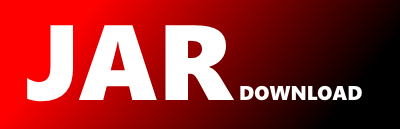
br.com.jhonsapp.file.manager.contract.FileManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of file-manager Show documentation
Show all versions of file-manager Show documentation
File Manager is an open source project created by Jhonys Camacho and Jhonathan Camacho to facilitate the file management in local and remote repositories.
package br.com.jhonsapp.file.manager.contract;
import java.io.File;
import java.io.Serializable;
/**
* Interface responsible for manipulating files in a specific directory.
*
* @author Jhonathan Camacho
*
*/
public interface FileManager extends Serializable {
/**
* Inserts or updates a file in the directory.
*
* @param fileName
* file name.
* @param format
* file format.
* @param file
* file to be inserted or updated.
*
* @return true if the insert or update operation was successful and false
* otherwise.
*/
public boolean insert(String fileName, String format, byte[] file);
/**
* Remove a file in the directory.
*
* @param fileName
* file name.
*
* @return true if the removal was successful and false otherwise.
*/
public boolean remove(String fileName);
/**
* Gets a file in byte format.
*
* @param fileName
* file name.
*
* @return if a file exists it will be returned in byte format otherwise it returns null.
*/
public byte[] getByte(String fileName);
/**
* Gets a file.
*
* @param fileName
* file name.
*
* @return if a file exists it will be returned otherwise it returns null.
*/
public File getFile(String fileName);
/**
* Checks whether the file exists in the directory.
*
* @param fileName
* file name.
*
* @return if the file exists it returns true otherwise false.
*/
public boolean hasFile(String fileName);
/**
* Gets the path of the repository.
*
* @return the repository path.
*/
public String getRepositoryPath();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy