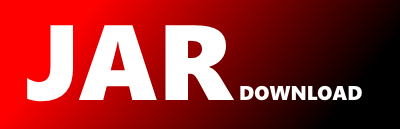
br.com.jhonsapp.bootstrap.test.restTemplate.command.GetCommand Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bootstrap-test Show documentation
Show all versions of bootstrap-test Show documentation
A complete architecture for creating and managing tests.
package br.com.jhonsapp.bootstrap.test.restTemplate.command;
import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.get;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.content;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.status;
import java.util.List;
import java.util.Map;
import org.springframework.test.web.servlet.MockMvc;
import org.springframework.test.web.servlet.ResultActions;
import org.springframework.test.web.servlet.request.MockHttpServletRequestBuilder;
import org.springframework.util.StringUtils;
import com.fasterxml.jackson.databind.ObjectMapper;
public class GetCommand {
private static final String authorizationKey = "Authorization";
private ObjectMapper mapper = new ObjectMapper();
private AuthenticationCommand authentication;
private Class typeClass;
private String requestMapping;
public GetCommand(AuthenticationCommand authentication, Class typeClass, String requestMapping) {
this.authentication = authentication;
this.typeClass = typeClass;
this.requestMapping = requestMapping;
}
private ResultActions obtainResultActions(String restMapping, MockMvc mockMvc, Map param) throws Exception {
MockHttpServletRequestBuilder builder = null;
if(StringUtils.hasText(restMapping))
builder = get("/" + requestMapping+ "/" + restMapping);
else
builder = get("/" + requestMapping);
//Add token authorization
builder.header(authorizationKey, authentication.obtainHeaderAuthorization(mockMvc))
.accept("application/json;charset=UTF-8");
//Add param if exist
if(param != null)
for(String s: param.keySet())
builder.param(s, param.get(s));
return mockMvc.perform(builder);
}
private String getStringObject(String restMapping, MockMvc mockMvc, Map param) throws Exception {
ResultActions result = obtainResultActions(restMapping, mockMvc, param)
.andExpect(content().contentType("application/json;charset=UTF-8"))
.andExpect(status().isOk());
return result.andReturn().getResponse().getContentAsString();
}
public List getList(MockMvc mockMvc) throws Exception {
String resultString = getStringObject("", mockMvc, null);
return mapper.readValue(resultString, mapper.getTypeFactory().constructCollectionType(List.class, typeClass));
}
public List getList(String restMapping, MockMvc mockMvc) throws Exception {
String resultString = getStringObject(restMapping, mockMvc, null);
return mapper.readValue(resultString, mapper.getTypeFactory().constructCollectionType(List.class, typeClass));
}
public List getList(String restMapping, MockMvc mockMvc, Map param) throws Exception {
String resultString = getStringObject(restMapping, mockMvc, param);
return mapper.readValue(resultString, mapper.getTypeFactory().constructCollectionType(List.class, typeClass));
}
public T isOk(MockMvc mockMvc) throws Exception {
String resultString = getStringObject("", mockMvc, null);
return mapper.readValue(resultString, typeClass);
}
public T isOk(String restMapping, MockMvc mockMvc) throws Exception {
String resultString = getStringObject(restMapping, mockMvc, null);
return mapper.readValue(resultString, typeClass);
}
public T isOk(String restMapping, MockMvc mockMvc, Map param) throws Exception {
String resultString = getStringObject(restMapping, mockMvc, param);
return mapper.readValue(resultString, typeClass);
}
public void isBadRequest(String restMapping, MockMvc mockMvc) throws Exception {
obtainResultActions(restMapping, mockMvc, null).andExpect(status().isBadRequest());
}
public void isBadRequest(String restMapping, MockMvc mockMvc, Map param) throws Exception {
obtainResultActions(restMapping, mockMvc, param).andExpect(status().isBadRequest());
}
public void isNotFound(String restMapping, MockMvc mockMvc) throws Exception {
obtainResultActions(restMapping, mockMvc, null).andExpect(status().isNotFound());
}
public void isNotFound(String restMapping, MockMvc mockMvc, Map param) throws Exception {
obtainResultActions(restMapping, mockMvc, param).andExpect(status().isNotFound());
}
public void isNoContent(String restMapping, MockMvc mockMvc) throws Exception {
obtainResultActions(restMapping, mockMvc, null).andExpect(status().isNoContent());
}
public void isNoContent(String restMapping, MockMvc mockMvc, Map param) throws Exception {
obtainResultActions(restMapping, mockMvc, param).andExpect(status().isNoContent());
}
public void isUnauthorized(String restMapping, MockMvc mockMvc) throws Exception {
obtainResultActions(restMapping, mockMvc, null).andExpect(status().isUnauthorized());
}
public void isUnauthorized(String restMapping, MockMvc mockMvc, Map param) throws Exception {
obtainResultActions(restMapping, mockMvc, param).andExpect(status().isUnauthorized());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy