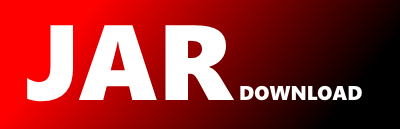
br.com.jhonsapp.repository.implementation.Repository Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of repository Show documentation
Show all versions of repository Show documentation
Repository is a project created by Jhonathan Camacho to facilitate the file management in local and remote repositories.
package br.com.jhonsapp.repository.implementation;
import java.io.Serializable;
import br.com.jhonsapp.repository.object.Object;
/**
* Interface responsible for manipulating files in a specific repository.
*
* @author Jhonathan Camacho
*
*/
public interface Repository extends Serializable {
public static final String LOCAL = "LOCAL";
public static final String REMOTE = "REMOTE";
public static final String AMAZON = "AMAZON";
/**
* Inserts or updates a object in the repository.
*
* @param object
* representation of the object to be inserted or updated.
*
* @return true if the insert or update operation was successful and false
* otherwise.
*/
public boolean archive(Object object);
/**
* Remove a object in the repository.
*
* @param folder
* the name of the folder in which the object is located.
* @param id
* the object id.
*
* @return true if the removal was successful and false otherwise.
*/
public boolean unarchive(String folder, String id);
/**
* Search for a specific object in a specific folder.
*
* @param folder
* the name of the folder in which the object is located.
* @param id
* the object id.
*
* @return if a file exists it will be returned otherwise it returns null.
*/
public Object search(String folder, String id);
/**
* Checks whether the file exists in the directory.
*
* @param folder
* the name of the folder in which the object is located.
* @param id
* the object id.
*
* @return if the file exists it returns true otherwise false.
*/
public boolean hasArchive(String folder, String id);
/**
* Create a folder in the repository.
*
* @param name
* the name of the folder in the repository.
*
* @return true if the folder was successfully created and false otherwise.
*/
public boolean createFolder(String name);
/**
* Checks whether the folser exists in the repository.
*
* @param name
* the name of the folder in the repository.
*
* @return if the file exists it returns true otherwise false.
*/
public boolean hasFolder(String name);
/**
* Gets the path of the repository.
*
* @return the repository path.
*/
public String getRepositoryPath();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy