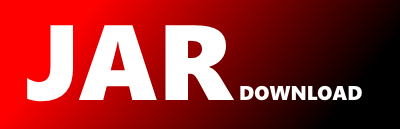
br.com.jhonsapp.util.primefaces.uploaded.UploadedFileUtilImpl Maven / Gradle / Ivy
package br.com.jhonsapp.util.primefaces.uploaded;
import javax.inject.Inject;
import org.primefaces.model.UploadedFile;
import br.com.jhonsapp.repository.concept.Repository;
import br.com.jhonsapp.repository.object.ObjectImpl;
import br.com.jhonsapp.repository.util.PathUtil;
import br.com.jhonsapp.util.jsf.project.information.WebProjectInformation;
import br.com.jhonsapp.util.verifier.StringVerifier;
/**
* Class responsible for loading files into the repository.
*
* @author Jhonathan Camacho
*
*/
public class UploadedFileUtilImpl implements UploadedFileUtil {
/**
* Generated serial version id created at 19 July 2017.
*/
private static final long serialVersionUID = 7753960891921754543L;
@Inject
private WebProjectInformation webProjectInformation;
private String servletName;
private Repository repository;
public UploadedFileUtilImpl(Repository repository, String servletName) {
if (StringVerifier.isBlanck(servletName))
throw new IllegalArgumentException("The servletName cannot be null.");
if (repository == null)
throw new IllegalArgumentException("The repository cannot be null.");
this.servletName = servletName;
this.repository = repository;
}
@Override
public String createImagePath(String fullPath) {
if (StringVerifier.isBlanck(fullPath))
throw new IllegalArgumentException("The fullPath cannot be null.");
return webProjectInformation.getHttpWebProject() + "/" + servletName + "/" + fullPath;
}
@Override
public boolean insertFile(UploadedFile uploadedFile, String fullPath) {
if (uploadedFile == null)
throw new IllegalArgumentException("The UploadedFile cannot be null.");
if (StringVerifier.isBlanck(fullPath))
throw new IllegalArgumentException("The fullPath cannot be null.");
if (!uploadedFile.getFileName().isEmpty())
return repository.archive(ObjectImpl.createObject(PathUtil.getFolder(fullPath),
PathUtil.getId(fullPath),
uploadedFile.getContents()));
else
return false;
}
@Override
public boolean removeFile(String fullPath) {
if (StringVerifier.isBlanck(fullPath))
throw new IllegalArgumentException("The fullPath cannot be null.");
return repository.unarchive(PathUtil.getFolder(fullPath), PathUtil.getId(fullPath));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy