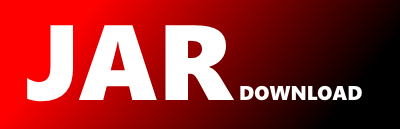
com.gitlab.oliverlj.jsonapi.configuration.ResourceConverterWrapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spring-boot-starter-json-api Show documentation
Show all versions of spring-boot-starter-json-api Show documentation
A spring boot starter which brings json api converter (https://github.com/jasminb/jsonapi-converter) for your great spring project
The newest version!
package com.gitlab.oliverlj.jsonapi.configuration;
import java.io.InputStream;
import java.lang.reflect.Type;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import org.eclipse.jdt.annotation.Nullable;
import org.springframework.core.GenericTypeResolver;
import org.springframework.data.domain.Page;
import com.fasterxml.jackson.databind.JavaType;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.type.SimpleType;
import com.fasterxml.jackson.databind.type.TypeFactory;
import com.github.jasminb.jsonapi.ConverterConfiguration;
import com.github.jasminb.jsonapi.DeserializationFeature;
import com.github.jasminb.jsonapi.JSONAPIDocument;
import com.github.jasminb.jsonapi.ResourceConverter;
import com.github.jasminb.jsonapi.SerializationFeature;
import com.github.jasminb.jsonapi.exceptions.DocumentSerializationException;
public class ResourceConverterWrapper {
private final ResourceConverter resourceConverter;
private final ObjectMapper objectMapper;
public ResourceConverterWrapper(ObjectMapper objectMapper, SpringBootStaterJsonApiProperties properties) {
Objects.requireNonNull(objectMapper, "An ObjectMapper must be provided.");
this.objectMapper = objectMapper;
this.resourceConverter = new ResourceConverter(objectMapper);
configureOptions(properties, resourceConverter);
}
private void configureOptions(SpringBootStaterJsonApiProperties properties, ResourceConverter resourceConverter) {
properties.getDisableDeserializationOptions().stream().map(DeserializationFeature::valueOf).forEach(resourceConverter::disableDeserializationOption);
properties.getEnableDeserializationOptions().stream().map(DeserializationFeature::valueOf).forEach(resourceConverter::enableDeserializationOption);
properties.getDisableSerializationOptions().stream().map(SerializationFeature::valueOf).forEach(resourceConverter::disableSerializationOption);
properties.getEnableSerializationOptions().stream().map(SerializationFeature::valueOf).forEach(resourceConverter::enableSerializationOption);
}
public boolean canReadOrWrite(Type type, @Nullable Class> contextClass) {
Class> clazz = getRawClass(type, contextClass);
if (clazz != null && supports(clazz) && !resourceConverter.isRegisteredType(clazz)) {
resourceConverter.registerType(clazz);
return true;
}
return resourceConverter.isRegisteredType(clazz);
}
private JavaType getJavaType(Type type, @Nullable Class> contextClass) {
TypeFactory typeFactory = this.objectMapper.getTypeFactory();
return typeFactory.constructType(GenericTypeResolver.resolveType(type, contextClass));
}
private @Nullable Class> getRawClass(Type type, @Nullable Class> contextClass) {
Class> rawClass = null;
JavaType javaType = getJavaType(type, contextClass);
if (javaType.isCollectionLikeType()) {
rawClass = javaType.getContentType().getRawClass();
} else if (SimpleType.class.isAssignableFrom(javaType.getClass())) {
if (Iterable.class.isAssignableFrom(javaType.getRawClass())) {
rawClass = javaType.getBindings().getBoundType(0).getRawClass();
} else {
rawClass = javaType.getRawClass();
}
}
return rawClass;
}
public Object deserialize(Type type, @Nullable Class> contextClass, InputStream inputStream) {
JavaType javaType = getJavaType(type, contextClass);
if (Iterable.class.isAssignableFrom(javaType.getRawClass())) {
JavaType itemType = javaType.getBindings().getBoundType(0);
List> collection = resourceConverter.readDocumentCollection(inputStream, itemType.getRawClass()).get();
return collection == null ? Collections.emptyList() : collection;
} else {
Object document = resourceConverter.readDocument(inputStream, javaType.getRawClass()).get();
return document == null ? Optional.empty() : document;
}
}
public boolean supports(Class> clazz) {
return ConverterConfiguration.isEligibleType(clazz);
}
@SuppressWarnings({"rawtypes", "unchecked"})
public byte[] serialize(Object object) throws DocumentSerializationException {
JSONAPIDocument jsonApiDocument;
if (JSONAPIDocument.class.isAssignableFrom(object.getClass())) {
jsonApiDocument = Objects.requireNonNull(JSONAPIDocument.class.cast(object));
} else if (Page.class.isAssignableFrom(object.getClass())) {
Page> page = Page.class.cast(object);
jsonApiDocument = new JSONAPIDocument<>(page.getContent());
jsonApiDocument.addMeta("totalPages", page.getTotalPages());
} else {
jsonApiDocument = new JSONAPIDocument<>(object);
}
Object document = jsonApiDocument.get();
if (document != null && Iterable.class.isAssignableFrom(document.getClass())) {
return resourceConverter.writeDocumentCollection(jsonApiDocument);
} else {
return resourceConverter.writeDocument(jsonApiDocument);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy