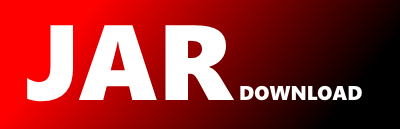
cdc.perfs.ui.fx.MeasureLevelTableCell Maven / Gradle / Ivy
package cdc.perfs.ui.fx;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import cdc.perfs.MeasureLevel;
import cdc.ui.fx.FxUtil;
import javafx.scene.control.ContentDisplay;
import javafx.scene.control.cell.ComboBoxTableCell;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
public class MeasureLevelTableCell extends ComboBoxTableCell {
private static final Logger LOGGER = LogManager.getLogger(MeasureLevelTableCell.class);
private static Image[] images = null;
private final ImageView[] imageViews = new ImageView[MeasureLevel.values().length];
public MeasureLevelTableCell() {
super(MeasureLevel.values());
init();
}
private synchronized void init() {
if (images == null) {
final Image[] tmp = new Image[MeasureLevel.values().length];
for (final MeasureLevel level : MeasureLevel.values()) {
tmp[level.ordinal()] = FxUtil.load("cdc/perfs/images/cdc-perfs-level-" + level.name().toLowerCase() + ".png");
}
images = tmp;
}
for (final MeasureLevel level : MeasureLevel.values()) {
imageViews[level.ordinal()] = new ImageView(images[level.ordinal()]);
}
}
@Override
public void cancelEdit() {
LOGGER.debug("cancelEdit()");
super.cancelEdit();
if (getItem() == null) {
setText(null);
setGraphic(null);
} else {
setGraphic(imageViews[getItem().ordinal()]);
}
}
@Override
public void updateItem(MeasureLevel item,
boolean empty) {
LOGGER.debug("updateItem(" + item + ", " + empty + ")");
super.updateItem(item, empty);
setContentDisplay(ContentDisplay.LEFT);
if (item == null || empty) {
setText(null);
setGraphic(null);
} else {
setGraphic(imageViews[item.ordinal()]);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy