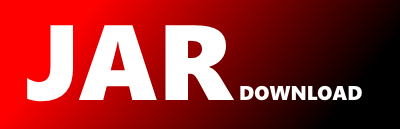
com.gitlab.spacetrucker.modularspringcontexts.module.ModularSpringContextsModuleBeanDefinitionParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of modular-spring-contexts Show documentation
Show all versions of modular-spring-contexts Show documentation
Small utility library to allow modular definitions of Spring ApplicationContexts.
package com.gitlab.spacetrucker.modularspringcontexts.module;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import org.springframework.beans.factory.config.RuntimeBeanReference;
import org.springframework.beans.factory.support.AbstractBeanDefinition;
import org.springframework.beans.factory.xml.AbstractBeanDefinitionParser;
import org.springframework.beans.factory.xml.ParserContext;
import org.springframework.context.support.GenericXmlApplicationContext;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
/**
* Bean definition parser for the module element defined in
* {@code /xsd/modular-spring-contexts.xsd}.
*/
public class ModularSpringContextsModuleBeanDefinitionParser extends AbstractBeanDefinitionParser {
/**
* The basic bean definition from which the module instance to be created is
* derived.
*/
private static final AbstractBeanDefinition MODULE_PROTOTYPE_DEFINITION;
static {
try (GenericXmlApplicationContext modulePrototypeContext = new GenericXmlApplicationContext(
"/module-prototype.xml")) {
MODULE_PROTOTYPE_DEFINITION = (AbstractBeanDefinition) modulePrototypeContext
.getBeanDefinition("modulePrototype");
}
}
/**
* Creates the bean definition that corresponds to a bean that represents a
* module.
*/
@Override
protected AbstractBeanDefinition parseInternal(Element element, ParserContext parserContext) {
List configuredLocations = getChildElementValues(element, "config", "location");
List requiredModules = getChildElementValues(element, "requires", "module");
String moduleStarter = element.getAttribute("moduleStarter");
AbstractBeanDefinition moduleDefinition = createBeanDefinition(configuredLocations, requiredModules,
moduleStarter);
return moduleDefinition;
}
/**
* Clones {@link #MODULE_PROTOTYPE_DEFINITION} and configures it to match
* the definition of the currently parsed {@code module} element.
*
* @param configLocations
* from which the module reads bean definitions
* @param requiredModules
* bean names of modules that the module defined by the element
* currently being parsed depends upon
* @param moduleStarter
* that is used to start the modules, {@code null} if none to use
* @return the newly created bean definition
*/
public static AbstractBeanDefinition createBeanDefinition(List configLocations,
List requiredModules, String moduleStarter) {
AbstractBeanDefinition moduleDefinition = MODULE_PROTOTYPE_DEFINITION.cloneBeanDefinition();
moduleDefinition.setAbstract(false);
moduleDefinition.getPropertyValues().add("configLocations", configLocations);
moduleDefinition.getPropertyValues().add("requiredModules", requiredModules);
if (moduleStarter != null && !moduleStarter.isEmpty()) {
moduleDefinition.getPropertyValues().add("moduleStarter", new RuntimeBeanReference(moduleStarter));
}
moduleDefinition.setLazyInit(false);
return moduleDefinition;
}
/**
* @param parent
* from which to read child element values
* @param childElementName
* used to filter child elements
* @param attributeName
* of child elements whose values are to be returned by this
* method
* @return the values of a specific attribute of child elements
*/
private List getChildElementValues(Node parent, String childElementName, String attributeName) {
NodeList nodeList = parent.getChildNodes();
List attributeValues = new ArrayList();
for (int i = 0; i < nodeList.getLength(); i++) {
Node node = nodeList.item(i);
if (node instanceof Element) {
Element element = (Element) node;
if (childElementName.equals(element.getLocalName())) {
attributeValues.add(element.getAttribute(attributeName));
}
}
}
return Collections.unmodifiableList(attributeValues);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy