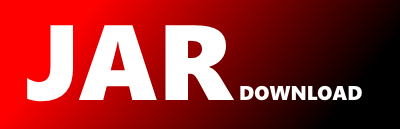
br.com.jhonsapp.bootstrap.user.service.UserService Maven / Gradle / Ivy
package br.com.jhonsapp.bootstrap.user.service;
import java.util.List;
import java.util.Optional;
import br.com.jhonsapp.bootstrap.user.model.FinalUser;
/**
* This interface describes the methods that all user service classes, from the
* same project, should have.
*
* @author Jhonathan Camacho
*
*/
public interface UserService {
/**
* Persisted user.
*
* @param user
* the user.
* @return an object or null if the query does not return results.
*/
public FinalUser save(FinalUser user);
/**
* Updated user.
*
* @param id
* the id user.
*
* @param user
* the object user.
* @return an object or null if the query does not return results.
*/
public FinalUser update(long id, FinalUser user);
/**
* Check if the username is valid.
*
* @param username
* the username
*
* @return true if the username is valid and false otherwise.
*/
public boolean isUserNameValid(String username);
/**
* Check if the user's password is valid.
*
* @param password
* the user's password
*
* @return true if the password is valid and false otherwise.
*/
public boolean isPasswordValid(String password);
/**
* Search for an object through its identifier.
*
* @param id
* the identifier of object.
*
* @return an object or null if the query does not return results.
**/
public FinalUser findById(long id);
/**
* Find all persisted user.
*
* @return a list with all user persisted in the database.
*/
public List findAll();
/**
* Returns a user through user email.
*
* @param userName
* the user's userName
*
* @return a user through user email.
*/
public Optional findUser(String userName);
/**
* Returns the android token through the user's email.
*
* @param userName
* the user's userName
*
* @return the android token through the user's email.
*/
public String findAndroidToken(String userName);
/**
* Send the code to the email.
*
* @param email
* the user's email
*
* @return True If the code was sent and false otherwise.
*/
public boolean sendResetPasswordCodeToEmail(String email);
/**
* Updates the user's password.
*
* @param userName
* the user's userName
* @param code
* the user's code
* @param password
* the user's password
*
* @return True if the password was reset and false otherwise.
*/
public boolean resetPassword(String userName, String code, String password);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy