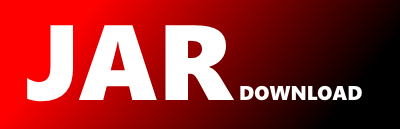
br.com.jhonsapp.util.primefaces.uploaded.ServletUtil Maven / Gradle / Ivy
package br.com.jhonsapp.util.primefaces.uploaded;
import java.io.IOException;
import java.net.URLDecoder;
import java.nio.file.Files;
import javax.servlet.ServletContext;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import br.com.jhonsapp.repository.concept.Repository;
import br.com.jhonsapp.repository.object.Object;
import br.com.jhonsapp.repository.util.PathUtil;
import br.com.jhonsapp.util.verifier.StringVerifier;
/**
* Class responsible for manipulating files within the servlet.
*
* @author Jhonathan Camacho
*
*/
public class ServletUtil {
/**
* Loads a file from a specific repository of an HTTP request into the
* servlet.
*
* @param repository
* responsible for manipulating files in a specific repository.
*
* @param request
* provide request information for HTTP servlets.
*
* @param response
* provide HTTP-specific functionality in sending a response.
*
* @param servletContext
* represents the servlet context.
* @throws IOException
* used when you have an error loading the information.
*
*/
public static void loadFile(Repository repository, HttpServletRequest request, HttpServletResponse response,
ServletContext servletContext) throws IOException {
if (repository == null)
throw new IllegalArgumentException("The Repository cannot be null.");
if (request == null)
throw new IllegalArgumentException("The HttpServletRequest cannot be null.");
if (response == null)
throw new IllegalArgumentException("The HttpServletResponse cannot be null.");
if (servletContext == null)
throw new IllegalArgumentException("The ServletContext cannot be null.");
checkURLInfo(request, response);
String id = getId(request);
String folder = getFolder(request);
Object object = repository.search(folder, id);
checkIfIsImage(object, response, servletContext);
writeImageToResponse(object, response, servletContext);
}
/**
* Check if file name is actually supplied to the request URI.
*
* @param request
* provide request information for HTTP servlets.
*
* @param response
* provide HTTP-specific functionality in sending a response.
*/
private static void checkURLInfo(HttpServletRequest request, HttpServletResponse response) throws IOException {
if (StringVerifier.isBlanck(request.getPathInfo()))
sendError(response);
}
/**
* Gets the identifier of the image.
*
* @param request
* provide request information for HTTP servlets.
*
* @return the identifier.
*/
private static String getId(HttpServletRequest request) throws IOException {
return PathUtil.getId(URLDecoder.decode(request.getPathInfo(), "UTF-8"));
}
/**
* Gets the path in the directory where the image is located.
*
* @param request
* provide request information for HTTP servlets.
*
* @return the path in the directory.
*/
private static String getFolder(HttpServletRequest request) throws IOException {
return PathUtil.getFolder(URLDecoder.decode(request.getPathInfo(), "UTF-8"));
}
/**
* Send a 404 error.
*
* @param response
* provide HTTP-specific functionality in sending a response.
*/
private static final void sendError(HttpServletResponse response) throws IOException {
response.sendError(HttpServletResponse.SC_NOT_FOUND); // 404.
return;
}
/**
* Check if file is actually an image (avoid download of other files by
* hackers!).
*
* @param object
* an abstract representation of the repository object.
*
* @param response
* provide HTTP-specific functionality in sending a response.
*
* @param servletContext
* represents the servlet context.
*/
private static void checkIfIsImage(Object object, HttpServletResponse response, ServletContext servletContext)
throws IOException {
if (object == null)
sendError(response);
String contentType = getContentType(object, servletContext);
if (contentType == null || !contentType.startsWith("image"))
sendError(response);
}
/**
* Gets the content type.
*
* @param object
* an abstract representation of the repository object.
*
* @param servletContext
* represents the servlet context.
*
* @return the content type.
*/
private static String getContentType(Object object, ServletContext servletContext) {
return servletContext.getMimeType(object.getContentsInFile().getName());
}
/**
* Copying the image into the response.
*
* @param object
* an abstract representation of the repository object.
*
* @param response
* provide HTTP-specific functionality in sending a response.
*
* @param servletContext
* represents the servlet context.
*
*/
private static void writeImageToResponse(Object object, HttpServletResponse response, ServletContext servletContext)
throws IOException {
response.reset();
response.setContentType(getContentType(object, servletContext));
response.setHeader("Content-Length", String.valueOf(object.getContentsInFile().length()));
Files.copy(object.getContentsInFile().toPath(), response.getOutputStream());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy