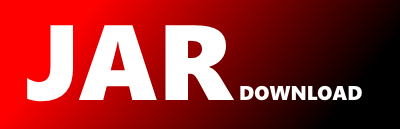
com.gitlab.summercattle.addons.wechat.officialaccounts.service.impl.OfficialAccountsServiceImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cattle-addons-wechat-starter Show documentation
Show all versions of cattle-addons-wechat-starter Show documentation
Cattle Framework Addons WeChat Component
The newest version!
/*
* Copyright (C) 2018 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.gitlab.summercattle.addons.wechat.officialaccounts.service.impl;
import org.apache.commons.lang3.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.http.MediaType;
import org.springframework.stereotype.Service;
import com.alibaba.fastjson2.JSONObject;
import com.gitlab.summercattle.addons.wechat.auth.service.UserService;
import com.gitlab.summercattle.addons.wechat.common.AppType;
import com.gitlab.summercattle.addons.wechat.common.service.WeChatService;
import com.gitlab.summercattle.addons.wechat.constants.WeChatConstants;
import com.gitlab.summercattle.addons.wechat.miniprogram.configure.MiniProgramConfigProperties;
import com.gitlab.summercattle.addons.wechat.miniprogram.configure.MiniProgramApplication;
import com.gitlab.summercattle.addons.wechat.officialaccounts.menu.MenuBar;
import com.gitlab.summercattle.addons.wechat.officialaccounts.menu.match.MatchRule;
import com.gitlab.summercattle.addons.wechat.officialaccounts.message.TemplateMessage;
import com.gitlab.summercattle.addons.wechat.officialaccounts.message.TemplateMessageData;
import com.gitlab.summercattle.addons.wechat.officialaccounts.service.OfficialAccountsService;
import com.gitlab.summercattle.addons.wechat.utils.ApplicationUtils;
import com.gitlab.summercattle.commons.exception.CommonException;
import com.gitlab.summercattle.commons.utils.spring.RestTemplateUtils;
/**
* 公众号服务实现
* @author orange
*
*/
@Service
public class OfficialAccountsServiceImpl implements OfficialAccountsService {
private static final Logger logger = LoggerFactory.getLogger(OfficialAccountsServiceImpl.class);
@Autowired
private WeChatService weChatService;
@Autowired
private UserService userService;
@Autowired
@Qualifier(WeChatConstants.REST_TEMPLATE_UTILS_NAME)
private RestTemplateUtils restTemplateUtils;
@Autowired
private MiniProgramConfigProperties miniProgramConfigProperties;
@Override
public void createMenu(String source, MenuBar menuBar) throws CommonException {
if (StringUtils.isBlank(source)) {
throw new CommonException("来源为空");
}
if (null == menuBar) {
throw new CommonException("一级菜单为空");
}
JSONObject menuJson = menuBar.toJson();
String accessToken = weChatService.getAccessToken(AppType.OfficialAccounts, source);
if (StringUtils.isBlank(accessToken)) {
throw new CommonException("微信公众号访问令牌为空");
}
String url = "https://api.weixin.qq.com/cgi-bin/menu/create?access_token=" + accessToken;
logger.debug("发送微信公众服务号自定义菜单创建接口,地址:" + url + ",请求Json:" + menuJson.toString());
JSONObject resultJson = restTemplateUtils.post(url, MediaType.APPLICATION_JSON, null, menuJson, JSONObject.class);
logger.debug("发送微信公众服务号自定义菜单创建接口,返回Json:" + resultJson.toString());
int errcode = resultJson.getInteger("errcode");
if (errcode != 0) {
throw new CommonException("微信公众服务号自定义菜单创建接口异常,错误码:" + errcode + ",错误信息:" + resultJson.getString("errmsg"));
}
}
@Override
public void deleteMenu(String source) throws CommonException {
if (StringUtils.isBlank(source)) {
throw new CommonException("来源为空");
}
String accessToken = weChatService.getAccessToken(AppType.OfficialAccounts, source);
if (StringUtils.isBlank(accessToken)) {
throw new CommonException("微信公众号访问令牌为空");
}
String url = "https://api.weixin.qq.com/cgi-bin/menu/delete?access_token=" + accessToken;
logger.debug("发送微信公众服务号自定义菜单删除接口,地址:" + url);
JSONObject resultJson = restTemplateUtils.get(url, MediaType.APPLICATION_JSON, null, JSONObject.class);
logger.debug("发送微信公众服务号自定义菜单删除接口,返回Json:" + resultJson.toString());
int errcode = resultJson.getInteger("errcode");
if (errcode != 0) {
throw new CommonException("微信公众服务号自定义菜单删除接口异常,错误码:" + errcode + ",错误信息:" + resultJson.getString("errmsg"));
}
}
@Override
public void createConditionalMenu(String source, String code, MenuBar menuBar, MatchRule[] matchRules) throws CommonException {
}
@Override
public void deleteConditionalMenu(String source, String code) throws CommonException {
}
@Override
public String sendTemplateMessage(String source, int userType, String userInfo, TemplateMessage message) throws CommonException {
String openId = userService.getOpenId(source, AppType.OfficialAccounts, userType, userInfo);
if (StringUtils.isBlank(openId)) {
throw new CommonException("微信公众号没有关注");
}
if (null == message) {
throw new CommonException("微信公众号模板消息为空");
}
if (StringUtils.isBlank(message.getTemplateId())) {
throw new CommonException("微信服务号模板消息的模板Id为空");
}
if (message.getKeywords().size() == 0) {
throw new CommonException("微信公众号模板消息的模板数据为空");
}
if (StringUtils.isNotBlank(message.getMiniProgramPagePath()) && StringUtils.isBlank(message.getMiniProgramSource())) {
throw new CommonException("微信小程序来源为空");
}
String miniProgramAppId = null;
if (StringUtils.isNotBlank(message.getMiniProgramSource())) {
MiniProgramApplication miniProgramApplication = ApplicationUtils.getMiniProgramApplication(source, miniProgramConfigProperties);
miniProgramAppId = miniProgramApplication.getAppId();
}
String accessToken = weChatService.getAccessToken(AppType.OfficialAccounts, source);
if (StringUtils.isBlank(accessToken)) {
throw new CommonException("微信公众号访问令牌为空");
}
JSONObject messageObj = new JSONObject();
messageObj.put("touser", openId);
messageObj.put("template_id", message.getTemplateId());
if (StringUtils.isNotBlank(message.getUrl())) {
messageObj.put("url", message.getUrl());
}
if (StringUtils.isNotBlank(miniProgramAppId)) {
JSONObject minAppObj = new JSONObject();
minAppObj.put("appid", miniProgramAppId);
if (StringUtils.isNotBlank(message.getMiniProgramPagePath())) {
minAppObj.put("pagepath", message.getMiniProgramPagePath());
}
messageObj.put("miniprogram", minAppObj);
}
JSONObject dataObj = new JSONObject();
if (message.getFirst() != null) {
dataObj.put("first", geMessagetData(message.getFirst()));
}
for (int i = 0; i < message.getKeywords().size(); i++) {
dataObj.put("keyword" + String.valueOf(i + 1), geMessagetData(message.getKeywords().get(i)));
}
if (message.getRemark() != null) {
dataObj.put("remark", geMessagetData(message.getRemark()));
}
messageObj.put("data", dataObj);
String url = "https://api.weixin.qq.com/cgi-bin/message/template/send?access_token=" + accessToken;
logger.debug("发送微信公众号模板消息接口,地址:" + url + ",请求Json:" + messageObj.toString());
JSONObject resultJson = restTemplateUtils.post(url, MediaType.APPLICATION_JSON, null, messageObj, JSONObject.class);
logger.debug("发送微信公众号模板消息接口,返回Json:" + resultJson.toString());
int errcode = resultJson.getIntValue("errcode");
if (errcode != 0) {
throw new CommonException("微信公众号模板消息接口异常,错误码:" + errcode + ",错误信息:" + resultJson.getString("errmsg"));
}
return resultJson.getString("msgid");
}
private JSONObject geMessagetData(TemplateMessageData messageData) {
JSONObject dataObj = new JSONObject();
dataObj.put("value", messageData.getValue());
if (StringUtils.isNotBlank(messageData.getColor())) {
dataObj.put("color", messageData.getColor());
}
return dataObj;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy