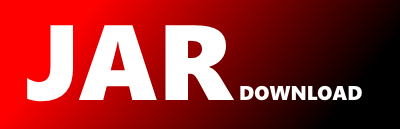
com.gitlab.summercattle.commons.security.crypto.CommonEncryptUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cattle-commons-security Show documentation
Show all versions of cattle-commons-security Show documentation
Cattle Framework Commons Security Component
The newest version!
/*
* Copyright (C) 2018 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.gitlab.summercattle.commons.security.crypto;
import java.security.AlgorithmParameters;
import java.security.InvalidAlgorithmParameterException;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import java.security.Provider;
import java.security.SecureRandom;
import java.security.spec.InvalidParameterSpecException;
import javax.crypto.BadPaddingException;
import javax.crypto.Cipher;
import javax.crypto.IllegalBlockSizeException;
import javax.crypto.KeyGenerator;
import javax.crypto.NoSuchPaddingException;
import javax.crypto.SecretKey;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.SecretKeySpec;
import org.bouncycastle.jce.provider.BouncyCastleProvider;
import com.gitlab.summercattle.commons.exception.CommonException;
import com.gitlab.summercattle.commons.exception.ExceptionWrapUtils;
import com.gitlab.summercattle.commons.security.crypto.constants.CommonEncryptType;
import com.gitlab.summercattle.commons.security.crypto.constants.PaddingType;
/**
* 通用加密工具
* @author orange
*
*/
public class CommonEncryptUtils {
private static final int KEY_SIZE_56 = 56;
private static final int KEY_SIZE_112 = 112;
private static final int KEY_SIZE_128 = 128;
private static final int KEY_SIZE_168 = 168;
private static final int KEY_SIZE_192 = 192;
private static final int KEY_SIZE_256 = 256;
public static byte[] getEncryptKey(CommonEncryptType encryptType, int keySize) throws CommonException {
if (encryptType == null) {
throw new CommonException("加密类型为空");
}
if (keySize <= 0) {
throw new CommonException("密钥长度必须大于零");
}
if (encryptType == CommonEncryptType.AES) {
if (keySize != KEY_SIZE_128 && keySize != KEY_SIZE_192 && keySize != KEY_SIZE_256) {
throw new CommonException(
"密钥长度只能为" + String.valueOf(KEY_SIZE_128) + "位或" + String.valueOf(KEY_SIZE_192) + "位或" + String.valueOf(KEY_SIZE_256) + "位");
}
}
else if (encryptType == CommonEncryptType.DES) {
if (keySize != KEY_SIZE_56) {
throw new CommonException("密钥长度只能为" + String.valueOf(KEY_SIZE_56) + "位");
}
}
else if (encryptType == CommonEncryptType.DESede) {
if (keySize != KEY_SIZE_112 && keySize != KEY_SIZE_168) {
throw new CommonException("密钥长度只能为" + String.valueOf(KEY_SIZE_112) + "位或" + String.valueOf(KEY_SIZE_168) + "位");
}
}
try {
KeyGenerator keyGenerator = KeyGenerator.getInstance(encryptType.toString());
keyGenerator.init(keySize, new SecureRandom());
SecretKey secretKey = keyGenerator.generateKey();
return secretKey.getEncoded();
}
catch (NoSuchAlgorithmException e) {
throw ExceptionWrapUtils.wrap(e);
}
}
public static byte[] encryptCbc(CommonEncryptType encryptType, byte[] data, byte[] key, byte[] iv, PaddingType paddingType)
throws CommonException {
if (encryptType == null) {
throw new CommonException("加密类型为空");
}
if (data == null) {
throw new CommonException("数据为空");
}
if (key == null) {
throw new CommonException("密钥为空");
}
if (iv == null) {
throw new CommonException("IV初始向量为空");
}
if (paddingType == null) {
throw new CommonException("填充类型为空");
}
try {
Cipher cipher = getCipher(encryptType, "CBC", paddingType);
initCbcCipher(cipher, encryptType, key, iv, Cipher.ENCRYPT_MODE);
return cipher.doFinal(data);
}
catch (IllegalBlockSizeException | BadPaddingException e) {
throw ExceptionWrapUtils.wrap(e);
}
}
public static byte[] decyrptCbc(CommonEncryptType encryptType, byte[] encryptData, byte[] key, byte[] iv, PaddingType paddingType)
throws CommonException {
if (encryptType == null) {
throw new CommonException("加密类型为空");
}
if (encryptData == null) {
throw new CommonException("加密后的数据为空");
}
if (key == null) {
throw new CommonException("密钥为空");
}
if (iv == null) {
throw new CommonException("IV初始向量为空");
}
if (paddingType == null) {
throw new CommonException("填充类型为空");
}
try {
Cipher cipher = getCipher(encryptType, "CBC", paddingType);
initCbcCipher(cipher, encryptType, key, iv, Cipher.DECRYPT_MODE);
return cipher.doFinal(encryptData);
}
catch (IllegalBlockSizeException | BadPaddingException e) {
throw ExceptionWrapUtils.wrap(e);
}
}
public static byte[] encryptEcb(CommonEncryptType encryptType, byte[] data, byte[] key, PaddingType paddingType) throws CommonException {
if (encryptType == null) {
throw new CommonException("加密类型为空");
}
if (data == null) {
throw new CommonException("数据为空");
}
if (key == null) {
throw new CommonException("密钥为空");
}
if (paddingType == null) {
throw new CommonException("填充类型为空");
}
try {
Cipher cipher = getCipher(encryptType, "ECB", paddingType);
initEcbCipher(cipher, encryptType, key, Cipher.ENCRYPT_MODE);
return cipher.doFinal(data);
}
catch (IllegalBlockSizeException | BadPaddingException e) {
throw ExceptionWrapUtils.wrap(e);
}
}
public static byte[] decyrptEcb(CommonEncryptType encryptType, byte[] encryptData, byte[] key, PaddingType paddingType) throws CommonException {
if (encryptType == null) {
throw new CommonException("加密类型为空");
}
if (encryptData == null) {
throw new CommonException("加密后的数据为空");
}
if (key == null) {
throw new CommonException("密钥为空");
}
if (paddingType == null) {
throw new CommonException("填充类型为空");
}
try {
Cipher cipher = getCipher(encryptType, "ECB", paddingType);
initEcbCipher(cipher, encryptType, key, Cipher.DECRYPT_MODE);
return cipher.doFinal(encryptData);
}
catch (IllegalBlockSizeException | BadPaddingException e) {
throw ExceptionWrapUtils.wrap(e);
}
}
private static Cipher getCipher(CommonEncryptType encryptType, String encryptMode, PaddingType paddingType) throws CommonException {
try {
String transformation = encryptType.toString() + "/" + encryptMode + "/" + paddingType.toString();
Cipher cipher;
if (encryptType == CommonEncryptType.AES && paddingType == PaddingType.PKCS7Padding) {
cipher = Cipher.getInstance(transformation, getProvider());
}
else {
cipher = Cipher.getInstance(transformation);
}
return cipher;
}
catch (NoSuchAlgorithmException | NoSuchPaddingException e) {
throw ExceptionWrapUtils.wrap(e);
}
}
private static void initEcbCipher(Cipher cipher, CommonEncryptType encryptType, byte[] key, int opmode) throws CommonException {
try {
SecretKeySpec secretKeySpec = new SecretKeySpec(key, encryptType.toString());
cipher.init(opmode, secretKeySpec);
}
catch (InvalidKeyException e) {
throw ExceptionWrapUtils.wrap(e);
}
}
private static void initCbcCipher(Cipher cipher, CommonEncryptType encryptType, byte[] key, byte[] iv, int opmode) throws CommonException {
try {
SecretKeySpec secretKeySpec = new SecretKeySpec(key, encryptType.toString());
AlgorithmParameters algorithmParameters = AlgorithmParameters.getInstance(encryptType.toString());
algorithmParameters.init(new IvParameterSpec(iv));
cipher.init(opmode, secretKeySpec, algorithmParameters);
}
catch (NoSuchAlgorithmException | InvalidParameterSpecException | InvalidKeyException | InvalidAlgorithmParameterException e) {
throw ExceptionWrapUtils.wrap(e);
}
}
private static Provider getProvider() {
return new BouncyCastleProvider();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy