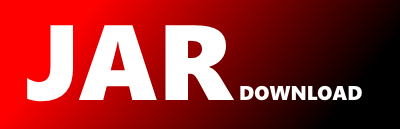
cdc.perfs.ui.swing.PerfsViewer Maven / Gradle / Ivy
package cdc.perfs.ui.swing;
import java.io.IOException;
import java.net.URL;
import java.util.ArrayList;
import java.util.List;
import javax.swing.JOptionPane;
import javax.swing.SwingUtilities;
import org.apache.commons.cli.CommandLine;
import org.apache.commons.cli.Option;
import org.apache.commons.cli.Options;
import org.apache.commons.cli.ParseException;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import cdc.perfs.core.io.PerfsIo;
import cdc.perfs.core.snapshot.SnapshotEnvironment;
import cdc.perfs.ui.Rendering;
import cdc.util.cli.AbstractMainSupport;
import cdc.util.cli.FeatureMask;
import cdc.util.cli.MainResult;
import cdc.util.cli.OptionEnum;
import cdc.util.files.Resources;
/**
* Performances viewer.
*
* This is a standalone tool that can be used to display previously recorded performances.
*
* @author Damien Carbonne
*
*/
public final class PerfsViewer {
private static final Logger LOGGER = LogManager.getLogger(PerfsViewer.class);
private final MainArgs margs;
public static class MainArgs {
public final List inputs = new ArrayList<>();
protected final FeatureMask features = new FeatureMask<>();
public void setEnabled(Feature feature,
boolean enabled) {
features.setEnabled(feature, enabled);
}
public boolean isEnabled(Feature feature) {
return features.isEnabled(feature);
}
/**
* Enumeration of possible boolean options.
*/
public enum Feature implements OptionEnum {
FASTEST("fastest", "If set, use fastest drawing algorithm (default)."),
BEST("best", "If set, use best drawing algorithm."),
SHOW_STATS("show-stats", "If set, show statictics."),
NO_SHOW_STATS("no-show-stats", "If set, do not show statictics (default)."),
DRAW_BORDERS("draw-borders", "If set, draw borders of measures (default)."),
NO_DRAW_BORDERS("no-draw-borders", "If set, hide borders of measures.");
private final String name;
private final String description;
private Feature(String name,
String description) {
this.name = name;
this.description = description;
}
@Override
public final String getName() {
return name;
}
@Override
public final String getDescription() {
return description;
}
}
}
private PerfsViewer(MainArgs margs) {
this.margs = margs;
}
private void execute() {
SwingUtilities.invokeLater(() -> {
final MainFrame frame = new MainFrame(false);
// Analyze features
final Rendering rendering = margs.isEnabled(MainArgs.Feature.BEST) ? Rendering.BEST : Rendering.FASTEST;
frame.setRendering(rendering);
final boolean showStats = margs.isEnabled(MainArgs.Feature.SHOW_STATS);
frame.setShowStats(showStats);
final boolean drawBorders = !margs.isEnabled(MainArgs.Feature.NO_DRAW_BORDERS);
frame.setDrawBorders(drawBorders);
frame.setVisible(true);
for (final URL url : margs.inputs) {
try {
final SnapshotEnvironment environment = PerfsIo.load(url);
frame.addSnaphot(environment, url.getPath());
} catch (final IOException e) {
LOGGER.error("Failed to load " + url, e);
JOptionPane.showMessageDialog(frame,
"Failed to open '" + url + "'\n" + e.getMessage(),
"Error",
JOptionPane.ERROR_MESSAGE);
}
}
});
}
public static void execute(MainArgs margs) {
final PerfsViewer instance = new PerfsViewer(margs);
instance.execute();
}
public static MainResult exec(String... args) {
final MainSupport support = new MainSupport();
support.main(args);
return support.getResult();
}
public static void main(String... args) {
// Do not exit
exec(args);
}
private static class MainSupport extends AbstractMainSupport {
public MainSupport() {
super(PerfsViewer.class, LOGGER);
}
@Override
protected String getVersion() {
return cdc.perfs.api.Config.VERSION;
}
@Override
protected void addSpecificOptions(Options options) {
options.addOption(Option.builder()
.longOpt(INPUT)
.desc("Optional name(s) of the XML/binary input(s) to open.")
.hasArgs()
.build());
addNoArgOptions(options, MainArgs.Feature.class);
createGroup(options, MainArgs.Feature.FASTEST, MainArgs.Feature.BEST);
createGroup(options, MainArgs.Feature.SHOW_STATS, MainArgs.Feature.NO_SHOW_STATS);
createGroup(options, MainArgs.Feature.DRAW_BORDERS, MainArgs.Feature.NO_DRAW_BORDERS);
}
@Override
protected MainArgs analyze(CommandLine cl) throws ParseException {
final MainArgs margs = new MainArgs();
if (cl.hasOption(INPUT)) {
for (final String input : cl.getOptionValues(INPUT)) {
final URL url = Resources.getResource(input);
if (url == null) {
throw new ParseException("Invalid url: " + input);
}
margs.inputs.add(url);
}
setMask(cl, MainArgs.Feature.class, margs.features::setEnabled);
}
return margs;
}
@Override
protected Void execute(MainArgs margs) throws Exception {
PerfsViewer.execute(margs);
return null;
}
}
}