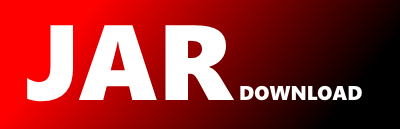
cdc.util.pstrings.PackedString32 Maven / Gradle / Ivy
package cdc.util.pstrings;
import java.nio.ByteBuffer;
final class PackedString32 extends PackedString {
private final int f1;
private final int f2;
private final int f3;
private final int f4;
private final int f5;
private final int f6;
private final int f7;
private final int f8;
public PackedString32(final byte[] bytes) {
f1 = get(bytes, 3) | get(bytes, 2) << 8 | get(bytes, 1) << 16 | get(bytes, 0) << 24;
f2 = get(bytes, 7) | get(bytes, 6) << 8 | get(bytes, 5) << 16 | get(bytes, 4) << 24;
f3 = get(bytes, 11) | get(bytes, 10) << 8 | get(bytes, 9) << 16 | get(bytes, 8) << 24;
f4 = get(bytes, 15) | get(bytes, 14) << 8 | get(bytes, 13) << 16 | get(bytes, 12) << 24;
f5 = get(bytes, 19) | get(bytes, 18) << 8 | get(bytes, 17) << 16 | get(bytes, 16) << 24;
f6 = get(bytes, 23) | get(bytes, 22) << 8 | get(bytes, 21) << 16 | get(bytes, 20) << 24;
f7 = get(bytes, 27) | get(bytes, 26) << 8 | get(bytes, 25) << 16 | get(bytes, 24) << 24;
f8 = get(bytes, 31) | get(bytes, 30) << 8 | get(bytes, 29) << 16 | get(bytes, 28) << 24;
}
@Override
protected byte[] toBytes() {
final ByteBuffer buffer = ByteBuffer.allocate(64);
buffer.putInt(f1);
buffer.putInt(f2);
buffer.putInt(f3);
buffer.putInt(f4);
buffer.putInt(f5);
buffer.putInt(f6);
buffer.putInt(f7);
buffer.putInt(f8);
return buffer.array();
}
@Override
public boolean equals(Object other) {
if (this == other) {
return true;
}
if (other == null || getClass() != other.getClass()) {
return false;
}
final PackedString32 o = (PackedString32) other;
return f1 == o.f1 && f2 == o.f2 && f3 == o.f3 && f4 == o.f4 && f5 == o.f5 && f6 == o.f6 && f7 == o.f7
&& f8 == o.f8;
}
@Override
public int hashCode() {
int result = f1;
result = 31 * result + f2;
result = 31 * result + f3;
result = 31 * result + f4;
result = 31 * result + f5;
result = 31 * result + f6;
result = 31 * result + f7;
result = 31 * result + f8;
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy