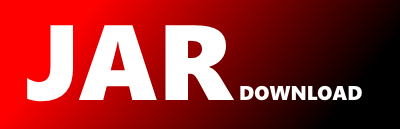
com.gitlab.summercattle.commons.security.crypto.RsaUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cattle-commons-security Show documentation
Show all versions of cattle-commons-security Show documentation
Cattle Framework Commons Security Component
The newest version!
/*
* Copyright (C) 2018 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.gitlab.summercattle.commons.security.crypto;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.security.InvalidKeyException;
import java.security.KeyFactory;
import java.security.KeyPair;
import java.security.KeyPairGenerator;
import java.security.NoSuchAlgorithmException;
import java.security.PrivateKey;
import java.security.PublicKey;
import java.security.SecureRandom;
import java.security.Signature;
import java.security.SignatureException;
import java.security.spec.InvalidKeySpecException;
import java.security.spec.PKCS8EncodedKeySpec;
import java.security.spec.X509EncodedKeySpec;
import java.util.Arrays;
import javax.crypto.BadPaddingException;
import javax.crypto.Cipher;
import javax.crypto.IllegalBlockSizeException;
import javax.crypto.NoSuchPaddingException;
import com.gitlab.summercattle.commons.exception.CommonException;
import com.gitlab.summercattle.commons.exception.ExceptionWrapUtils;
/**
* Rsa工具
* @author orange
*
*/
public class RsaUtils {
private static final int ENCRYPT_SEG = 64;
private static final int DECRYPT_SEG = 128;
private static final int MINIMUM_KEY_SIZE = 512;
private static final String KEY_ALGORITHM = "RSA";
private static final String SIGN_ALGORITHM = "MD5withRSA";
public static KeyPair getEncryptKey(int keySize) throws CommonException {
if (keySize < MINIMUM_KEY_SIZE) {
throw new CommonException("密钥长度必须至少有" + String.valueOf(MINIMUM_KEY_SIZE) + "位长");
}
try {
KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance(KEY_ALGORITHM);
keyPairGenerator.initialize(keySize, new SecureRandom());
return keyPairGenerator.generateKeyPair();
}
catch (NoSuchAlgorithmException e) {
throw ExceptionWrapUtils.wrap(e);
}
}
public static PublicKey getPublicKey(byte[] publicKey) throws CommonException {
if (publicKey == null) {
throw new CommonException("公有密钥为空");
}
try {
X509EncodedKeySpec x509EncodedKeySpec = new X509EncodedKeySpec(publicKey);
KeyFactory keyFactory = KeyFactory.getInstance(KEY_ALGORITHM);
return keyFactory.generatePublic(x509EncodedKeySpec);
}
catch (NoSuchAlgorithmException | InvalidKeySpecException e) {
throw ExceptionWrapUtils.wrap(e);
}
}
public static PrivateKey getPrivateKey(byte[] privateKey) throws CommonException {
if (privateKey == null) {
throw new CommonException("私有密钥为空");
}
try {
PKCS8EncodedKeySpec pkcs8EncodedKeySpec = new PKCS8EncodedKeySpec(privateKey);
KeyFactory keyFactory = KeyFactory.getInstance(KEY_ALGORITHM);
return keyFactory.generatePrivate(pkcs8EncodedKeySpec);
}
catch (InvalidKeySpecException | NoSuchAlgorithmException e) {
throw ExceptionWrapUtils.wrap(e);
}
}
public static byte[] sign(byte[] data, PrivateKey privateKey) throws CommonException {
if (data == null) {
throw new CommonException("需签名的数据为空");
}
if (privateKey == null) {
throw new CommonException("私有密钥为空");
}
try {
Signature signature = Signature.getInstance(SIGN_ALGORITHM);
signature.initSign(privateKey);
signature.update(data);
return signature.sign();
}
catch (NoSuchAlgorithmException | InvalidKeyException | SignatureException e) {
throw ExceptionWrapUtils.wrap(e);
}
}
public static boolean verify(byte[] data, PublicKey publicKey, byte[] sign) throws CommonException {
if (data == null) {
throw new CommonException("数据为空");
}
if (publicKey == null) {
throw new CommonException("公有密钥为空");
}
if (sign == null) {
throw new CommonException("签名为空");
}
try {
Signature signature = Signature.getInstance(SIGN_ALGORITHM);
signature.initVerify(publicKey);
signature.update(data);
return signature.verify(sign);
}
catch (InvalidKeyException | NoSuchAlgorithmException | SignatureException e) {
throw ExceptionWrapUtils.wrap(e);
}
}
public static byte[] encryptByPublicKey(byte[] data, PublicKey publicKey) throws CommonException {
byte[] result = null;
try (ByteArrayOutputStream baos = new ByteArrayOutputStream()) {
int length = data.length;
int pos = 0;
if (length == 0) {
byte[] tmpResult = doEncryptByPublicKey(data, publicKey);
baos.write(tmpResult);
}
else {
while (pos < length) {
byte[] tmp = null;
if (pos + ENCRYPT_SEG < length) {
tmp = Arrays.copyOfRange(data, pos, pos + ENCRYPT_SEG);
}
else {
tmp = Arrays.copyOfRange(data, pos, length);
}
byte[] tmpResult = doEncryptByPublicKey(tmp, publicKey);
baos.write(tmpResult);
pos += ENCRYPT_SEG;
}
}
result = baos.toByteArray();
}
catch (IOException e) {
throw ExceptionWrapUtils.wrap(e);
}
return result;
}
private static byte[] doEncryptByPublicKey(byte[] data, PublicKey publicKey) throws CommonException {
if (data == null) {
throw new CommonException("数据为空");
}
if (publicKey == null) {
throw new CommonException("公有密钥为空");
}
try {
Cipher cipher = Cipher.getInstance(KEY_ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, publicKey);
return cipher.doFinal(data);
}
catch (NoSuchAlgorithmException | InvalidKeyException | NoSuchPaddingException | IllegalBlockSizeException | BadPaddingException e) {
throw ExceptionWrapUtils.wrap(e);
}
}
public static byte[] encryptByPrivateKey(byte[] data, PrivateKey privateKey) throws CommonException {
try (ByteArrayOutputStream baos = new ByteArrayOutputStream()) {
int length = data.length;
int pos = 0;
if (length == 0) {
byte[] tmpResult = doEncryptByPrivateKey(data, privateKey);
baos.write(tmpResult);
}
else {
while (pos < length) {
byte[] tmp = null;
if (pos + ENCRYPT_SEG < length) {
tmp = Arrays.copyOfRange(data, pos, pos + ENCRYPT_SEG);
}
else {
tmp = Arrays.copyOfRange(data, pos, length);
}
byte[] tmpResult = doEncryptByPrivateKey(tmp, privateKey);
baos.write(tmpResult);
pos += ENCRYPT_SEG;
}
}
return baos.toByteArray();
}
catch (IOException e) {
throw ExceptionWrapUtils.wrap(e);
}
}
private static byte[] doEncryptByPrivateKey(byte[] data, PrivateKey privateKey) throws CommonException {
if (data == null) {
throw new CommonException("数据为空");
}
if (privateKey == null) {
throw new CommonException("私有密钥为空");
}
try {
Cipher cipher = Cipher.getInstance(KEY_ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, privateKey);
return cipher.doFinal(data);
}
catch (NoSuchAlgorithmException | InvalidKeyException | NoSuchPaddingException | IllegalBlockSizeException | BadPaddingException e) {
throw ExceptionWrapUtils.wrap(e);
}
}
public static byte[] decryptByPublicKey(byte[] encryptData, PublicKey publicKey) throws CommonException {
try (ByteArrayOutputStream baos = new ByteArrayOutputStream()) {
int length = encryptData.length;
int pos = 0;
while (pos < length) {
byte[] tmp = null;
if (pos + DECRYPT_SEG < length) {
tmp = Arrays.copyOfRange(encryptData, pos, pos + DECRYPT_SEG);
}
else {
tmp = Arrays.copyOfRange(encryptData, pos, length);
}
byte[] tmpResult = doDecryptByPublicKey(tmp, publicKey);
baos.write(tmpResult);
pos += DECRYPT_SEG;
}
return baos.toByteArray();
}
catch (IOException e) {
throw ExceptionWrapUtils.wrap(e);
}
}
private static byte[] doDecryptByPublicKey(byte[] encryptData, PublicKey publicKey) throws CommonException {
if (encryptData == null) {
throw new CommonException("加密后的数据为空");
}
if (publicKey == null) {
throw new CommonException("公有密钥为空");
}
try {
Cipher cipher = Cipher.getInstance(KEY_ALGORITHM);
cipher.init(Cipher.DECRYPT_MODE, publicKey);
return cipher.doFinal(encryptData);
}
catch (NoSuchAlgorithmException | NoSuchPaddingException | IllegalBlockSizeException | BadPaddingException | InvalidKeyException e) {
throw ExceptionWrapUtils.wrap(e);
}
}
public static byte[] decryptByPrivateKey(byte[] encryptData, PrivateKey privateKey) throws CommonException {
try (ByteArrayOutputStream baos = new ByteArrayOutputStream()) {
int length = encryptData.length;
int pos = 0;
while (pos < length) {
byte[] tmp = null;
if (pos + DECRYPT_SEG < length) {
tmp = Arrays.copyOfRange(encryptData, pos, pos + DECRYPT_SEG);
}
else {
tmp = Arrays.copyOfRange(encryptData, pos, length);
}
byte[] tmpResult = doDecryptByPrivateKey(tmp, privateKey);
baos.write(tmpResult);
pos += DECRYPT_SEG;
}
baos.flush();
return baos.toByteArray();
}
catch (IOException e) {
throw ExceptionWrapUtils.wrap(e);
}
}
private static byte[] doDecryptByPrivateKey(byte[] encryptData, PrivateKey privateKey) throws CommonException {
if (encryptData == null) {
throw new CommonException("加密后的数据为空");
}
if (privateKey == null) {
throw new CommonException("私有密钥为空");
}
try {
Cipher cipher = Cipher.getInstance(KEY_ALGORITHM);
cipher.init(Cipher.DECRYPT_MODE, privateKey);
return cipher.doFinal(encryptData);
}
catch (NoSuchAlgorithmException | NoSuchPaddingException | IllegalBlockSizeException | BadPaddingException | InvalidKeyException e) {
throw ExceptionWrapUtils.wrap(e);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy