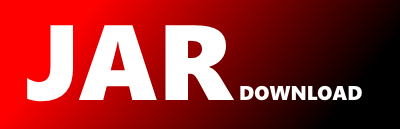
br.com.jhonsapp.bootstrap.user.resource.UserResource Maven / Gradle / Ivy
package br.com.jhonsapp.bootstrap.user.resource;
import java.util.List;
import java.util.Optional;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.validation.Valid;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.ApplicationEventPublisher;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.security.access.prepost.PreAuthorize;
import org.springframework.web.bind.annotation.DeleteMapping;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.PutMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import br.com.jhonsapp.bootstrap.user.event.ResourceCreatedEvent;
import br.com.jhonsapp.bootstrap.user.model.FinalUser;
import br.com.jhonsapp.bootstrap.user.service.UserService;
import br.com.jhonsapp.bootstrap.util.RefreshTokenUtil;
@RestController
@RequestMapping("/users")
public class UserResource {
@Autowired
private RefreshTokenUtil refreshTokenUtil;
@Autowired
private ApplicationEventPublisher publisher;
@Autowired
private UserService userService;
@GetMapping
@PreAuthorize("hasAuthority('ROLE_CONSULT_USER') and #oauth2.hasScope('read')")
public List findAll(){
return userService.findAll();
}
@GetMapping("/findByUserName")
@PreAuthorize("hasAuthority('ROLE_CONSULT_USER') and #oauth2.hasScope('read')")
public ResponseEntity findByUserName(@RequestParam String userName){
Optional finalUser = userService.findUser(userName);
return finalUser.isPresent() ? ResponseEntity.ok(finalUser.get()) : ResponseEntity.notFound().build();
}
@GetMapping("/findById/{id}")
@PreAuthorize("hasAuthority('ROLE_CONSULT_USER') and #oauth2.hasScope('read')")
public ResponseEntity findById(@PathVariable Long id){
FinalUser finalUser = userService.findById(id);
return finalUser != null ? ResponseEntity.ok(finalUser) : ResponseEntity.notFound().build();
}
@PostMapping
@PreAuthorize("hasAuthority('ROLE_CREATE_USER') and #oauth2.hasScope('write')")
public ResponseEntity save(@Valid @RequestBody FinalUser user, HttpServletResponse response){
FinalUser finalUserSaved = userService.save(user);
publisher.publishEvent(new ResourceCreatedEvent(this, response, finalUserSaved.getId()));
return ResponseEntity.status(HttpStatus.CREATED).body(finalUserSaved);
}
@PutMapping("/{id}")
@PreAuthorize("hasAuthority('ROLE_UPDATE_USER') and #oauth2.hasScope('write')")
public ResponseEntity update(@PathVariable Long id, @Valid @RequestBody FinalUser user) {
FinalUser finalUserSaved = userService.update(id, user);
return ResponseEntity.ok(finalUserSaved);
}
@DeleteMapping("/logout")
public void logout(HttpServletRequest req, HttpServletResponse resp) {
refreshTokenUtil.invalidateRefreshTokenCookie(req, resp);
resp.setStatus(HttpStatus.NO_CONTENT.value());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy