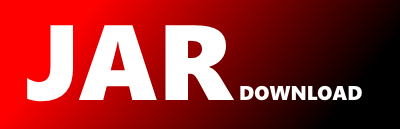
cdc.util.pstrings.PackedString64 Maven / Gradle / Ivy
package cdc.util.pstrings;
import java.nio.ByteBuffer;
final class PackedString64 extends PackedString {
private final int f1;
private final int f2;
private final int f3;
private final int f4;
private final int f5;
private final int f6;
private final int f7;
private final int f8;
private final int f9;
private final int f10;
private final int f11;
private final int f12;
private final int f13;
private final int f14;
private final int f15;
private final int f16;
public PackedString64(final byte[] bytes) {
f1 = get(bytes, 3) | get(bytes, 2) << 8 | get(bytes, 1) << 16 | get(bytes, 0) << 24;
f2 = get(bytes, 7) | get(bytes, 6) << 8 | get(bytes, 5) << 16 | get(bytes, 4) << 24;
f3 = get(bytes, 11) | get(bytes, 10) << 8 | get(bytes, 9) << 16 | get(bytes, 8) << 24;
f4 = get(bytes, 15) | get(bytes, 14) << 8 | get(bytes, 13) << 16 | get(bytes, 12) << 24;
f5 = get(bytes, 19) | get(bytes, 18) << 8 | get(bytes, 17) << 16 | get(bytes, 16) << 24;
f6 = get(bytes, 23) | get(bytes, 22) << 8 | get(bytes, 21) << 16 | get(bytes, 20) << 24;
f7 = get(bytes, 27) | get(bytes, 26) << 8 | get(bytes, 25) << 16 | get(bytes, 24) << 24;
f8 = get(bytes, 31) | get(bytes, 30) << 8 | get(bytes, 29) << 16 | get(bytes, 28) << 24;
f9 = get(bytes, 35) | get(bytes, 34) << 8 | get(bytes, 33) << 16 | get(bytes, 32) << 24;
f10 = get(bytes, 39) | get(bytes, 38) << 8 | get(bytes, 37) << 16 | get(bytes, 36) << 24;
f11 = get(bytes, 43) | get(bytes, 42) << 8 | get(bytes, 41) << 16 | get(bytes, 40) << 24;
f12 = get(bytes, 47) | get(bytes, 46) << 8 | get(bytes, 45) << 16 | get(bytes, 44) << 24;
f13 = get(bytes, 51) | get(bytes, 50) << 8 | get(bytes, 49) << 16 | get(bytes, 48) << 24;
f14 = get(bytes, 55) | get(bytes, 54) << 8 | get(bytes, 53) << 16 | get(bytes, 52) << 24;
f15 = get(bytes, 59) | get(bytes, 58) << 8 | get(bytes, 57) << 16 | get(bytes, 56) << 24;
f16 = get(bytes, 63) | get(bytes, 62) << 8 | get(bytes, 61) << 16 | get(bytes, 60) << 24;
}
@Override
protected byte[] toBytes() {
final ByteBuffer buffer = ByteBuffer.allocate(64);
buffer.putInt(f1);
buffer.putInt(f2);
buffer.putInt(f3);
buffer.putInt(f4);
buffer.putInt(f5);
buffer.putInt(f6);
buffer.putInt(f7);
buffer.putInt(f8);
buffer.putInt(f9);
buffer.putInt(f10);
buffer.putInt(f11);
buffer.putInt(f12);
buffer.putInt(f13);
buffer.putInt(f14);
buffer.putInt(f15);
buffer.putInt(f16);
return buffer.array();
}
@Override
public boolean equals(Object other) {
if (this == other) {
return true;
}
if (other == null || getClass() != other.getClass()) {
return false;
}
final PackedString64 o = (PackedString64) other;
return f1 == o.f1 && f2 == o.f2 && f3 == o.f3 && f4 == o.f4 && f5 == o.f5 && f6 == o.f6 && f7 == o.f7
&& f8 == o.f8 && f9 == o.f9 && f10 == o.f10 && f11 == o.f11 && f12 == o.f12 && f13 == o.f13
&& f14 == o.f14 && f15 == o.f15 && f16 == o.f16;
}
@Override
public int hashCode() {
int result = f1;
result = 31 * result + f2;
result = 31 * result + f3;
result = 31 * result + f4;
result = 31 * result + f5;
result = 31 * result + f6;
result = 31 * result + f7;
result = 31 * result + f8;
result = 31 * result + f9;
result = 31 * result + f10;
result = 31 * result + f11;
result = 31 * result + f12;
result = 31 * result + f13;
result = 31 * result + f14;
result = 31 * result + f15;
result = 31 * result + f16;
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy