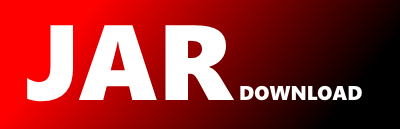
com.gitlab.summercattle.addons.wechat.common.service.impl.WeChatServiceImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cattle-addons-wechat-starter Show documentation
Show all versions of cattle-addons-wechat-starter Show documentation
Cattle Framework Addons WeChat Component
The newest version!
/*
* Copyright (C) 2018 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.gitlab.summercattle.addons.wechat.common.service.impl;
import java.util.Date;
import org.apache.commons.lang3.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.http.MediaType;
import org.springframework.stereotype.Service;
import com.alibaba.fastjson2.JSONObject;
import com.gitlab.summercattle.addons.wechat.common.AppType;
import com.gitlab.summercattle.addons.wechat.common.service.RidInfo;
import com.gitlab.summercattle.addons.wechat.common.service.WeChatService;
import com.gitlab.summercattle.addons.wechat.constants.WeChatConstants;
import com.gitlab.summercattle.addons.wechat.memory.TokenMemoryService;
import com.gitlab.summercattle.addons.wechat.miniprogram.configure.MiniProgramConfigProperties;
import com.gitlab.summercattle.addons.wechat.miniprogram.configure.MiniProgramApplication;
import com.gitlab.summercattle.addons.wechat.officialaccounts.configure.OfficialAccountsConfigProperties;
import com.gitlab.summercattle.addons.wechat.officialaccounts.configure.OfficialAccountsApplication;
import com.gitlab.summercattle.addons.wechat.utils.ApplicationUtils;
import com.gitlab.summercattle.commons.exception.CommonException;
import com.gitlab.summercattle.commons.utils.reflect.ClassType;
import com.gitlab.summercattle.commons.utils.reflect.ReflectUtils;
import com.gitlab.summercattle.commons.utils.spring.RestTemplateUtils;
/**
* 微信服务实现
* @author orange
*
*/
@Service
public class WeChatServiceImpl implements WeChatService {
private static final Logger logger = LoggerFactory.getLogger(WeChatServiceImpl.class);
@Autowired
private MiniProgramConfigProperties miniProgramConfigProperties;
@Autowired
private OfficialAccountsConfigProperties officialAccountsConfigProperties;
@Autowired
private TokenMemoryService tokenMemoryService;
@Autowired
@Qualifier(WeChatConstants.REST_TEMPLATE_UTILS_NAME)
private RestTemplateUtils restTemplateUtils;
@Override
public String getAccessToken(AppType type, String source) throws CommonException {
if (type == null) {
throw new CommonException("应用类型为空");
}
if (StringUtils.isBlank(source)) {
throw new CommonException("来源为空");
}
String accessToken = null;
String appId;
String secret;
if (type == AppType.OfficialAccounts) {
OfficialAccountsApplication officialAccountsApplication = ApplicationUtils.getOfficialAccountsApplication(source,
officialAccountsConfigProperties);
appId = officialAccountsApplication.getAppId();
secret = officialAccountsApplication.getSecret();
}
else if (type == AppType.MiniProgram) {
MiniProgramApplication miniProgramApplication = ApplicationUtils.getMiniProgramApplication(source, miniProgramConfigProperties);
appId = miniProgramApplication.getAppId();
secret = miniProgramApplication.getSecret();
}
else {
throw new CommonException("应用类型'" + type.toString() + "'无效");
}
accessToken = tokenMemoryService.get(type, source, appId);
if (StringUtils.isBlank(accessToken)) {
String url = "https://api.weixin.qq.com/cgi-bin/token?grant_type=client_credential&appid=" + appId + "&secret=" + secret;
logger.debug("微信的访问令牌请求地址:" + url);
JSONObject respJson = restTemplateUtils.get(url, null, null, JSONObject.class);
logger.debug("微信的访问令牌返回:" + respJson.toString());
boolean isErrMsg = respJson.containsKey("errmsg");
if (isErrMsg) {
throw new CommonException(
"微信获取访问令牌接口异常,错误码:" + ((String) ReflectUtils.convertValue(ClassType.String, respJson.getIntValue("errcode"))) + ",错误信息:"
+ respJson.getString("errmsg"));
}
accessToken = respJson.getString("access_token");
tokenMemoryService.save(type, source, appId, accessToken, respJson.getLongValue("expires_in"));
}
return accessToken;
}
@Override
public RidInfo getRidInfo(AppType type, String source, String rid) throws CommonException {
if (StringUtils.isBlank(rid)) {
throw new CommonException("Rid号为空");
}
String accessToken = getAccessToken(type, source);
if (StringUtils.isBlank(accessToken)) {
if (type == AppType.MiniProgram) {
throw new CommonException("微信小程序访问令牌为空");
}
else if (type == AppType.OfficialAccounts) {
throw new CommonException("微信公众号访问令牌为空");
}
}
JSONObject requestJson = new JSONObject();
requestJson.put("rid", rid);
String url = "https://api.weixin.qq.com/cgi-bin/openapi/rid/get?access_token=" + accessToken;
logger.debug("查询Rid详情信息接口,地址:" + url + ",请求Json:" + requestJson.toString());
JSONObject resultJson = restTemplateUtils.post(url, MediaType.APPLICATION_JSON, null, requestJson, JSONObject.class);
logger.debug("查询Rid详情信息接口,返回Json:" + resultJson.toString());
int errcode = resultJson.getIntValue("errcode");
if (errcode != 0) {
throw new CommonException("查询Rid详情信息接口异常,错误码:" + resultJson.getIntValue("errcode") + ",错误信息:" + resultJson.getString("errmsg"));
}
JSONObject resultRequestJson = resultJson.getJSONObject("request");
return new RidInfo(new Date(resultRequestJson.getLongValue("invoke_time") * 1000L), resultRequestJson.getLongValue("cost_in_ms"),
resultRequestJson.getString("request_url"), resultRequestJson.getString("request_body"), resultRequestJson.getString("response_body"),
resultRequestJson.getString("client_ip"));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy