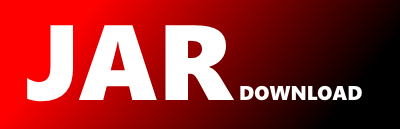
cdc.ui.swing.cells.CellEditorHelper Maven / Gradle / Ivy
package cdc.ui.swing.cells;
import java.awt.Component;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.ItemEvent;
import java.awt.event.ItemListener;
import java.awt.event.MouseEvent;
import java.util.EventObject;
import javax.swing.AbstractCellEditor;
import javax.swing.JComponent;
import javax.swing.JTable;
import javax.swing.JTree;
import javax.swing.table.TableCellEditor;
import javax.swing.tree.TreeCellEditor;
public class CellEditorHelper extends AbstractCellEditor
implements TableCellEditor, TreeCellEditor {
private static final long serialVersionUID = 1L;
protected W wComponent;
/**
* The delegate class which handles all methods sent from the
* CellEditor
.
*/
protected EditorDelegate delegate;
/**
* An integer specifying the number of clicks needed to start editing.
* Even if clickCountToStart
is defined as zero, it
* will not initiate until a click occurs.
*/
protected int clickCountToStart = 1;
protected CellEditorHelper() {
}
/**
* Returns a reference to the editor component.
*
* @return the editor Component
*/
public final W getComponent() {
return wComponent;
}
/**
* Specifies the number of clicks needed to start editing.
*
* @param count an int specifying the number of clicks needed to start
* editing
* @see #getClickCountToStart
*/
public final void setClickCountToStart(int count) {
clickCountToStart = count;
}
/**
* Returns the number of clicks needed to start editing.
*
* @return the number of clicks needed to start editing
*/
public final int getClickCountToStart() {
return clickCountToStart;
}
/**
* Forwards the message from the CellEditor
to
* the delegate
.
*
* @see EditorDelegate#getCellEditorValue
*/
@Override
public Object getCellEditorValue() {
return delegate.getCellEditorValue();
}
/**
* Forwards the message from the CellEditor
to
* the delegate
.
*
* @see EditorDelegate#isCellEditable(EventObject)
*/
@Override
public final boolean isCellEditable(EventObject event) {
return delegate.isCellEditable(event);
}
/**
* Forwards the message from the CellEditor
to
* the delegate
.
*
* @see EditorDelegate#shouldSelectCell(EventObject)
*/
@Override
public final boolean shouldSelectCell(EventObject event) {
return delegate.shouldSelectCell(event);
}
/**
* Forwards the message from the CellEditor
to
* the delegate
.
*
* @see EditorDelegate#stopCellEditing
*/
@Override
public final boolean stopCellEditing() {
return delegate.stopCellEditing();
}
/**
* Forwards the message from the CellEditor
to
* the delegate
.
*
* @see EditorDelegate#cancelCellEditing
*/
@Override
public final void cancelCellEditing() {
delegate.cancelCellEditing();
}
@Override
public Component getTreeCellEditorComponent(JTree tree,
Object value,
boolean isSelected,
boolean expanded,
boolean leaf,
int row) {
delegate.setValue(value);
return wComponent;
}
@Override
public Component getTableCellEditorComponent(JTable table,
Object value,
boolean isSelected,
int row,
int column) {
delegate.setValue(value);
return wComponent;
}
protected abstract class EditorDelegate implements ActionListener, ItemListener {
/**
* @return The value of this cell.
*/
public abstract Object getCellEditorValue();
/**
* Sets the value of this cell.
*
* @param value The new value of this cell.
*/
public abstract void setValue(Object value);
/**
* Returns true if event
is not a
* MouseEvent
. Otherwise, it returns true
* if the necessary number of clicks have occurred, and
* returns false otherwise.
*
* @param event The event.
* @return true if cell is ready for editing, false otherwise
* @see #setClickCountToStart
* @see #shouldSelectCell
*/
public boolean isCellEditable(EventObject event) {
if (event instanceof MouseEvent) {
return ((MouseEvent) event).getClickCount() >= clickCountToStart;
}
return true;
}
/**
* Returns true to indicate that the editing cell may
* be selected.
*
* @param event The event.
* @return true.
* @see #isCellEditable
*/
public boolean shouldSelectCell(EventObject event) {
return true;
}
/**
* Returns true to indicate that editing has begun.
*
* @param event The event.
* @return true.
*/
public boolean startCellEditing(EventObject event) {
return true;
}
/**
* Stops editing and returns true to indicate that editing has stopped.
*
* This method calls {@link AbstractCellEditor#fireEditingStopped()}.
*
* @return {@code true}
*/
public boolean stopCellEditing() {
fireEditingStopped();
return true;
}
/**
* Cancels editing.
*
* This method calls {@link AbstractCellEditor#fireEditingCanceled}.
*/
public void cancelCellEditing() {
fireEditingCanceled();
}
/**
* When an action is performed, editing is ended.
*
* @param event the action event
* @see #stopCellEditing
*/
@Override
public void actionPerformed(ActionEvent event) {
CellEditorHelper.this.stopCellEditing();
}
/**
* When an item's state changes, editing is ended.
*
* @param event the action event
* @see #stopCellEditing
*/
@Override
public void itemStateChanged(ItemEvent event) {
CellEditorHelper.this.stopCellEditing();
}
}
}