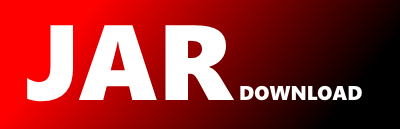
br.com.jhonsapp.repository.util.FileUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of repository Show documentation
Show all versions of repository Show documentation
Repository is a project created by Jhonathan Camacho to facilitate the file management in local and remote repositories.
package br.com.jhonsapp.repository.util;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.nio.file.FileSystemNotFoundException;
/**
* This class is a file utility.
*
* @author Jhonathan Camacho
*/
public class FileUtil {
/**
* Convert the file in bytes.
*
* @param file
* the file to be converted.
*
* @return the file in byte.
*
* @throws FileSystemNotFoundException
* if was an error converting the file to the byte array.
*/
public static byte[] convertToByte(File file) {
try {
BufferedInputStream in = new BufferedInputStream(new FileInputStream(file));
byte[] bytes = new byte[in.available()];
in.read(bytes);
in.close();
return bytes;
} catch (IOException e) {
throw new FileSystemNotFoundException(
"There was an error converting the file to the byte array. Possibly the file does not exist.");
}
}
/**
* Convert the bytes in file.
*
* @param bytes
* the bytes to be converted.
*
* @param fileName
* the name of the file converted.
*
* @return the byte in file.
*
* @throws FileSystemNotFoundException
* if was an error converting the byte array to the file.
*/
public static File convertToFile(byte[] bytes, String fileName) {
try {
File file = new File(fileName);
BufferedOutputStream bos = new BufferedOutputStream(new FileOutputStream(file));
bos.write(bytes);
bos.close();
return file;
} catch (IOException e) {
throw new FileSystemNotFoundException(
"There was an error converting the byte array to the file. Possibly the file does not exist.");
}
}
/**
* Verify if the bytes is null or empty.
*
* @param bytes
* the bytes that will be verified.
*
* @return true if the bytes is null or empty. Return false otherwise.
*/
public static boolean isInvalid(byte[] bytes) {
return bytes == null || bytes.length == 0;
}
/**
* Verify if the bytes is not blank.
*
* @param bytes
* the bytes that will be verified.
*
* @return true if the bytes is not blank. Return false otherwise.
*/
public static boolean isValid(byte[] bytes) {
return bytes != null && bytes.length > 0;
}
/**
* Verify if the file is null or empty.
*
* @param file
* the bytes that will be verified.
*
* @return true if the bytes is null or empty. Return false otherwise.
*/
public static boolean isInvalid(File file) {
return file == null || file.length() == 0;
}
/**
* Verify if the file is not blank.
*
* @param file
* the bytes that will be verified.
*
* @return true if the bytes is not blank. Return false otherwise.
*/
public static boolean isValid(File file) {
return file != null && file.length() > 0;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy