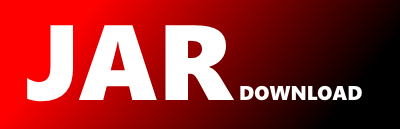
cdc.util.rdb.RdbDatabaseXmlIo Maven / Gradle / Ivy
package cdc.util.rdb;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.io.PrintStream;
import java.net.URL;
import cdc.util.data.Document;
import cdc.util.data.Element;
import cdc.util.data.xml.XmlDataReader;
import cdc.util.function.Iterables;
import cdc.util.xml.XmlWriter;
import cdc.util.xml.XmlWriter.Feature;
public final class RdbDatabaseXmlIo {
private static final String ATTRIBUTE = "attribute";
private static final String AUTO_INCREMENT = "auto-increment";
private static final String BOOLEAN_PROPERTIES = "boolean-properties";
private static final String CASE_SENSITIVE = "case-sensitive";
private static final String CATALOG = "catalog";
private static final String CATALOGS = "catalogs";
private static final String CLASS_NAME = "class-name";
private static final String COLUMN = "column";
private static final String COLUMNS = "columns";
private static final String COMMENTS = "comments";
private static final String CREATE_PARAMS = "create-params";
private static final String DATABASE = "database";
private static final String DATA_TYPE = "data-type";
private static final String DATA_TYPES = "data-types";
private static final String DEFAULT_VALUE = "default-value";
private static final String DIGITS = "digits";
private static final String ENUM_PROPERTIES = "enum-properties";
private static final String FIXED_PRECISION_SCALE = "fixed-precision-scale";
private static final String FOREIGN_KEY = "foreign-key";
private static final String FOREIGN_KEYS = "foreign-keys";
private static final String FUNCTION = "function";
private static final String FUNCTIONS = "functions";
private static final String GENERATED = "generated";
private static final String INDEX = "index";
private static final String INDICES = "indices";
private static final String INTEGER_PROPERTIES = "integer-properties";
private static final String LITERAL_PREFIX = "literal-prefix";
private static final String LITERAL_SUFFIX = "literal-suffix";
private static final String LOCALIZED_NAME = "localized-name";
private static final String LONG_PROPERTIES = "long-properties";
private static final String MAX_PRECISION = "max-precision";
private static final String MAX_SCALE = "max-scale";
private static final String MIN_SCALE = "min-scale";
private static final String NAME = "name";
private static final String NULLABLE = "nullable";
private static final String ORDINAL = "ordinal";
private static final String PRIMARY_KEY = "primary-key";
private static final String PROCEDURE = "procedure";
private static final String PROCEDURES = "procedures";
private static final String PROPERTY = "property";
private static final String RADIX = "radix";
private static final String REF_CATALOG = "ref-catalog";
private static final String REF_COLUMN = "ref-coluln";
private static final String REF_SCHEMA = "ref-schema";
private static final String REF_TABLE = "ref-table";
private static final String RESULT_TYPE = "result-type";
private static final String SCHEMA = "schema";
private static final String SIZE = "size";
private static final String SPECIFIC_NAME = "specific-name";
private static final String STRING_PROPERTIES = "string-properties";
private static final String TABLE = "table";
private static final String TABLES = "tables";
private static final String TABLE_TYPE = "table-type";
private static final String TABLE_TYPES = "table-types";
private static final String TYPE = "type";
private static final String UNSIGNED = "unsigned";
private static final String USER_DATA_TYPE = "user-data-type";
private static final String USER_DATA_TYPES = "user-data-types";
private static final String VALUE = "value";
private RdbDatabaseXmlIo() {
}
public static void print(RdbDatabase database,
XmlWriter writer) throws IOException {
final Printer printer = new Printer(writer);
printer.print(database);
}
public static void print(RdbDatabase database,
PrintStream out) throws IOException {
print(database, new XmlWriter(out));
}
public static void print(RdbDatabase database,
File file) throws IOException {
print(database, new XmlWriter(file));
}
private static class Printer {
private final XmlWriter writer;
Printer(XmlWriter writer) {
this.writer = writer;
this.writer.setEnabled(Feature.PRETTY_PRINT, true);
this.writer.setTabSize(2);
}
void print(RdbDatabase database) throws IOException {
writer.beginDocument();
writer.beginElement(DATABASE);
writer.addAttribute(NAME, database.getName());
printComments(database);
printProperties(database);
printDataTypes(database);
printTableTypes(database);
printCatalogs(database);
writer.endElement();
writer.endDocument();
writer.close();
}
private void printComments(RdbElement element) throws IOException {
if (element.getComments() != null && !element.getComments().isEmpty()) {
writer.beginElement(COMMENTS);
writer.addElementContent(element.getComments());
writer.endElement();
}
}
private void printProperty(Enum> name,
Object value) throws IOException {
writer.beginElement(PROPERTY);
writer.addAttribute(NAME, name.name());
writer.addAttribute(VALUE, value == null ? "" : value.toString());
writer.endElement();
}
private void printProperties(RdbDatabase database) throws IOException {
writer.beginElement(BOOLEAN_PROPERTIES);
for (final RdbDatabase.BooleanProperty property : RdbDatabase.BooleanProperty.values()) {
if (database.isDefined(property)) {
final Boolean value = database.getProperty(property);
printProperty(property, value);
}
}
writer.endElement();
writer.beginElement(ENUM_PROPERTIES);
for (final RdbDatabase.EnumProperty property : RdbDatabase.EnumProperty.values()) {
if (database.isDefined(property)) {
final Enum> value = database.getProperty(property);
printProperty(property, value);
}
}
writer.endElement();
writer.beginElement(INTEGER_PROPERTIES);
for (final RdbDatabase.IntegerProperty property : RdbDatabase.IntegerProperty.values()) {
if (database.isDefined(property)) {
final Integer value = database.getProperty(property);
printProperty(property, value);
}
}
writer.endElement();
writer.beginElement(LONG_PROPERTIES);
for (final RdbDatabase.LongProperty property : RdbDatabase.LongProperty.values()) {
if (database.isDefined(property)) {
final Long value = database.getProperty(property);
printProperty(property, value);
}
}
writer.endElement();
writer.beginElement(STRING_PROPERTIES);
for (final RdbDatabase.StringProperty property : RdbDatabase.StringProperty.values()) {
if (database.isDefined(property)) {
final String value = database.getProperty(property);
printProperty(property, value);
}
}
writer.endElement();
}
private void printDataTypes(RdbDatabase database) throws IOException {
writer.beginElement(DATA_TYPES);
for (final RdbDataType type : database.getChildren(RdbDataType.class)) {
writer.beginElement(DATA_TYPE);
writer.addAttribute(NAME, type.getName());
writer.addAttribute(LITERAL_PREFIX, type.getLiteralPrefix());
writer.addAttribute(LITERAL_SUFFIX, type.getLiteralSuffix());
writer.addAttribute(CREATE_PARAMS, type.getCreateParams());
writer.addAttribute(MAX_PRECISION, type.getMaxPrecision());
writer.addAttribute(MIN_SCALE, type.getMinScale());
writer.addAttribute(MAX_SCALE, type.getMaxScale());
writer.addAttribute(NULLABLE, type.getNullable().name());
writer.addAttribute(TYPE, type.getType().name());
writer.addAttribute(RADIX, type.getRadix());
writer.addAttribute(AUTO_INCREMENT, type.isAutoIncrement());
writer.addAttribute(LOCALIZED_NAME, type.getLocalizedName());
writer.addAttribute(CASE_SENSITIVE, type.isCaseSensitive());
writer.addAttribute(FIXED_PRECISION_SCALE, type.isFixedPrecisionScale());
writer.addAttribute(UNSIGNED, type.isUnsigned());
printComments(type);
// TODO
writer.endElement();
}
writer.endElement();
}
private void printTableTypes(RdbDatabase database) throws IOException {
writer.beginElement(TABLE_TYPES);
for (final RdbTableType x : database.getChildren(RdbTableType.class)) {
writer.beginElement(TABLE_TYPE);
writer.addAttribute(NAME, x.getName());
printComments(x);
writer.endElement();
}
writer.endElement();
}
private void printCatalogs(RdbDatabase database) throws IOException {
writer.beginElement(CATALOGS);
for (final RdbCatalog catalog : database.getCatalogs()) {
printCatalog(catalog);
}
writer.endElement();
}
private void printCatalog(RdbCatalog catalog) throws IOException {
writer.beginElement(CATALOG);
writer.addAttribute(NAME, catalog.getName());
printComments(catalog);
for (final RdbSchema schema : catalog.getSchemas()) {
printSchema(schema);
}
writer.endElement();
}
private void printSchema(RdbSchema schema) throws IOException {
writer.beginElement(SCHEMA);
writer.addAttribute(NAME, schema.getName());
printComments(schema);
printUserDataTypes(schema);
printFunctions(schema);
printProcedures(schema);
printTables(schema);
writer.endElement();
}
private void printUserDataTypes(RdbSchema schema) throws IOException {
if (!Iterables.isEmpty(schema.getUserDataTypes())) {
writer.beginElement(USER_DATA_TYPES);
for (final RdbUserDataType type : schema.getUserDataTypes()) {
printUserDataType(type);
}
writer.endElement();
}
}
private void printUserDataType(RdbUserDataType type) throws IOException {
writer.beginElement(USER_DATA_TYPE);
writer.addAttribute(NAME, type.getName());
writer.addAttribute(CLASS_NAME, type.getClassName());
writer.addAttribute(TYPE, type.getType().name());
// TODO
printComments(type);
for (final RdbAttribute attribute : type.getAttributes()) {
printAttribute(attribute);
}
writer.endElement();
}
private void printAttribute(RdbAttribute attribute) throws IOException {
writer.beginElement(ATTRIBUTE);
writer.addAttribute(NAME, attribute.getName());
// TODO
writer.endElement();
}
private void printFunctions(RdbSchema schema) throws IOException {
if (!Iterables.isEmpty(schema.getFunctions())) {
writer.beginElement(FUNCTIONS);
for (final RdbFunction function : schema.getFunctions()) {
printFunction(function);
}
writer.endElement();
}
}
private void printFunction(RdbFunction function) throws IOException {
writer.beginElement(FUNCTION);
writer.addAttribute(NAME, function.getName());
writer.addAttribute(SPECIFIC_NAME, function.getSpecificName());
writer.addAttribute(RESULT_TYPE, function.getResultType());
printComments(function);
for (final RdbFunctionColumn column : function.getColumns()) {
printFunctionColumn(column);
}
writer.endElement();
}
private void printFunctionColumn(RdbFunctionColumn column) throws IOException {
writer.beginElement(COLUMN);
writer.addAttribute(NAME, column.getName());
// TODO
writer.endElement();
}
private void printProcedures(RdbSchema schema) throws IOException {
if (!Iterables.isEmpty(schema.getProcedures())) {
writer.beginElement(PROCEDURES);
for (final RdbProcedure procedure : schema.getProcedures()) {
printProcedure(procedure);
}
writer.endElement();
}
}
private void printProcedure(RdbProcedure procedure) throws IOException {
writer.beginElement(PROCEDURE);
writer.addAttribute(NAME, procedure.getName());
writer.addAttribute(SPECIFIC_NAME, procedure.getSpecificName());
writer.addAttribute(RESULT_TYPE, procedure.getResultType().name());
printComments(procedure);
for (final RdbProcedureColumn column : procedure.getColumns()) {
printProcedureColumn(column);
}
writer.endElement();
}
private void printProcedureColumn(RdbProcedureColumn column) throws IOException {
writer.beginElement(COLUMN);
writer.addAttribute(NAME, column.getName());
// TODO
writer.endElement();
}
private void printTables(RdbSchema schema) throws IOException {
if (!Iterables.isEmpty(schema.getTables())) {
writer.beginElement(TABLES);
for (final RdbTable table : schema.getTables()) {
printTable(table);
}
writer.endElement();
}
}
private void printTable(RdbTable table) throws IOException {
writer.beginElement(TABLE);
writer.addAttribute(NAME, table.getName());
writer.addAttribute(TABLE_TYPE, table.getTableTypeName());
printComments(table);
printTableColumns(table);
if (table.getOptionalPrimaryKey() != null) {
printPrimaryKey(table.getOptionalPrimaryKey());
}
printForeignKeys(table);
printIndices(table);
writer.endElement();
}
private void printTableColumns(RdbTable table) throws IOException {
if (!Iterables.isEmpty(table.getColumns())) {
writer.beginElement(COLUMNS);
for (final RdbTableColumn column : table.getColumns()) {
printTableColumn(column);
}
writer.endElement();
}
}
private void printTableColumn(RdbTableColumn column) throws IOException {
writer.beginElement(COLUMN);
writer.addAttribute(NAME, column.getName());
writer.addAttribute(ORDINAL, column.getOrdinal());
writer.addAttribute(DATA_TYPE, column.getDataType());
writer.addAttribute(TYPE, column.getTypeName());
writer.addAttribute(SIZE, column.getSize());
writer.addAttribute(RADIX, column.getRadix());
writer.addAttribute(DIGITS, column.getDigits());
writer.addAttribute(NULLABLE, column.getNullable());
writer.addAttribute(DEFAULT_VALUE, column.getDefaultValue());
writer.addAttribute(AUTO_INCREMENT, column.getAutoIncrement());
writer.addAttribute(GENERATED, column.getGenerated());
// TODO
printComments(column);
writer.endElement();
}
private void printPrimaryKey(RdbPrimaryKey key) throws IOException {
writer.beginElement(PRIMARY_KEY);
writer.addAttribute(NAME, key.getName());
for (final RdbPrimaryKeyColumn column : key.getColumns()) {
printPrimaryKeyColumn(column);
}
writer.endElement();
}
private void printPrimaryKeyColumn(RdbPrimaryKeyColumn column) throws IOException {
writer.beginElement(COLUMN);
writer.addAttribute(NAME, column.getName());
writer.addAttribute(ORDINAL, column.getOrdinal());
writer.endElement();
}
private void printForeignKeys(RdbTable table) throws IOException {
if (!Iterables.isEmpty(table.getForeignKeys())) {
writer.beginElement(FOREIGN_KEYS);
for (final RdbForeignKey key : table.getForeignKeys()) {
printForeignKey(key);
}
writer.endElement();
}
}
private void printForeignKey(RdbForeignKey key) throws IOException {
writer.beginElement(FOREIGN_KEY);
writer.addAttribute(NAME, key.getName());
writer.addAttribute(REF_CATALOG, key.getRefCatalogName());
writer.addAttribute(REF_SCHEMA, key.getRefSchemaName());
writer.addAttribute(REF_TABLE, key.getRefTableName());
for (final RdbForeignKeyColumn column : key.getColumns()) {
printForeignKeyColumn(column);
}
writer.endElement();
}
private void printForeignKeyColumn(RdbForeignKeyColumn column) throws IOException {
writer.beginElement(COLUMN);
writer.addAttribute(NAME, column.getName());
writer.addAttribute(ORDINAL, column.getOrdinal());
writer.addAttribute(REF_COLUMN, column.getRefColumnName());
writer.endElement();
}
private void printIndices(RdbTable table) throws IOException {
if (!Iterables.isEmpty(table.getIndices())) {
writer.beginElement(INDICES);
for (final RdbIndex index : table.getIndices()) {
printIndex(index);
}
writer.endElement();
}
}
private void printIndex(RdbIndex index) throws IOException {
writer.beginElement(INDEX);
writer.addAttribute(NAME, index.getName());
writer.addAttribute(TYPE, index.getType() == null ? "" : index.getType().name());
for (final RdbIndexColumn column : index.getColumns()) {
printIndexColumn(column);
}
writer.endElement();
}
private void printIndexColumn(RdbIndexColumn column) throws IOException {
writer.beginElement(COLUMN);
writer.addAttribute(NAME, column.getName());
writer.addAttribute(ORDINAL, column.getOrdinal());
writer.endElement();
}
}
public static RdbDatabase read(InputStream is) throws IOException {
final XmlDataReader reader = new XmlDataReader();
final Document doc = reader.read(is);
return Reader.parseRoot(doc.getRootElement());
}
public static RdbDatabase read(String filename) throws IOException {
final XmlDataReader reader = new XmlDataReader();
final Document doc = reader.read(filename);
return Reader.parseRoot(doc.getRootElement());
}
public static RdbDatabase read(URL url) throws IOException {
try (final InputStream is = url.openStream()) {
return read(is);
}
}
private static class Reader {
public static RdbDatabase parseRoot(Element root) {
final String name = root.getAttributeValue(NAME, null);
final RdbDatabase database = new RdbDatabase(name);
for (final Element child : root.getChildren(Element.class)) {
switch (child.getName()) {
case COMMENTS:
readComments(child, database);
break;
case BOOLEAN_PROPERTIES:
case ENUM_PROPERTIES:
case INTEGER_PROPERTIES:
case LONG_PROPERTIES:
case STRING_PROPERTIES:
readProperties(child, database);
break;
case DATA_TYPES:
readDataTypes(child, database);
break;
case TABLE_TYPES:
readTableTypes(child, database);
break;
case CATALOGS:
readCatalogs(child, database);
break;
default:
break;
}
}
readComments(root, database);
readProperties(root, database);
return database;
}
private static void readComments(Element comments,
RdbElement element) {
element.setComments(comments.getText(null));
}
private static void readProperties(Element element,
RdbDatabase database) {
for (final Element child : element.getChildren(Element.class)) {
readProperty(child, database);
}
}
private static void readProperty(Element element,
RdbDatabase database) {
final String name = element.getAttributeValue(NAME, null);
final RdbDatabase.PropertyKind kind = RdbDatabase.getPropertyKind(name);
if (kind != null) {
switch (kind) {
case BOOLEAN:
final RdbDatabase.BooleanProperty bp = RdbDatabase.BooleanProperty.valueOf(name);
final boolean b = element.getAttributeAsBoolean(VALUE, false);
database.setProperty(bp, b);
break;
case ENUM:
final RdbDatabase.EnumProperty ep = RdbDatabase.EnumProperty.valueOf(name);
final Class extends Enum>> enumClass = ep.getEnumClass();
final Enum> e = element.getAttributeAsRawEnum(VALUE, enumClass, null);
database.setProperty(ep, e);
break;
case INTEGER:
final RdbDatabase.IntegerProperty ip = RdbDatabase.IntegerProperty.valueOf(name);
final int i = element.getAttributeAsInt(VALUE, 0);
database.setProperty(ip, i);
break;
case LONG:
final RdbDatabase.LongProperty lp = RdbDatabase.LongProperty.valueOf(name);
final long l = element.getAttributeAsLong(VALUE, 0L);
database.setProperty(lp, l);
break;
case STRING:
final RdbDatabase.StringProperty sp = RdbDatabase.StringProperty.valueOf(name);
final String s = element.getAttributeValue(VALUE, null);
database.setProperty(sp, s);
break;
default:
break;
}
}
}
private static void readDataTypes(Element element,
RdbDatabase database) {
for (final Element child : element.getChildren(Element.class)) {
readDataType(child, database);
}
}
private static void readDataType(Element element,
RdbDatabase database) {
final String name = element.getAttributeValue(NAME, null);
final SqlDataType type = element.getAttributeAsOptionalEnum(TYPE, SqlDataType.class, null);
final RdbDataType tmp = database.createDataType(name, type);
tmp.setLiteralPrefix(element.getAttributeValue(LITERAL_PREFIX, null));
tmp.setLiteralSuffix(element.getAttributeValue(LITERAL_SUFFIX, null));
tmp.setCreateParams(element.getAttributeValue(CREATE_PARAMS, null));
tmp.setMaxPrecison(element.getAttributeAsInt(MAX_PRECISION, 0));
tmp.setMinScale(element.getAttributeAsShort(MIN_SCALE, (short) 0));
tmp.setMaxScale(element.getAttributeAsShort(MAX_SCALE, (short) 0));
tmp.setNullable(element.getAttributeAsOptionalEnum(NULLABLE, YesNoUnknown.class, null));
tmp.setRadix(element.getAttributeAsInt(RADIX, 0));
tmp.setLocalizedName(element.getAttributeValue(LOCALIZED_NAME, null));
tmp.setAutoIncrement(element.getAttributeAsBoolean(AUTO_INCREMENT, false));
tmp.setCaseSensitive(element.getAttributeAsBoolean(CASE_SENSITIVE, false));
tmp.setFixedPrecisionScale(element.getAttributeAsBoolean(FIXED_PRECISION_SCALE, false));
tmp.setUnsigned(element.getAttributeAsBoolean(UNSIGNED, false));
for (final Element child : element.getChildren(Element.class)) {
if (COMMENTS.equals(child.getName())) {
readComments(child, tmp);
}
}
}
private static void readTableTypes(Element element,
RdbDatabase database) {
for (final Element child : element.getChildren(Element.class)) {
final String name = child.getAttributeValue(NAME, null);
database.createTableType(name);
}
}
private static void readCatalogs(Element element,
RdbDatabase database) {
for (final Element child : element.getChildren(Element.class)) {
readCatalog(child, database);
}
}
private static void readCatalog(Element element,
RdbDatabase database) {
final String name = element.getAttributeValue(NAME, null);
final RdbCatalog catalog = database.createCatalog(name);
for (final Element child : element.getChildren(Element.class)) {
switch (child.getName()) {
case COMMENTS:
readComments(child, catalog);
break;
case SCHEMA:
readSchema(child, catalog);
break;
default:
break;
}
}
}
private static void readSchema(Element element,
RdbCatalog catalog) {
final String name = element.getAttributeValue(NAME, null);
final RdbSchema schema = catalog.createSchema(name);
for (final Element child : element.getChildren(Element.class)) {
switch (child.getName()) {
case COMMENTS:
readComments(child, schema);
break;
case USER_DATA_TYPES:
readUserDataTypes(child, schema);
break;
case FUNCTIONS:
readFunctions(child, schema);
break;
case PROCEDURES:
readProcedures(child, schema);
break;
case TABLES:
readTables(child, schema);
break;
default:
break;
}
}
}
private static void readUserDataTypes(Element element,
RdbSchema schema) {
for (final Element child : element.getChildren(Element.class)) {
readUserDataType(child, schema);
}
}
private static void readUserDataType(Element element,
RdbSchema schema) {
final String name = element.getAttributeValue(NAME, null);
final RdbUserDataType udt = schema.createUserDataType(name);
udt.setClassName(element.getAttributeValue(CLASS_NAME, null));
udt.setType(element.getAttributeAsEnum(TYPE, SqlDataType.class, null));
for (final Element child : element.getChildren(Element.class)) {
switch (child.getName()) {
case COMMENTS:
readComments(child, udt);
break;
case ATTRIBUTE:
readAttribute(child, udt);
break;
default:
break;
}
}
}
private static void readAttribute(Element element,
RdbUserDataType udt) {
final String name = element.getAttributeValue(NAME, null);
final RdbAttribute attribute = udt.createAttribute(name);
// TODO
for (final Element child : element.getChildren(Element.class)) {
if (COMMENTS.equals(child.getName())) {
readComments(child, attribute);
}
}
}
private static void readFunctions(Element element,
RdbSchema catalog) {
for (final Element child : element.getChildren(Element.class)) {
readFunction(child, catalog);
}
}
private static void readFunction(Element element,
RdbSchema schema) {
final String name = element.getAttributeValue(NAME, null);
final RdbFunction function = schema.createFunction(name);
function.setSpecificName(element.getAttributeValue(SPECIFIC_NAME, null));
function.setResultType(element.getAttributeAsEnum(RESULT_TYPE, FunctionResultType.class, null));
for (final Element child : element.getChildren(Element.class)) {
switch (child.getName()) {
case COMMENTS:
readComments(child, function);
break;
case COLUMN:
readFunctionColumn(child, function);
break;
default:
break;
}
}
}
private static void readFunctionColumn(Element element,
RdbFunction function) {
final String name = element.getAttributeValue(NAME, null);
final RdbFunctionColumn column = function.createColumn(name);
// TODO
for (final Element child : element.getChildren(Element.class)) {
if (COMMENTS.equals(child.getName())) {
readComments(child, column);
}
}
}
private static void readProcedures(Element element,
RdbSchema schema) {
for (final Element child : element.getChildren(Element.class)) {
readProcedure(child, schema);
}
}
private static void readProcedure(Element element,
RdbSchema schema) {
final String name = element.getAttributeValue(NAME, null);
final RdbProcedure procedure = schema.createProcedure(name);
procedure.setSpecificName(element.getAttributeValue(SPECIFIC_NAME, null));
procedure.setResultType(element.getAttributeAsEnum(RESULT_TYPE, ProcedureResultType.class, null));
// TODO
for (final Element child : element.getChildren(Element.class)) {
switch (child.getName()) {
case COMMENTS:
readComments(child, procedure);
break;
case COLUMN:
readProcedureColumn(child, procedure);
break;
default:
break;
}
}
}
private static void readProcedureColumn(Element element,
RdbProcedure procedure) {
final String name = element.getAttributeValue(NAME, null);
final RdbProcedureColumn column = procedure.createColumn(name);
// TODO
for (final Element child : element.getChildren(Element.class)) {
if (COMMENTS.equals(child.getName())) {
readComments(child, column);
}
}
}
private static void readTables(Element element,
RdbSchema schema) {
for (final Element child : element.getChildren(Element.class)) {
readTable(child, schema);
}
}
private static void readTable(Element element,
RdbSchema schema) {
final String name = element.getAttributeValue(NAME, null);
final RdbTable table = schema.createTable(name);
table.setTableTypeName(element.getAttributeValue(TABLE_TYPE, null));
// TODO
for (final Element child : element.getChildren(Element.class)) {
switch (child.getName()) {
case COMMENTS:
readComments(child, table);
break;
case PRIMARY_KEY:
readPrimaryKey(child, table);
break;
case FOREIGN_KEYS:
readForeignKeys(child, table);
break;
case COLUMNS:
readTableColumns(child, table);
break;
case INDICES:
readIndices(child, table);
break;
default:
break;
}
}
}
private static void readPrimaryKey(Element element,
RdbTable table) {
final String name = element.getAttributeValue(NAME, null);
final RdbPrimaryKey key = table.createPrimaryKey(name);
for (final Element child : element.getChildren(Element.class)) {
readPrimaryKeyColumn(child, key);
}
}
private static void readPrimaryKeyColumn(Element element,
RdbPrimaryKey key) {
final String name = element.getAttributeValue(NAME, null);
final RdbPrimaryKeyColumn column = key.createColumn(name);
column.setOrdinal(element.getAttributeAsShort(ORDINAL, (short) -1));
for (final Element child : element.getChildren(Element.class)) {
if (COMMENTS.equals(child.getName())) {
readComments(child, column);
}
}
}
private static void readForeignKeys(Element element,
RdbTable table) {
for (final Element child : element.getChildren(Element.class)) {
readForeignKey(child, table);
}
}
private static void readForeignKey(Element element,
RdbTable table) {
final String name = element.getAttributeValue(NAME, null);
final RdbForeignKey key = table.createForeignKey(name);
key.setRefCatalogName(element.getAttributeValue(REF_CATALOG, null));
key.setRefSchemaName(element.getAttributeValue(REF_SCHEMA, null));
key.setRefTableName(element.getAttributeValue(REF_TABLE, null));
// TODO
for (final Element child : element.getChildren(Element.class)) {
switch (child.getName()) {
case COMMENTS:
readComments(child, table);
break;
case COLUMN:
readForeignKeyColumn(child, key);
break;
default:
break;
}
}
}
private static void readForeignKeyColumn(Element element,
RdbForeignKey key) {
final String name = element.getAttributeValue(NAME, null);
final RdbForeignKeyColumn column = key.createColumn(name);
column.setOrdinal(element.getAttributeAsShort(ORDINAL, (short) -1));
column.setRefColumnName(element.getAttributeValue(REF_COLUMN, null));
for (final Element child : element.getChildren(Element.class)) {
if (COMMENTS.equals(child.getName())) {
readComments(child, key);
}
}
}
private static void readTableColumns(Element element,
RdbTable table) {
for (final Element child : element.getChildren(Element.class)) {
readTableColumn(child, table);
}
}
private static void readTableColumn(Element element,
RdbTable table) {
final String name = element.getAttributeValue(NAME, null);
final RdbTableColumn column = table.createColumn(name);
column.setAutoIncrement(element.getAttributeAsEnum(AUTO_INCREMENT, YesNoUnknown.class, null));
column.setDataType(element.getAttributeAsEnum(DATA_TYPE, SqlDataType.class, null));
column.setDefaultValue(element.getAttributeValue(DEFAULT_VALUE, null));
column.setDigits(element.getAttributeAsInt(DIGITS, -1));
column.setGenerated(element.getAttributeAsEnum(GENERATED, YesNoUnknown.class, null));
column.setNullable(element.getAttributeAsEnum(NULLABLE, YesNoUnknown.class, null));
column.setOrdinal(element.getAttributeAsInt(ORDINAL, -1));
column.setRadix(element.getAttributeAsInt(RADIX, -1));
column.setSize(element.getAttributeAsInt(SIZE, -1));
column.setTypeName(element.getAttributeValue(TYPE, null));
// TODO
for (final Element child : element.getChildren(Element.class)) {
if (COMMENTS.equals(child.getName())) {
readComments(child, column);
}
}
}
private static void readIndices(Element element,
RdbTable table) {
for (final Element child : element.getChildren(Element.class)) {
readIndex(child, table);
}
}
private static void readIndex(Element element,
RdbTable table) {
final String name = element.getAttributeValue(NAME, null);
final RdbIndex index = table.createIndex(name);
index.setType(element.getAttributeAsEnum(TYPE, RdbIndexType.class, null));
// TODO
for (final Element child : element.getChildren(Element.class)) {
switch (child.getName()) {
case COMMENTS:
readComments(child, index);
break;
case COLUMN:
readIndexColumn(child, index);
break;
default:
break;
}
}
}
private static void readIndexColumn(Element element,
RdbIndex index) {
final String name = element.getAttributeValue(NAME, null);
final RdbIndexColumn column = index.createColumn(name);
column.setOrdinal(element.getAttributeAsShort(ORDINAL, (short) -1));
// TODO
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy