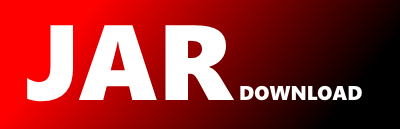
br.com.jhonsapp.file.manager.implementation.LocalHDFileManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of file-manager Show documentation
Show all versions of file-manager Show documentation
File Manager is an open source project created by Jhonys Camacho and Jhonathan Camacho to facilitate the file management in local and remote repositories.
package br.com.jhonsapp.file.manager.implementation;
import java.io.BufferedInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.nio.file.FileSystemNotFoundException;
import br.com.jhonsapp.file.manager.contract.AbstractFileManager;
import br.com.jhonsapp.util.verifier.StringVerifier;
/**
* This class is responsible for manipulating files in a local directory.
*
* @author Jhonathan Camacho
*/
public class LocalHDFileManager extends AbstractFileManager {
/**
* Generated serial version id created at 09 July 2017.
*/
private static final long serialVersionUID = -4504893324193495328L;
public LocalHDFileManager(String repositoryPath) {
super(repositoryPath);
}
@Override
public boolean insert(String fileName, String format, byte[] file) {
if(StringVerifier.isBlanck(format))
throw new IllegalArgumentException("The format cannot be null.");
if(file == null || file.length == 0)
throw new IllegalArgumentException("The content cannot be null.");
try {
this.remove(fileName);
String path = getRepositoryPath();
FileOutputStream fileOutput = new FileOutputStream(path + fileName + "." + format);
fileOutput.write(file);
fileOutput.close();
return true;
} catch (IOException e) {
return false;
}
}
@Override
public boolean remove(String fileName) {
if (this.hasFile(fileName)) {
File file = this.findFile(fileName);
file.delete();
return true;
} else {
return false;
}
}
@Override
public byte[] getByte(String fileName) {
try {
File imagem = this.findFile(fileName);
if (imagem != null) {
BufferedInputStream in = new BufferedInputStream(new FileInputStream(imagem));
byte[] bytes = new byte[in.available()];
in.read(bytes);
in.close();
return bytes;
} else {
return null;
}
} catch (IOException e) {
throw new FileSystemNotFoundException(
"There was an error converting the file to the byte array. Possibly the file does not exist.");
}
}
@Override
public File getFile(String fileName) {
return this.findFile(fileName);
}
@Override
public boolean hasFile(String fileName) {
return this.findFile(fileName) != null;
}
private File findFile(String fileName) {
if(StringVerifier.isBlanck(fileName))
throw new IllegalArgumentException("The fileName cannot be null.");
File arquivo = null;
File[] lista = new File(this.getRepositoryPath()).listFiles();
for (int i = 0; i < lista.length; i++) {
if (lista[i].getName().contains(fileName)) {
arquivo = lista[i];
break;
}
}
return arquivo;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy