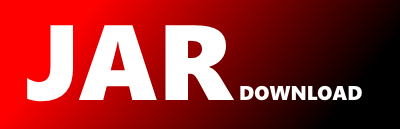
com.gitlab.summercattle.cloud.config.rule.parser.xml.XmlRuleReader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cattle-cloud-config-rule-parser Show documentation
Show all versions of cattle-cloud-config-rule-parser Show documentation
Cattle Framework Cloud Config Rule Parser Component
The newest version!
/*
* Copyright (C) 2018 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.gitlab.summercattle.cloud.config.rule.parser.xml;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import org.apache.commons.lang3.StringUtils;
import org.dom4j.Attribute;
import org.dom4j.Document;
import org.dom4j.Element;
import com.alibaba.fastjson2.JSON;
import com.alibaba.fastjson2.JSONReader.Feature;
import com.alibaba.fastjson2.TypeReference;
import com.gitlab.summercattle.cloud.config.rule.parser.RuleReader;
import com.gitlab.summercattle.cloud.rule.entity.AddressWeightEntity;
import com.gitlab.summercattle.cloud.rule.entity.CountFilterEntity;
import com.gitlab.summercattle.cloud.rule.entity.DiscoveryEntity;
import com.gitlab.summercattle.cloud.rule.entity.FilterHolderEntity;
import com.gitlab.summercattle.cloud.rule.entity.HostFilterEntity;
import com.gitlab.summercattle.cloud.rule.entity.RegionEntity;
import com.gitlab.summercattle.cloud.rule.entity.RegionFilterEntity;
import com.gitlab.summercattle.cloud.rule.entity.RegionWeightEntity;
import com.gitlab.summercattle.cloud.rule.entity.RegisterEntity;
import com.gitlab.summercattle.cloud.rule.entity.RuleEntity;
import com.gitlab.summercattle.cloud.rule.entity.StrategyBlacklistEntity;
import com.gitlab.summercattle.cloud.rule.entity.StrategyConditionBlueGreenEntity;
import com.gitlab.summercattle.cloud.rule.entity.StrategyConditionGrayEntity;
import com.gitlab.summercattle.cloud.rule.entity.StrategyEntity;
import com.gitlab.summercattle.cloud.rule.entity.StrategyFailoverEntity;
import com.gitlab.summercattle.cloud.rule.entity.StrategyHeaderEntity;
import com.gitlab.summercattle.cloud.rule.entity.StrategyReleaseEntity;
import com.gitlab.summercattle.cloud.rule.entity.StrategyRouteEntity;
import com.gitlab.summercattle.cloud.rule.entity.VersionEntity;
import com.gitlab.summercattle.cloud.rule.entity.VersionFilterEntity;
import com.gitlab.summercattle.cloud.rule.entity.VersionWeightEntity;
import com.gitlab.summercattle.cloud.rule.entity.WeightEntity;
import com.gitlab.summercattle.cloud.rule.entity.WeightFilterEntity;
import com.gitlab.summercattle.cloud.rule.entity.constants.ConditionType;
import com.gitlab.summercattle.cloud.rule.entity.constants.FilterType;
import com.gitlab.summercattle.cloud.rule.entity.constants.StrategyRouteType;
import com.gitlab.summercattle.cloud.rule.entity.constants.WeightType;
import com.gitlab.summercattle.cloud.rule.entity.wrapper.WeightEntityWrapper;
import com.gitlab.summercattle.commons.exception.CommonRuntimeException;
import com.gitlab.summercattle.commons.utils.auxiliary.Dom4jUtils;
public class XmlRuleReader implements RuleReader {
@Override
public RuleEntity read(String ruleContent) {
if (org.apache.commons.lang3.StringUtils.isBlank(ruleContent)) {
throw new CommonRuntimeException("规则内容是空的");
}
Document document = Dom4jUtils.getDocument(ruleContent);
Element rootElement = document.getRootElement();
RuleEntity ruleEntity = readRoot(rootElement);
ruleEntity.setContent(ruleContent);
return ruleEntity;
}
private RuleEntity readRoot(Element rootElement) {
if (rootElement.elements(XmlRuleConstants.REGISTER_ELEMENT_NAME).size() > 1) {
throw new CommonRuntimeException("规则内容的要素'" + XmlRuleConstants.REGISTER_ELEMENT_NAME + "'只允许存在一个");
}
if (rootElement.elements(XmlRuleConstants.DISCOVERY_ELEMENT_NAME).size() > 1) {
throw new CommonRuntimeException("规则内容的要素'" + XmlRuleConstants.DISCOVERY_ELEMENT_NAME + "'只允许存在一个");
}
if (rootElement.elements(XmlRuleConstants.STRATEGY_ELEMENT_NAME).size() > 1) {
throw new CommonRuntimeException("规则内容的要素'" + XmlRuleConstants.STRATEGY_ELEMENT_NAME + "'只允许存在一个");
}
if (rootElement.elements(XmlRuleConstants.STRATEGY_RELEASE_ELEMENT_NAME).size() > 1) {
throw new CommonRuntimeException("规则内容的要素'" + XmlRuleConstants.STRATEGY_RELEASE_ELEMENT_NAME + "'只允许存在一个");
}
if (rootElement.elements(XmlRuleConstants.STRATEGY_FAILOVER_ELEMENT_NAME).size() > 1) {
throw new CommonRuntimeException("规则内容的要素'" + XmlRuleConstants.STRATEGY_FAILOVER_ELEMENT_NAME + "'只允许存在一个");
}
if (rootElement.elements(XmlRuleConstants.STRATEGY_BLACKLIST_ELEMENT_NAME).size() > 1) {
throw new CommonRuntimeException("规则内容的要素'" + XmlRuleConstants.STRATEGY_BLACKLIST_ELEMENT_NAME + "'只允许存在一个");
}
RegisterEntity registerEntity = null;
DiscoveryEntity discoveryEntity = null;
StrategyEntity strategyEntity = null;
StrategyReleaseEntity strategyReleaseEntity = null;
StrategyFailoverEntity strategyFailoverEntity = null;
StrategyBlacklistEntity strategyBlacklistEntity = null;
List elements = rootElement.elements();
for (Element element : elements) {
if (org.apache.commons.lang3.StringUtils.equals(element.getName(), XmlRuleConstants.REGISTER_ELEMENT_NAME)) {
registerEntity = new RegisterEntity();
readRegisterEntity(element, registerEntity);
}
else if (org.apache.commons.lang3.StringUtils.equals(element.getName(), XmlRuleConstants.DISCOVERY_ELEMENT_NAME)) {
discoveryEntity = new DiscoveryEntity();
readDiscoveryEntity(element, discoveryEntity);
}
else if (org.apache.commons.lang3.StringUtils.equals(element.getName(), XmlRuleConstants.STRATEGY_ELEMENT_NAME)) {
strategyEntity = new StrategyEntity();
readStrategyEntity(element, strategyEntity);
}
else if (org.apache.commons.lang3.StringUtils.equals(element.getName(), XmlRuleConstants.STRATEGY_RELEASE_ELEMENT_NAME)) {
strategyReleaseEntity = new StrategyReleaseEntity();
readStrategyRelease(element, strategyReleaseEntity);
}
else if (org.apache.commons.lang3.StringUtils.equals(element.getName(), XmlRuleConstants.STRATEGY_FAILOVER_ELEMENT_NAME)) {
strategyFailoverEntity = new StrategyFailoverEntity();
readStrategyFailover(element, strategyFailoverEntity);
}
else if (org.apache.commons.lang3.StringUtils.equals(element.getName(), XmlRuleConstants.STRATEGY_BLACKLIST_ELEMENT_NAME)) {
strategyBlacklistEntity = new StrategyBlacklistEntity();
readStrategyBlacklist(element, strategyBlacklistEntity);
}
}
RuleEntity ruleEntity = new RuleEntity();
ruleEntity.setRegisterEntity(registerEntity);
ruleEntity.setDiscoveryEntity(discoveryEntity);
ruleEntity.setStrategyEntity(strategyEntity);
ruleEntity.setStrategyReleaseEntity(strategyReleaseEntity);
ruleEntity.setStrategyFailoverEntity(strategyFailoverEntity);
ruleEntity.setStrategyBlacklistEntity(strategyBlacklistEntity);
return ruleEntity;
}
private void readRegisterEntity(Element registerElement, RegisterEntity registerEntity) {
List elements = registerElement.elements();
for (Element element : elements) {
if (org.apache.commons.lang3.StringUtils.equals(element.getName(), XmlRuleConstants.WHITELIST_ELEMENT_NAME)) {
readHostFilter(element, FilterType.WHITELIST, registerEntity);
}
else if (org.apache.commons.lang3.StringUtils.equals(element.getName(), XmlRuleConstants.BLACKLIST_ELEMENT_NAME)) {
readHostFilter(element, FilterType.BLACKLIST, registerEntity);
}
else if (org.apache.commons.lang3.StringUtils.equals(element.getName(), XmlRuleConstants.COUNT_ELEMENT_NAME)) {
readCountFilter(element, registerEntity);
}
}
}
private void readCountFilter(Element countFilterElement, RegisterEntity registerEntity) {
CountFilterEntity countFilterEntity = registerEntity.getCountFilterEntity();
if (countFilterEntity != null) {
throw new CommonRuntimeException("规则内容的要素'" + XmlRuleConstants.COUNT_ELEMENT_NAME + "',只允许存在一个");
}
countFilterEntity = new CountFilterEntity();
Attribute valueAttribute = countFilterElement.attribute(XmlRuleConstants.VALUE_ATTRIBUTE_NAME);
if (valueAttribute != null) {
String valueAttributeStr = valueAttribute.getData().toString().trim();
if (org.apache.commons.lang3.StringUtils.isNotBlank(valueAttributeStr)) {
Integer value;
try {
value = Integer.valueOf(valueAttributeStr);
}
catch (final NumberFormatException e) {
throw new CommonRuntimeException(
"规则内容中要素'" + countFilterElement.getName() + "'的属性'" + XmlRuleConstants.VALUE_ATTRIBUTE_NAME + "'值无效,必须是整数类型", e);
}
countFilterEntity.setFilterValue(value);
}
}
Map filterMap = countFilterEntity.getFilterMap();
List elements = countFilterElement.elements();
for (Element element : elements) {
if (org.apache.commons.lang3.StringUtils.equals(element.getName(), XmlRuleConstants.SERVICE_ELEMENT_NAME)) {
Attribute nameAttribute = element.attribute(XmlRuleConstants.NAME_ATTRIBUTE_NAME);
if (nameAttribute == null) {
throw new CommonRuntimeException("规则内容中要素'" + element.getName() + "'的属性'" + XmlRuleConstants.NAME_ATTRIBUTE_NAME + "'缺失");
}
String serviceName = nameAttribute.getData().toString().trim();
Integer value = null;
Attribute serviceValueAttribute = element.attribute(XmlRuleConstants.VALUE_ATTRIBUTE_NAME);
if (valueAttribute != null) {
String valueAttributeStr = serviceValueAttribute.getData().toString().trim();
if (org.apache.commons.lang3.StringUtils.isNotBlank(valueAttributeStr)) {
try {
value = Integer.valueOf(valueAttributeStr);
}
catch (NumberFormatException e) {
throw new CommonRuntimeException(
"规则内容中要素'" + element.getName() + "'的属性'" + XmlRuleConstants.VALUE_ATTRIBUTE_NAME + "'值无效,必须是整数类型", e);
}
}
}
filterMap.put(serviceName, value);
}
}
registerEntity.setCountFilterEntity(countFilterEntity);
}
private void readHostFilter(Element hostFilterElement, FilterType filterType, FilterHolderEntity filterHolderEntity) {
HostFilterEntity hostFilterEntity = filterHolderEntity.getHostFilterEntity();
if (hostFilterEntity != null) {
String elementName;
if (filterType == FilterType.WHITELIST) {
elementName = XmlRuleConstants.WHITELIST_ELEMENT_NAME;
}
else if (filterType == FilterType.BLACKLIST) {
elementName = XmlRuleConstants.BLACKLIST_ELEMENT_NAME;
}
else {
throw new CommonRuntimeException("过滤类型'" + filterType.toString() + "'未知");
}
throw new CommonRuntimeException("规则内容的过滤要素'" + elementName + "',只允许存在一个");
}
hostFilterEntity = new HostFilterEntity();
hostFilterEntity.setFilterType(filterType);
Attribute valueAttribute = hostFilterElement.attribute(XmlRuleConstants.VALUE_ATTRIBUTE_NAME);
if (valueAttribute != null) {
String valueAttributeStr = valueAttribute.getData().toString().trim();
List filterValueList = com.gitlab.summercattle.cloud.utils.StringUtils.splitToList(valueAttributeStr);
hostFilterEntity.setFilterValueList(filterValueList);
}
Map> filterMap = hostFilterEntity.getFilterMap();
List elements = hostFilterElement.elements();
for (Element element : elements) {
if (org.apache.commons.lang3.StringUtils.equals(element.getName(), XmlRuleConstants.SERVICE_ELEMENT_NAME)) {
Attribute nameAttribute = element.attribute(XmlRuleConstants.NAME_ATTRIBUTE_NAME);
if (nameAttribute == null) {
throw new CommonRuntimeException("规则内容中要素'" + element.getName() + "'的属性'" + XmlRuleConstants.NAME_ATTRIBUTE_NAME + "'缺失");
}
String serviceName = nameAttribute.getData().toString().trim();
Attribute serviceValueAttribute = element.attribute(XmlRuleConstants.VALUE_ATTRIBUTE_NAME);
List filterValueList = null;
if (serviceValueAttribute != null) {
String filterValueStr = serviceValueAttribute.getData().toString().trim();
filterValueList = com.gitlab.summercattle.cloud.utils.StringUtils.splitToList(filterValueStr);
}
filterMap.put(serviceName, filterValueList);
}
}
filterHolderEntity.setHostFilterEntity(hostFilterEntity);
}
private void readDiscoveryEntity(Element discoveryElement, DiscoveryEntity discoveryEntity) {
List elements = discoveryElement.elements();
for (Element element : elements) {
if (org.apache.commons.lang3.StringUtils.equals(element.getName(), XmlRuleConstants.WHITELIST_ELEMENT_NAME)) {
readHostFilter(element, FilterType.WHITELIST, discoveryEntity);
}
else if (org.apache.commons.lang3.StringUtils.equals(element.getName(), XmlRuleConstants.BLACKLIST_ELEMENT_NAME)) {
readHostFilter(element, FilterType.BLACKLIST, discoveryEntity);
}
else if (org.apache.commons.lang3.StringUtils.equals(element.getName(), XmlRuleConstants.VERSION_ELEMENT_NAME)) {
readVersionFilter(element, discoveryEntity);
}
else if (org.apache.commons.lang3.StringUtils.equals(element.getName(), XmlRuleConstants.REGION_ELEMENT_NAME)) {
readRegionFilter(element, discoveryEntity);
}
else if (org.apache.commons.lang3.StringUtils.equals(element.getName(), XmlRuleConstants.WEIGHT_ELEMENT_NAME)) {
readWeightFilter(element, discoveryEntity);
}
}
}
private void readVersionFilter(Element versionFilterElement, DiscoveryEntity discoveryEntity) {
VersionFilterEntity versionFilterEntity = discoveryEntity.getVersionFilterEntity();
if (versionFilterEntity != null) {
throw new CommonRuntimeException("规则内容的要素'" + XmlRuleConstants.VERSION_ELEMENT_NAME + "',只允许存在一个");
}
versionFilterEntity = new VersionFilterEntity();
Map> versionEntityMap = versionFilterEntity.getVersionEntityMap();
List elements = versionFilterElement.elements();
for (Element element : elements) {
if (StringUtils.equals(element.getName(), XmlRuleConstants.SERVICE_ELEMENT_NAME)) {
VersionEntity versionEntity = new VersionEntity();
Attribute consumerServiceNameAttribute = element.attribute(XmlRuleConstants.CONSUMER_SERVICE_NAME_ATTRIBUTE_NAME);
if (consumerServiceNameAttribute == null) {
throw new CommonRuntimeException(
"规则内容中要素'" + element.getName() + "'的属性'" + XmlRuleConstants.CONSUMER_SERVICE_NAME_ATTRIBUTE_NAME + "'缺失");
}
String consumerServiceName = consumerServiceNameAttribute.getData().toString().trim();
versionEntity.setConsumerServiceName(consumerServiceName);
Attribute providerServiceNameAttribute = element.attribute(XmlRuleConstants.PROVIDER_SERVICE_NAME_ATTRIBUTE_NAME);
if (providerServiceNameAttribute == null) {
throw new CommonRuntimeException(
"规则内容中要素'" + element.getName() + "'的属性'" + XmlRuleConstants.PROVIDER_SERVICE_NAME_ATTRIBUTE_NAME + "'缺失");
}
String providerServiceName = providerServiceNameAttribute.getData().toString().trim();
versionEntity.setProviderServiceName(providerServiceName);
Attribute consumerVersionValueAttribute = element.attribute(XmlRuleConstants.CONSUMER_VERSION_VALUE_ATTRIBUTE_NAME);
if (consumerVersionValueAttribute != null) {
String consumerVersionValue = consumerVersionValueAttribute.getData().toString().trim();
List consumerVersionValueList = com.gitlab.summercattle.cloud.utils.StringUtils.splitToList(consumerVersionValue);
versionEntity.setConsumerVersionValueList(consumerVersionValueList);
}
Attribute providerVersionValueAttribute = element.attribute(XmlRuleConstants.PROVIDER_VERSION_VALUE_ATTRIBUTE_NAME);
if (providerVersionValueAttribute != null) {
String providerVersionValue = providerVersionValueAttribute.getData().toString().trim();
List providerVersionValueList = com.gitlab.summercattle.cloud.utils.StringUtils.splitToList(providerVersionValue);
versionEntity.setProviderVersionValueList(providerVersionValueList);
}
List versionEntityList = versionEntityMap.get(consumerServiceName);
if (versionEntityList == null) {
versionEntityList = new ArrayList();
versionEntityMap.put(consumerServiceName, versionEntityList);
}
versionEntityList.add(versionEntity);
}
}
discoveryEntity.setVersionFilterEntity(versionFilterEntity);
}
private void readRegionFilter(Element regionFilterElement, DiscoveryEntity discoveryEntity) {
RegionFilterEntity regionFilterEntity = discoveryEntity.getRegionFilterEntity();
if (regionFilterEntity != null) {
throw new CommonRuntimeException("规则内容的要素'" + XmlRuleConstants.REGION_ELEMENT_NAME + "',只允许存在一个");
}
regionFilterEntity = new RegionFilterEntity();
Map> regionEntityMap = regionFilterEntity.getRegionEntityMap();
List elements = regionFilterElement.elements();
for (Element element : elements) {
if (StringUtils.equals(element.getName(), XmlRuleConstants.SERVICE_ELEMENT_NAME)) {
RegionEntity regionEntity = new RegionEntity();
Attribute consumerServiceNameAttribute = element.attribute(XmlRuleConstants.CONSUMER_SERVICE_NAME_ATTRIBUTE_NAME);
if (consumerServiceNameAttribute == null) {
throw new CommonRuntimeException(
"规则内容中要素'" + element.getName() + "'的属性'" + XmlRuleConstants.CONSUMER_SERVICE_NAME_ATTRIBUTE_NAME + "'缺失");
}
String consumerServiceName = consumerServiceNameAttribute.getData().toString().trim();
regionEntity.setConsumerServiceName(consumerServiceName);
Attribute providerServiceNameAttribute = element.attribute(XmlRuleConstants.PROVIDER_SERVICE_NAME_ATTRIBUTE_NAME);
if (providerServiceNameAttribute == null) {
throw new CommonRuntimeException(
"规则内容中要素'" + element.getName() + "'的属性'" + XmlRuleConstants.PROVIDER_SERVICE_NAME_ATTRIBUTE_NAME + "'缺失");
}
String providerServiceName = providerServiceNameAttribute.getData().toString().trim();
regionEntity.setProviderServiceName(providerServiceName);
Attribute consumerRegionValueAttribute = element.attribute(XmlRuleConstants.CONSUMER_REGION_VALUE_ATTRIBUTE_NAME);
if (consumerRegionValueAttribute != null) {
String consumerRegionValue = consumerRegionValueAttribute.getData().toString().trim();
List consumerRegionValueList = com.gitlab.summercattle.cloud.utils.StringUtils.splitToList(consumerRegionValue);
regionEntity.setConsumerRegionValueList(consumerRegionValueList);
}
Attribute providerRegionValueAttribute = element.attribute(XmlRuleConstants.PROVIDER_REGION_VALUE_ATTRIBUTE_NAME);
if (providerRegionValueAttribute != null) {
String providerRegionValue = providerRegionValueAttribute.getData().toString().trim();
List providerRegionValueList = com.gitlab.summercattle.cloud.utils.StringUtils.splitToList(providerRegionValue);
regionEntity.setProviderRegionValueList(providerRegionValueList);
}
List regionEntityList = regionEntityMap.get(consumerServiceName);
if (regionEntityList == null) {
regionEntityList = new ArrayList();
regionEntityMap.put(consumerServiceName, regionEntityList);
}
regionEntityList.add(regionEntity);
}
}
discoveryEntity.setRegionFilterEntity(regionFilterEntity);
}
private void readWeightFilter(Element weightFilterElement, DiscoveryEntity discoveryEntity) {
WeightFilterEntity weightFilterEntity = discoveryEntity.getWeightFilterEntity();
if (weightFilterEntity != null) {
throw new CommonRuntimeException("规则内容的要素'" + XmlRuleConstants.WEIGHT_ELEMENT_NAME + "',只允许存在一个");
}
weightFilterEntity = new WeightFilterEntity();
Map> versionWeightEntityMap = new LinkedHashMap>();
List versionWeightEntityList = new ArrayList();
weightFilterEntity.setVersionWeightEntityMap(versionWeightEntityMap);
weightFilterEntity.setVersionWeightEntityList(versionWeightEntityList);
Map> regionWeightEntityMap = new LinkedHashMap>();
List regionWeightEntityList = new ArrayList();
weightFilterEntity.setRegionWeightEntityMap(regionWeightEntityMap);
weightFilterEntity.setRegionWeightEntityList(regionWeightEntityList);
List elements = weightFilterElement.elements();
for (Element element : elements) {
if (StringUtils.equals(element.getName(), XmlRuleConstants.SERVICE_ELEMENT_NAME)) {
WeightEntity weightEntity = new WeightEntity();
Attribute typeAttribute = element.attribute(XmlRuleConstants.TYPE_ATTRIBUTE_NAME);
if (typeAttribute == null) {
throw new CommonRuntimeException("规则内容中要素'" + element.getName() + "'的属性'" + XmlRuleConstants.TYPE_ATTRIBUTE_NAME + "'缺失");
}
String type = typeAttribute.getData().toString().trim();
WeightType weightType = WeightType.parse(type);
weightEntity.setType(weightType);
Attribute consumerServiceNameAttribute = element.attribute(XmlRuleConstants.CONSUMER_SERVICE_NAME_ATTRIBUTE_NAME);
String consumerServiceName = null;
if (consumerServiceNameAttribute != null) {
consumerServiceName = consumerServiceNameAttribute.getData().toString().trim();
}
weightEntity.setConsumerServiceName(consumerServiceName);
Attribute providerServiceNameAttribute = element.attribute(XmlRuleConstants.PROVIDER_SERVICE_NAME_ATTRIBUTE_NAME);
if (providerServiceNameAttribute == null) {
throw new CommonRuntimeException(
"规则内容中要素'" + element.getName() + "'的属性'" + XmlRuleConstants.PROVIDER_SERVICE_NAME_ATTRIBUTE_NAME + "'缺失");
}
String providerServiceName = providerServiceNameAttribute.getData().toString().trim();
weightEntity.setProviderServiceName(providerServiceName);
Attribute providerWeightValueAttribute = element.attribute(XmlRuleConstants.PROVIDER_WEIGHT_VALUE_ATTRIBUTE_NAME);
if (providerWeightValueAttribute == null) {
throw new CommonRuntimeException(
"规则内容中要素'" + element.getName() + "'的属性'" + XmlRuleConstants.PROVIDER_WEIGHT_VALUE_ATTRIBUTE_NAME + "'缺失");
}
String providerWeightValue = providerWeightValueAttribute.getData().toString().trim();
WeightEntityWrapper.readWeightEntity(weightEntity, providerWeightValue);
if (StringUtils.isNotEmpty(consumerServiceName)) {
if (weightType == WeightType.VERSION) {
List list = versionWeightEntityMap.get(consumerServiceName);
if (list == null) {
list = new ArrayList();
versionWeightEntityMap.put(consumerServiceName, list);
}
list.add(weightEntity);
}
else if (weightType == WeightType.REGION) {
List list = regionWeightEntityMap.get(consumerServiceName);
if (list == null) {
list = new ArrayList();
regionWeightEntityMap.put(consumerServiceName, list);
}
list.add(weightEntity);
}
}
else {
if (weightType == WeightType.VERSION) {
versionWeightEntityList.add(weightEntity);
}
else if (weightType == WeightType.REGION) {
regionWeightEntityList.add(weightEntity);
}
}
}
else if (StringUtils.equals(element.getName(), XmlRuleConstants.VERSION_ELEMENT_NAME)) {
VersionWeightEntity versionWeightEntity = weightFilterEntity.getVersionWeightEntity();
if (versionWeightEntity != null) {
throw new CommonRuntimeException("规则内容的要素'" + XmlRuleConstants.VERSION_ELEMENT_NAME + "',只允许存在一个");
}
versionWeightEntity = new VersionWeightEntity();
Attribute providerWeightValueAttribute = element.attribute(XmlRuleConstants.PROVIDER_WEIGHT_VALUE_ATTRIBUTE_NAME);
if (providerWeightValueAttribute == null) {
throw new CommonRuntimeException(
"规则内容中要素'" + element.getName() + "'的属性'" + XmlRuleConstants.PROVIDER_WEIGHT_VALUE_ATTRIBUTE_NAME + "'缺失");
}
String providerWeightValue = providerWeightValueAttribute.getData().toString().trim();
WeightEntityWrapper.readWeightEntity(versionWeightEntity, providerWeightValue);
weightFilterEntity.setVersionWeightEntity(versionWeightEntity);
}
else if (StringUtils.equals(element.getName(), XmlRuleConstants.REGION_ELEMENT_NAME)) {
RegionWeightEntity regionWeightEntity = weightFilterEntity.getRegionWeightEntity();
if (regionWeightEntity != null) {
throw new CommonRuntimeException("规则内容的要素'" + XmlRuleConstants.REGION_ELEMENT_NAME + "',只允许存在一个");
}
regionWeightEntity = new RegionWeightEntity();
Attribute providerWeightValueAttribute = element.attribute(XmlRuleConstants.PROVIDER_WEIGHT_VALUE_ATTRIBUTE_NAME);
if (providerWeightValueAttribute == null) {
throw new CommonRuntimeException(
"规则内容中要素'" + element.getName() + "'的属性'" + XmlRuleConstants.PROVIDER_WEIGHT_VALUE_ATTRIBUTE_NAME + "'缺失");
}
String providerWeightValue = providerWeightValueAttribute.getData().toString().trim();
WeightEntityWrapper.readWeightEntity(regionWeightEntity, providerWeightValue);
weightFilterEntity.setRegionWeightEntity(regionWeightEntity);
}
}
discoveryEntity.setWeightFilterEntity(weightFilterEntity);
}
private void readStrategyEntity(Element strategyEntityElement, StrategyEntity strategyEntity) {
int versionElementCount = strategyEntityElement.elements(XmlRuleConstants.VERSION_ELEMENT_NAME).size();
if (versionElementCount > 1) {
throw new CommonRuntimeException("规则内容的要素'" + XmlRuleConstants.VERSION_ELEMENT_NAME + "',只允许存在一个");
}
int regionElementCount = strategyEntityElement.elements(XmlRuleConstants.REGION_ELEMENT_NAME).size();
if (regionElementCount > 1) {
throw new CommonRuntimeException("规则内容的要素'" + XmlRuleConstants.REGION_ELEMENT_NAME + "',只允许存在一个");
}
int addressElementCount = strategyEntityElement.elements(XmlRuleConstants.ADDRESS_ELEMENT_NAME).size();
if (addressElementCount > 1) {
throw new CommonRuntimeException("规则内容的要素'" + XmlRuleConstants.ADDRESS_ELEMENT_NAME + "',只允许存在一个");
}
int versionWeightElementCount = strategyEntityElement.elements(XmlRuleConstants.VERSION_WEIGHT_ELEMENT_NAME).size();
if (versionWeightElementCount > 1) {
throw new CommonRuntimeException("规则内容的要素'" + XmlRuleConstants.VERSION_WEIGHT_ELEMENT_NAME + "',只允许存在一个");
}
int regionWeightElementCount = strategyEntityElement.elements(XmlRuleConstants.REGION_WEIGHT_ELEMENT_NAME).size();
if (regionWeightElementCount > 1) {
throw new CommonRuntimeException("规则内容的要素'" + XmlRuleConstants.REGION_WEIGHT_ELEMENT_NAME + "',只允许存在一个");
}
List elements = strategyEntityElement.elements();
for (Element element : elements) {
if (StringUtils.equals(element.getName(), XmlRuleConstants.VERSION_ELEMENT_NAME)) {
String versionValue = element.getTextTrim();
strategyEntity.setVersionValue(versionValue);
}
else if (StringUtils.equals(element.getName(), XmlRuleConstants.REGION_ELEMENT_NAME)) {
String regionValue = element.getTextTrim();
strategyEntity.setRegionValue(regionValue);
}
else if (StringUtils.equals(element.getName(), XmlRuleConstants.ADDRESS_ELEMENT_NAME)) {
String addressValue = element.getTextTrim();
strategyEntity.setAddressValue(addressValue);
}
else if (StringUtils.equals(element.getName(), XmlRuleConstants.VERSION_WEIGHT_ELEMENT_NAME)) {
String versionWeightValue = element.getTextTrim();
strategyEntity.setVersionWeightValue(versionWeightValue);
}
else if (StringUtils.equals(element.getName(), XmlRuleConstants.REGION_WEIGHT_ELEMENT_NAME)) {
String regionWeightValue = element.getTextTrim();
strategyEntity.setRegionWeightValue(regionWeightValue);
}
}
}
private void readStrategyRelease(Element strategyReleaseElement, StrategyReleaseEntity strategyReleaseEntity) {
List elements = strategyReleaseElement.elements();
for (Element element : elements) {
if (StringUtils.equals(element.getName(), XmlRuleConstants.CONDITIONS_ELEMENT_NAME)) {
Attribute typeAttribute = element.attribute(XmlRuleConstants.TYPE_ATTRIBUTE_NAME);
if (typeAttribute == null) {
throw new CommonRuntimeException("规则内容中要素'" + element.getName() + "'的属性'" + XmlRuleConstants.TYPE_ATTRIBUTE_NAME + "'缺失");
}
String type = typeAttribute.getData().toString().trim();
ConditionType conditionType = ConditionType.parse(type);
switch (conditionType) {
case BLUE_GREEN:
readStrategyConditionBlueGreen(element, strategyReleaseEntity);
break;
case GRAY:
readStrategyConditionGray(element, strategyReleaseEntity);
break;
}
}
else if (StringUtils.equals(element.getName(), XmlRuleConstants.ROUTES_ELEMENT_NAME)) {
readStrategyRoute(element, strategyReleaseEntity);
}
else if (StringUtils.equals(element.getName(), XmlRuleConstants.HEADER_ELEMENT_NAME)) {
readStrategyHeader(element, strategyReleaseEntity);
}
}
}
private void readStrategyConditionGray(Element strategyReleaseItemElement, StrategyReleaseEntity strategyReleaseEntity) {
List strategyConditionGrayEntityList = strategyReleaseEntity.getStrategyConditionGrayEntityList();
if (strategyConditionGrayEntityList != null) {
throw new CommonRuntimeException("规则内容中要素'" + XmlRuleConstants.REGION_WEIGHT_ELEMENT_NAME + "'的属性'" + XmlRuleConstants.TYPE_ATTRIBUTE_NAME
+ "'值'" + ConditionType.GRAY.toString() + "'只允许存在一个");
}
strategyConditionGrayEntityList = new ArrayList();
strategyReleaseEntity.setStrategyConditionGrayEntityList(strategyConditionGrayEntityList);
List elements = strategyReleaseItemElement.elements();
for (Element element : elements) {
if (StringUtils.equals(element.getName(), XmlRuleConstants.CONDITION_ELEMENT_NAME)) {
StrategyConditionGrayEntity strategyConditionGrayEntity = new StrategyConditionGrayEntity();
Attribute idAttribute = element.attribute(XmlRuleConstants.ID_ATTRIBUTE_NAME);
if (idAttribute == null) {
throw new CommonRuntimeException("规则内容中要素'" + element.getName() + "'的属性'" + XmlRuleConstants.ID_ATTRIBUTE_NAME + "'缺失");
}
String id = idAttribute.getData().toString().trim();
strategyConditionGrayEntity.setId(id);
Attribute expressionAttribute = element.attribute(XmlRuleConstants.EXPRESSION_ATTRIBUTE_NAME);
Attribute headerAttribute = element.attribute(XmlRuleConstants.HEADER_ATTRIBUTE_NAME);
if (expressionAttribute != null && headerAttribute != null) {
throw new CommonRuntimeException("规则内容中要素'" + element.getName() + "'的属性'" + XmlRuleConstants.EXPRESSION_ATTRIBUTE_NAME + "'和'"
+ XmlRuleConstants.HEADER_ATTRIBUTE_NAME + "'已经被全部配置,它们各项只允许存在一个");
}
if (expressionAttribute != null) {
String expression = expressionAttribute.getData().toString().trim();
strategyConditionGrayEntity.setExpression(expression);
}
if (headerAttribute != null) {
String expression = headerAttribute.getData().toString().trim();
strategyConditionGrayEntity.setExpression(expression);
}
Attribute versionIdAttribute = element
.attribute(XmlRuleConstants.VERSION_ELEMENT_NAME + XmlRuleConstants.DASH + XmlRuleConstants.ID_ATTRIBUTE_NAME);
if (versionIdAttribute != null) {
String versionId = versionIdAttribute.getData().toString().trim();
VersionWeightEntity versionWeightEntity = new VersionWeightEntity();
WeightEntityWrapper.readWeightEntity(versionWeightEntity, versionId);
strategyConditionGrayEntity.setVersionWeightEntity(versionWeightEntity);
}
Attribute regionIdAttribute = element
.attribute(XmlRuleConstants.REGION_ELEMENT_NAME + XmlRuleConstants.DASH + XmlRuleConstants.ID_ATTRIBUTE_NAME);
if (regionIdAttribute != null) {
String regionId = regionIdAttribute.getData().toString().trim();
RegionWeightEntity regionWeightEntity = new RegionWeightEntity();
WeightEntityWrapper.readWeightEntity(regionWeightEntity, regionId);
strategyConditionGrayEntity.setRegionWeightEntity(regionWeightEntity);
}
Attribute addressIdAttribute = element
.attribute(XmlRuleConstants.ADDRESS_ELEMENT_NAME + XmlRuleConstants.DASH + XmlRuleConstants.ID_ATTRIBUTE_NAME);
if (addressIdAttribute != null) {
String addressId = addressIdAttribute.getData().toString().trim();
AddressWeightEntity addressWeightEntity = new AddressWeightEntity();
WeightEntityWrapper.readWeightEntity(addressWeightEntity, addressId);
strategyConditionGrayEntity.setAddressWeightEntity(addressWeightEntity);
}
strategyConditionGrayEntityList.add(strategyConditionGrayEntity);
}
}
}
private void readStrategyConditionBlueGreen(Element strategyReleaseItemElement, StrategyReleaseEntity strategyReleaseEntity) {
List strategyConditionBlueGreenEntityList = strategyReleaseEntity.getStrategyConditionBlueGreenEntityList();
if (strategyConditionBlueGreenEntityList != null) {
throw new CommonRuntimeException("规则内容中要素'" + XmlRuleConstants.CONDITIONS_ELEMENT_NAME + "'的属性'" + XmlRuleConstants.TYPE_ATTRIBUTE_NAME
+ "'值'" + ConditionType.BLUE_GREEN.toString() + "'只允许存在一个");
}
strategyConditionBlueGreenEntityList = new ArrayList();
strategyReleaseEntity.setStrategyConditionBlueGreenEntityList(strategyConditionBlueGreenEntityList);
List elements = strategyReleaseItemElement.elements();
for (Element element : elements) {
if (StringUtils.equals(element.getName(), XmlRuleConstants.CONDITION_ELEMENT_NAME)) {
StrategyConditionBlueGreenEntity strategyConditionBlueGreenEntity = new StrategyConditionBlueGreenEntity();
Attribute idAttribute = element.attribute(XmlRuleConstants.ID_ATTRIBUTE_NAME);
if (idAttribute == null) {
throw new CommonRuntimeException("规则内容中要素'" + element.getName() + "'的属性'" + XmlRuleConstants.ID_ATTRIBUTE_NAME + "'缺失");
}
String id = idAttribute.getData().toString().trim();
strategyConditionBlueGreenEntity.setId(id);
Attribute expressionAttribute = element.attribute(XmlRuleConstants.EXPRESSION_ATTRIBUTE_NAME);
Attribute headerAttribute = element.attribute(XmlRuleConstants.HEADER_ATTRIBUTE_NAME);
if (expressionAttribute != null && headerAttribute != null) {
throw new CommonRuntimeException("规则内容中要素'" + element.getName() + "'的属性'" + XmlRuleConstants.EXPRESSION_ATTRIBUTE_NAME + "'和'"
+ XmlRuleConstants.HEADER_ATTRIBUTE_NAME + "'已经被全部配置,它们各项只允许存在一个");
}
if (expressionAttribute != null) {
String expression = expressionAttribute.getData().toString().trim();
strategyConditionBlueGreenEntity.setExpression(expression);
}
if (headerAttribute != null) {
String expression = headerAttribute.getData().toString().trim();
strategyConditionBlueGreenEntity.setExpression(expression);
}
Attribute versionIdAttribute = element
.attribute(XmlRuleConstants.VERSION_ELEMENT_NAME + XmlRuleConstants.DASH + XmlRuleConstants.ID_ATTRIBUTE_NAME);
if (versionIdAttribute != null) {
String versionId = versionIdAttribute.getData().toString().trim();
strategyConditionBlueGreenEntity.setVersionId(versionId);
}
Attribute regionIdAttribute = element
.attribute(XmlRuleConstants.REGION_ELEMENT_NAME + XmlRuleConstants.DASH + XmlRuleConstants.ID_ATTRIBUTE_NAME);
if (regionIdAttribute != null) {
String regionId = regionIdAttribute.getData().toString().trim();
strategyConditionBlueGreenEntity.setRegionId(regionId);
}
Attribute addressIdAttribute = element
.attribute(XmlRuleConstants.ADDRESS_ELEMENT_NAME + XmlRuleConstants.DASH + XmlRuleConstants.ID_ATTRIBUTE_NAME);
if (addressIdAttribute != null) {
String addressId = addressIdAttribute.getData().toString().trim();
strategyConditionBlueGreenEntity.setAddressId(addressId);
}
Attribute versionWeightIdAttribute = element
.attribute(XmlRuleConstants.VERSION_WEIGHT_ELEMENT_NAME + XmlRuleConstants.DASH + XmlRuleConstants.ID_ATTRIBUTE_NAME);
if (versionWeightIdAttribute != null) {
String versionWeightId = versionWeightIdAttribute.getData().toString().trim();
strategyConditionBlueGreenEntity.setVersionWeightId(versionWeightId);
}
Attribute regionWeightIdAttribute = element
.attribute(XmlRuleConstants.REGION_WEIGHT_ELEMENT_NAME + XmlRuleConstants.DASH + XmlRuleConstants.ID_ATTRIBUTE_NAME);
if (regionWeightIdAttribute != null) {
String regionWeightId = regionWeightIdAttribute.getData().toString().trim();
strategyConditionBlueGreenEntity.setRegionWeightId(regionWeightId);
}
strategyConditionBlueGreenEntityList.add(strategyConditionBlueGreenEntity);
}
}
}
private void readStrategyBlacklist(Element strategyBlacklistElement, StrategyBlacklistEntity strategyBlacklistEntity) {
int addressElementCount = strategyBlacklistElement.elements(XmlRuleConstants.ADDRESS_ELEMENT_NAME).size();
if (addressElementCount > 1) {
throw new CommonRuntimeException("规则内容的要素'" + XmlRuleConstants.ADDRESS_ELEMENT_NAME + "',只允许存在一个");
}
List elements = strategyBlacklistElement.elements();
for (Element element : elements) {
if (StringUtils.equals(element.getName(), XmlRuleConstants.ADDRESS_ELEMENT_NAME)) {
String addressValue = element.getTextTrim();
strategyBlacklistEntity.setAddressValue(addressValue);
}
}
}
private void readStrategyRoute(Element strategyRouteElement, StrategyReleaseEntity strategyReleaseEntity) {
List strategyRouteEntityList = strategyReleaseEntity.getStrategyRouteEntityList();
if (strategyRouteEntityList != null) {
throw new CommonRuntimeException("规则内容的要素'" + XmlRuleConstants.ROUTES_ELEMENT_NAME + "',只允许存在一个");
}
strategyRouteEntityList = new ArrayList();
strategyReleaseEntity.setStrategyRouteEntityList(strategyRouteEntityList);
List elements = strategyRouteElement.elements();
for (Element element : elements) {
if (StringUtils.equals(element.getName(), XmlRuleConstants.ROUTE_ELEMENT_NAME)) {
StrategyRouteEntity strategyRouteEntity = new StrategyRouteEntity();
Attribute idAttribute = element.attribute(XmlRuleConstants.ID_ATTRIBUTE_NAME);
if (idAttribute == null) {
throw new CommonRuntimeException("规则内容中要素'" + element.getName() + "'的属性'" + XmlRuleConstants.ID_ATTRIBUTE_NAME + "'缺失");
}
String id = idAttribute.getData().toString().trim();
strategyRouteEntity.setId(id);
Attribute typeAttribute = element.attribute(XmlRuleConstants.TYPE_ATTRIBUTE_NAME);
if (typeAttribute == null) {
throw new CommonRuntimeException("规则内容中要素'" + element.getName() + "'的属性'" + XmlRuleConstants.TYPE_ATTRIBUTE_NAME + "'缺失");
}
String type = typeAttribute.getData().toString().trim();
StrategyRouteType strategyRouteType = StrategyRouteType.parse(type);
strategyRouteEntity.setType(strategyRouteType);
String value = element.getTextTrim();
strategyRouteEntity.setValue(value);
strategyRouteEntityList.add(strategyRouteEntity);
}
}
}
private void readStrategyHeader(Element strategyHeaderElement, StrategyReleaseEntity strategyReleaseEntity) {
StrategyHeaderEntity strategyHeaderEntity = strategyReleaseEntity.getStrategyHeaderEntity();
if (strategyHeaderEntity != null) {
throw new CommonRuntimeException("规则内容的要素'" + XmlRuleConstants.HEADER_ELEMENT_NAME + "'只允许存在一个");
}
strategyHeaderEntity = new StrategyHeaderEntity();
strategyReleaseEntity.setStrategyHeaderEntity(strategyHeaderEntity);
String headerValue = strategyHeaderElement.getTextTrim();
Map headerMap = JSON.parseObject(headerValue, new TypeReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy