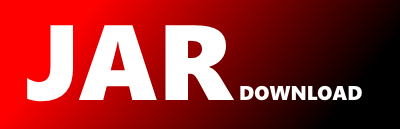
cdc.ui.swing.GridBagConstraintsBuilder Maven / Gradle / Ivy
package cdc.ui.swing;
import java.awt.GridBagConstraints;
import java.awt.Insets;
public class GridBagConstraintsBuilder {
private int gridx = GridBagConstraints.RELATIVE;
private int gridy = GridBagConstraints.RELATIVE;
private int gridwidth = 1;
private int gridheight = 1;
private double weightx = 0.0;
private double weighty = 0.0;
private int anchor = GridBagConstraints.CENTER;
private int fill = GridBagConstraints.NONE;
private Insets insets = new Insets(0, 0, 0, 0);
private int ipadx = 0;
private int ipady = 0;
public static GridBagConstraintsBuilder builder() {
return new GridBagConstraintsBuilder();
}
public GridBagConstraintsBuilder clear() {
gridx = GridBagConstraints.RELATIVE;
gridy = GridBagConstraints.RELATIVE;
gridwidth = 1;
gridheight = 1;
weightx = 0.0;
weighty = 0.0;
anchor = GridBagConstraints.CENTER;
fill = GridBagConstraints.NONE;
insets.set(0, 0, 0, 0);
ipadx = 0;
ipady = 0;
return this;
}
public GridBagConstraintsBuilder gridx(int value) {
this.gridx = value;
return this;
}
public GridBagConstraintsBuilder gridy(int value) {
this.gridy = value;
return this;
}
public GridBagConstraintsBuilder gridwidth(int value) {
this.gridwidth = value;
return this;
}
public GridBagConstraintsBuilder gridheight(int value) {
this.gridheight = value;
return this;
}
public GridBagConstraintsBuilder weightx(double value) {
this.weightx = value;
return this;
}
public GridBagConstraintsBuilder weighty(double value) {
this.weighty = value;
return this;
}
public GridBagConstraintsBuilder anchor(int value) {
this.anchor = value;
return this;
}
public GridBagConstraintsBuilder fill(int value) {
this.fill = value;
return this;
}
public GridBagConstraintsBuilder insets(Insets value) {
this.insets = value;
return this;
}
public GridBagConstraintsBuilder insets(int top,
int left,
int bottom,
int right) {
this.insets.set(top, left, bottom, right);
return this;
}
public GridBagConstraintsBuilder ipadx(int value) {
this.ipadx = value;
return this;
}
public GridBagConstraintsBuilder ipady(int value) {
this.ipady = value;
return this;
}
public GridBagConstraints build() {
return new GridBagConstraints(gridx,
gridy,
gridwidth,
gridheight,
weightx,
weighty,
anchor,
fill,
(Insets) insets.clone(),
ipadx,
ipady);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy