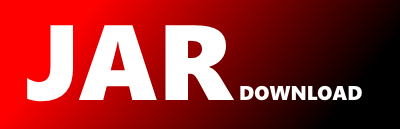
cdc.util.rdb.tools.dump.AbstractHandler Maven / Gradle / Ivy
package cdc.util.rdb.tools.dump;
import java.io.File;
import java.io.IOException;
import java.util.List;
/**
* Base class of specific dump handlers (XML, CSV).
*
*
* {@code
*
* startTable()
* header()
*
* startRow()
*
* column()
* endRow()
* endTable()
* }
*
*
* @author Damien Carbonne
*
*/
abstract class AbstractHandler {
protected final RdbDump.MainArgs margs;
protected AbstractHandler(RdbDump.MainArgs margs) {
this.margs = margs;
}
/**
* Returns the file to use to dump a table.
*
* It is built as: {@code outputDir + "/" + (prefix) ? + basename + "." + extension}.
*
* @param basename The file base name.
* @return The file to use to dump a table.
*/
protected File getFile(String basename) {
return new File(margs.outputDir, (margs.prefix == null ? "" : margs.prefix) + basename + "." + getExtension());
}
/**
* @return The file extension associated to this handler.
*/
public abstract String getExtension();
/**
* Invoked at the beginning of each table to dump.
*
* @param basename Base name to be used for file name.
* @param tableName Name of the dumped table.
* @throws IOException When an IO exception occurs.
*/
public abstract void startTable(String basename,
String tableName) throws IOException;
/**
* Invoked just after startTable.
*
* @param columnNames A list of column names to be dumped.
* @throws IOException When an IO exception occurs.
*/
public abstract void header(List columnNames) throws IOException;
/**
* Invoked at the beginning of each row.
*
* @param rowIndex Index of the row to be dumped.
* @throws IOException When an IO exception occurs.
*/
public abstract void startRow(int rowIndex) throws IOException;
/**
* Invoked for each column of current row.
*
* @param name The column name.
* @param value The column value.
* @throws IOException When an IO exception occurs.
*/
public abstract void column(String name,
String value) throws IOException;
/**
* Invoked at the end of each row.
*
* @throws IOException When an IO exception occurs.
*/
public abstract void endRow() throws IOException;
/**
* Invoked at the end of each table.
*
* @throws IOException When an IO exception occurs.
*/
public abstract void endTable() throws IOException;
}