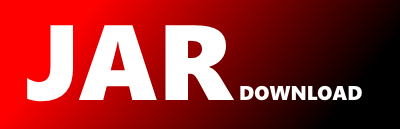
com.gitlab.spacetrucker.modularspringcontexts.SingletonBeanImportFactoryBean Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of modular-spring-contexts Show documentation
Show all versions of modular-spring-contexts Show documentation
Small utility library to allow modular definitions of Spring ApplicationContexts.
package com.gitlab.spacetrucker.modularspringcontexts;
import java.text.MessageFormat;
import javax.annotation.PostConstruct;
import org.springframework.beans.BeansException;
import org.springframework.beans.factory.NoSuchBeanDefinitionException;
import org.springframework.beans.factory.config.AbstractFactoryBean;
import org.springframework.context.ApplicationContext;
import org.springframework.context.ApplicationContextAware;
/**
* A factory bean for creating singleton imports of beans defined in an
* {@link ApplicationContext} into the ApplicationContext that is defining this
* import. The {@code import} element defined in {@code modular-spring-contexts.xsd} will
* trigger the initialization of an instance of this bean when using the default
* spring namespace resolution of spring xml context authoring.
*/
public class SingletonBeanImportFactoryBean extends AbstractFactoryBean
© 2015 - 2025 Weber Informatics LLC | Privacy Policy