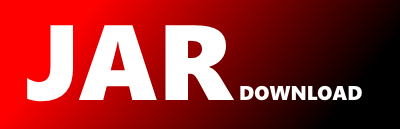
cdc.perfs.ui.swing.EnvironmentPanel Maven / Gradle / Ivy
package cdc.perfs.ui.swing;
import java.awt.BorderLayout;
import java.io.File;
import java.io.IOException;
import javax.swing.JButton;
import javax.swing.JComponent;
import javax.swing.JFileChooser;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JSplitPane;
import javax.swing.JTabbedPane;
import javax.swing.JToolBar;
import javax.swing.filechooser.FileNameExtensionFilter;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import cdc.perfs.Environment;
import cdc.perfs.EnvironmentKind;
import cdc.perfs.io.PerfsIo;
import cdc.perfs.runtime.RuntimeEnvironment;
import cdc.perfs.snapshot.SnapshotEnvironment;
/**
* Panel containing control and display of an environment:
*
* - Display and control of contexts
* - Display and control of sources
* - Display and control of chart (contexts and measures)
*
*
* @author D. Carbonne
*
*/
public final class EnvironmentPanel extends JPanel {
private static final Logger LOGGER = LogManager.getLogger(EnvironmentPanel.class);
private static final long serialVersionUID = 1L;
protected final MainFrame wFrame;
private final JToolBar wToolBar = new JToolBar();
private final JButton wSnaphot;
protected final JButton wSave;
protected final JButton wSaveAs;
private final ContextsTableModel contextsModel;
private final SourcesTableModel sourcesModel;
private final ContextsPanel wContextsPanel;
private final SourcesPanel wSourcesPanel;
private final ControlledChartPanel wControlledChartPanel;
private String filename;
public EnvironmentPanel(MainFrame frame,
Environment environment) {
super();
wFrame = frame;
setLayout(new BorderLayout(0, 0));
add(wToolBar, BorderLayout.PAGE_START);
if (environment.getKind() == EnvironmentKind.RUNTIME) {
wSnaphot = new JButton(MainFrame.SNAPSHOT);
wSnaphot.addActionListener(event -> {
final SnapshotEnvironment snaphot = new SnapshotEnvironment(RuntimeEnvironment.getInstance());
wFrame.addSnaphot(snaphot, null);
});
wSave = null;
wSaveAs = null;
wToolBar.add(wSnaphot);
} else {
wSnaphot = null;
wSave = new JButton("Save");
wSave.addActionListener(event -> save(wSave));
wToolBar.add(wSave);
wSaveAs = new JButton("Save As");
wSaveAs.addActionListener(event -> saveAs(wSaveAs));
wToolBar.add(wSaveAs);
}
contextsModel = new ContextsTableModel(environment);
sourcesModel = new SourcesTableModel(environment);
final JSplitPane wSplitPane = new JSplitPane();
wSplitPane.setOrientation(JSplitPane.HORIZONTAL_SPLIT);
wSplitPane.setOneTouchExpandable(true);
wSplitPane.setDividerLocation(175);
add(wSplitPane);
final JTabbedPane wTabbedPane = new JTabbedPane();
wSplitPane.setLeftComponent(wTabbedPane);
wContextsPanel = new ContextsPanel(contextsModel);
wTabbedPane.addTab("Contexts", wContextsPanel);
wSourcesPanel = new SourcesPanel(sourcesModel);
wTabbedPane.addTab("Sources", wSourcesPanel);
wControlledChartPanel = new ControlledChartPanel(contextsModel);
wSplitPane.setRightComponent(wControlledChartPanel);
}
@Override
public void removeNotify() {
super.removeNotify();
wFrame.removeSnapshot(this);
}
public Environment getEnvironment() {
return wControlledChartPanel.getEnvironment();
}
public ContextsTableModel getContextsTableModel() {
return contextsModel;
}
public ContextsPanel getContextsPanel() {
return wContextsPanel;
}
public SourcesTableModel getSourcesTableModel() {
return sourcesModel;
}
public SourcesPanel getSourcesPanel() {
return wSourcesPanel;
}
public ControlledChartPanel getControlledChartPanel() {
return wControlledChartPanel;
}
public String getFilename() {
return filename;
}
private void setFilename(String filename) {
this.filename = filename;
wFrame.processFilenameChange(this);
}
protected void save(JComponent parent) {
if (getFilename() == null) {
saveAs(parent);
} else {
saveAs(parent, getFilename(), false);
}
}
protected void saveAs(JComponent parent) {
final JFileChooser wChooser = new JFileChooser();
final FileNameExtensionFilter filter =
new FileNameExtensionFilter("Snapshot binary or xml files ", "dat", "xml");
wChooser.setFileFilter(filter);
final int result = wChooser.showOpenDialog(wSave);
if (result == JFileChooser.APPROVE_OPTION) {
saveAs(wChooser, wChooser.getSelectedFile().getPath(), true);
}
}
private void saveAs(JComponent parent,
String filename,
boolean check) {
final String path = PerfsIo.fixFilename(filename);
if (check) {
final File file = new File(path);
if (file.exists()) {
final int result =
JOptionPane.showConfirmDialog(parent,
"File '" + path + "' already exists.\nOverwrite it?",
"File exists",
JOptionPane.WARNING_MESSAGE,
JOptionPane.OK_CANCEL_OPTION);
if (result == JOptionPane.CANCEL_OPTION) {
return;
}
}
}
try {
PerfsIo.save(getEnvironment(), path);
setFilename(path);
} catch (final IOException e) {
LOGGER.catching(e);
JOptionPane.showMessageDialog(parent,
"Failed to save '" + path + "'\n" + e.getMessage(),
null,
JOptionPane.ERROR_MESSAGE);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy