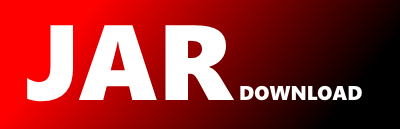
br.com.jhonsapp.repository.object.ObjectImpl Maven / Gradle / Ivy
package br.com.jhonsapp.repository.object;
import java.io.File;
import org.springframework.util.StringUtils;
import br.com.jhonsapp.repository.util.FileUtil;
import br.com.jhonsapp.repository.util.PathUtil;
/**
* An abstract representation of image.
*
* @see Object
*
* @author Jhonathan Camacho.
*/
public class ObjectImpl implements Object {
/**
* Generated serial version id created at 14 July 2017.
*/
private static final long serialVersionUID = 8962259017237194771L;
private String folder;
private String id;
private byte[] bytes;
private File file;
private ObjectImpl(String folder, String id, byte[] file) {
if (!StringUtils.hasText(id))
throw new IllegalArgumentException("The id cannot be null.");
if (FileUtil.isInvalid(file))
throw new IllegalArgumentException("The content cannot be null.");
this.folder = folder;
this.id = id;
this.bytes = file;
}
private ObjectImpl(String folder, String id, File file) {
if (!StringUtils.hasText(id))
throw new IllegalArgumentException("The id cannot be null.");
if (FileUtil.isInvalid(file))
throw new IllegalArgumentException("The content cannot be null.");
this.folder = folder;
this.id = id;
this.file = file;
}
@Override
public String getId() {
return id;
}
@Override
public String getFolder() {
return PathUtil.pathFormat(folder);
}
@Override
public String getAbsolutePath() {
if (!StringUtils.hasText(getFolder()))
return getId();
else
return getFolder() + File.separatorChar + getId();
}
@Override
public File getContentsInFile() {
if (FileUtil.isInvalid(file))
return FileUtil.convertToFile(bytes, getAbsolutePath());
else
return file;
}
@Override
public byte[] getContentsInByte() {
if (FileUtil.isInvalid(bytes))
return FileUtil.convertToByte(file);
else
return bytes;
}
public static final Object createObject(String folder, String id, byte[] file) {
return new ObjectImpl(folder, id, file);
}
public static final Object createObject(String folder, String id, File file) {
return new ObjectImpl(folder, id, file);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy