util.Random;
import cdc.perfs.api.RuntimeManager;
import cdc.perfs.api.RuntimeProbe;
import cdc.perfs.api.Source;
/**
* Class used to generate periodic measures.
* A degree of randomness can be added.
* A depth of recursion can be also defined.
*
* @author Damien Carbonne
*
*/
public final class PerfsSupport {
private static int sourceCount = 0;
private final Source source = RuntimeManager.getSource("Test" + sourceCount++);
private final Random random = new Random();
private final boolean randomValues;
private final boolean randomErrors;
private final int maxDepth;
private int count = 0;
private int getValue(int ref) {
if (randomValues) {
final double k = this.random.nextDouble();
return (int) ((0.5 + k) * ref);
} else {
return ref;
}
}
private boolean generateError() {
if (randomErrors) {
final double k = this.random.nextDouble();
return k <= 0.1;
} else {
return false;
}
}
private int getInt(int ref) {
if (ref <= 1) {
return ref;
} else {
return random.nextInt(ref);
}
}
protected static void sleep(int millis) {
try {
if (millis > 0) {
Thread.sleep(millis);
}
} catch (final InterruptedException e) {
// Ignore
}
}
protected PerfsSupport(int max,
int activeMillis,
int passiveMillis,
int maxDepth,
boolean randomValues,
boolean randomErrors) {
this.randomValues = randomValues;
this.randomErrors = randomErrors;
this.maxDepth = maxDepth;
// Possible infinite loop here. This is expected.
for (int index = 0; max <= 0 || index < max; index++) {
recurse(0, activeMillis, passiveMillis);
}
}
private void recurse(int depth,
int activeMillis,
int passiveMillis) {
final RuntimeProbe probe = RuntimeManager.createProbe(source);
count++;
if (count % 10 == 0) {
probe.start();
} else {
probe.start(Integer.toString(count));
}
final boolean deeper = random.nextBoolean();
if (deeper && depth < maxDepth) {
int total = 0;
while (total < activeMillis + passiveMillis) {
final int subActiveMillis = getInt(activeMillis);
final int subPassiveMillis = getInt(passiveMillis);
recurse(depth + 1, subActiveMillis, subPassiveMillis);
total += subActiveMillis + subPassiveMillis;
}
} else {
sleep(getValue(activeMillis));
}
if (depth != 0 && generateError()) {
// Do not stop, on purpose
// In that case, next started probe will be erroneously considered as a sub probe
} else {
probe.stop();
}
sleep(getValue(passiveMillis));
}
public static void start(int delay,
int max,
int activeMillis,
int passiveMillis,
int maxDepth,
boolean randomValues,
boolean randomErrors) {
new Thread() {
@Override
public void run() {
PerfsSupport.sleep(delay);
new PerfsSupport(max, activeMillis, passiveMillis, maxDepth, randomValues, randomErrors);
}
}.start();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy