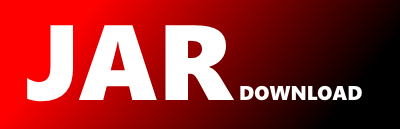
cdc.perfs.ui.swing.SourcesTableModel Maven / Gradle / Ivy
package cdc.perfs.ui.swing;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.concurrent.CopyOnWriteArrayList;
import javax.swing.table.AbstractTableModel;
import cdc.perfs.api.MeasureLevel;
import cdc.perfs.api.Source;
import cdc.perfs.core.Context;
import cdc.perfs.core.Environment;
import cdc.perfs.core.EnvironmentKind;
import cdc.perfs.core.EnvironmentListener;
import cdc.perfs.core.Measure;
import cdc.perfs.ui.SourceDisplay;
/**
* Implementation of a table model for sources used by an environment.
*
* Columns:
*
* - Source.
*
- Max level of generated measures for the source.
*
- Display of the source.
*
*
* @author Damien Carbonne
*
*/
final class SourcesTableModel extends AbstractTableModel {
private static final long serialVersionUID = 1L;
private final transient Environment environment;
private static final String[] COLUMN_NAMES = { "Source", "Max Level", "Display", "Measures" };
private static final int COLUMN_SOURCE = 0;
private static final int COLUMN_MAX_LEVEL = 1;
private static final int COLUMN_DISPLAY = 2;
private static final int COLUMN_MEASURES = 3;
protected final transient List sources = new CopyOnWriteArrayList<>();
protected final transient Map sourcesToRecords = new HashMap<>();
private final transient EnvironmentListener listener = new EnvironmentListener() {
@Override
public void processContextCreated(Context context) {
// Ignore
}
@Override
public void processContextChanged(Context context) {
// Ignore
}
@Override
public void processSourceCreated(Source source) {
createRecord(source);
}
@Override
public void processSourceChanged(Source source) {
final int row = getRowIndex(source);
if (row >= 0) {
fireTableRowsUpdated(row, row);
}
}
@Override
public void processMeasureCreated(Context context,
Measure measure) {
// Ignore
}
@Override
public void processMeasureChanged(Measure measure) {
// Ignore
}
};
public SourcesTableModel(Environment environment) {
this.environment = environment;
for (final Source source : environment.getSources()) {
createRecord(source);
}
environment.addListener(listener);
}
private static final class Record {
private final Source source;
private SourceDisplay display = SourceDisplay.NORMAL;
public Record(Source source) {
this.source = source;
}
public Source getSource() {
return source;
}
public SourceDisplay getDisplay() {
return display;
}
public void setDisplay(SourceDisplay display) {
this.display = display;
}
}
protected synchronized void createRecord(Source source) {
final Record record = new Record(source);
sources.add(source);
sourcesToRecords.put(source, record);
final int index = getRowIndex(source);
fireTableRowsInserted(index, index);
}
private Record getRecord(Source source) {
return sourcesToRecords.get(source);
}
private Record getRecord(int rowIndex) {
return sourcesToRecords.get(sources.get(rowIndex));
}
protected int getRowIndex(Source source) {
return sources.indexOf(source);
}
public Environment getEnvironment() {
return environment;
}
public List getSources() {
return sources;
}
public SourceDisplay getDisplay(Source source) {
final Record record = getRecord(source);
return record.getDisplay();
}
@Override
public int getRowCount() {
return sources.size();
}
@Override
public int getColumnCount() {
return COLUMN_NAMES.length;
}
@Override
public String getColumnName(int column) {
if (column >= 0 && column < getColumnCount()) {
return COLUMN_NAMES[column];
} else {
return null;
}
}
@Override
public Class> getColumnClass(int columnIndex) {
switch (columnIndex) {
case COLUMN_SOURCE:
return Source.class;
case COLUMN_MAX_LEVEL:
return MeasureLevel.class;
case COLUMN_DISPLAY:
return SourceDisplay.class;
case COLUMN_MEASURES:
return Integer.class;
default:
return null;
}
}
@Override
public boolean isCellEditable(int rowIndex,
int columnIndex) {
return getEnvironment().getKind() == EnvironmentKind.RUNTIME
&& columnIndex == COLUMN_MAX_LEVEL || columnIndex == COLUMN_DISPLAY;
}
@Override
public Object getValueAt(int rowIndex,
int columnIndex) {
final Record record = getRecord(rowIndex);
switch (columnIndex) {
case COLUMN_SOURCE:
return record.getSource();
case COLUMN_MAX_LEVEL:
return record.getSource().getMaxLevel();
case COLUMN_DISPLAY:
return record.getDisplay();
case COLUMN_MEASURES:
return record.getSource().getMeasuresCount();
default:
return null;
}
}
@Override
public void setValueAt(Object value,
int rowIndex,
int columnIndex) {
if (columnIndex == COLUMN_MAX_LEVEL) {
final Record record = getRecord(rowIndex);
record.getSource().setMaxLevel((MeasureLevel) value);
fireTableRowsUpdated(rowIndex, rowIndex);
} else if (columnIndex == COLUMN_DISPLAY) {
final Record record = getRecord(rowIndex);
record.setDisplay((SourceDisplay) value);
fireTableRowsUpdated(rowIndex, rowIndex);
}
}
public void setDisplay(Source source,
SourceDisplay display) {
setValueAt(display, getRowIndex(source), COLUMN_DISPLAY);
}
public void setDisplay(SourceDisplay display) {
for (int rowIndex = 0; rowIndex < getRowCount(); rowIndex++) {
setValueAt(display, rowIndex, COLUMN_DISPLAY);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy