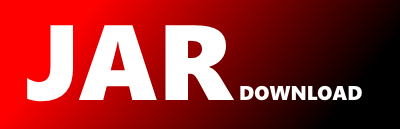
br.com.jhonsapp.bootstrap.user.model.User Maven / Gradle / Ivy
package br.com.jhonsapp.bootstrap.user.model;
import java.io.Serializable;
import java.util.List;
import br.com.jhonsapp.bootstrap.user.model.roleGroup.RoleGroup;
import br.com.jhonsapp.bootstrap.user.model.token.android.FirebaseToken;
/**
* This interface represents a final user in a application.
*
* @see RoleGroup
*
* @author Jhonathan Camacho
* @author Jhonys Camacho
*
*/
public interface User extends Serializable {
/**
* Returns the name.
*
* @return the name.
*/
public String getName();
/**
* Sets the name.
*
* @param name
* the user name.
*/
public void setName(String name);
/**
* Returns the user name.
*
* @return the user name.
*/
public String getUserName();
/**
* Sets the user name.
*
* @param userName
* the user e-mail. The e-mail shuold be valid, i.e., not null
* and with domain (e.g., [email protected]).
*/
public void setUserName(String userName);
/**
* Returns the user password.
*
* @return the user password
*/
public String getPassword();
/**
* Sets the user password.
*
* @param password
* the user password.
*/
public void setPassword(String password);
/**
* Adds a role group to a user's role group list.
*
* @param roleGroup
* the role group added to a user'r role group list.
*/
public void addRoleGroup(RoleGroup roleGroup);
/**
* Remove a role group to a user's role group list.
*
* @param roleGroup
* the tole group removed from a user's role group list.
*/
public void removeRoleGroup(RoleGroup roleGroup);
/**
* Returns the user's role group list.
*
* @return the user's role group list.
*/
public List getRoleGroups();
/**
* Returns the android token.
*
* @return the android token
*/
public FirebaseToken getAndroidToken();
/**
* Returns the code that makes it possible to update the password.
*
* @return the code that makes it possible to update the password.
*/
public String getResetPasswordCode();
/**
* Returns true if the given code is equal to the generated code and false
* otherwise.
*
* @param code
* that will be validated.
*
* @return true if the given code is equal to the generated code and false
* otherwise.
*/
public boolean isValidResetPasswordCode(String code);
/**
* Clears the code used to reset the password.
*/
public void clearResetPasswordCode();
/**
* Returns true if the user is active and false otherwise.
*
* @return true if the user is active and false otherwise.
*/
public boolean isActive();
/**
* Adds true for the user to be marked as active and false otherwise.
*
* @param active
* true if the user is active and false otherwise.
*/
public void setActive(boolean active);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy